Mastering FastAPI: The Ultimate Guide to Securing and Retrieving Current User Data
Welcome to the ultimate guide on mastering FastAPI, specifically focusing on securing and retrieving current user data. In the modern era of web development, ensuring the security of user data and efficiently managing sessions is paramount. FastAPI, a high-performance web framework for building APIs with Python 3.7+, offers robust solutions for these challenges. This guide will navigate through the intricacies of implementing authentication, securing endpoints, and retrieving the current user data effectively. Whether you're a beginner or an experienced developer, this comprehensive exploration will equip you with the knowledge to enhance your FastAPI applications.
Understanding Authentication in FastAPI
Authentication is the cornerstone of securing user data. FastAPI provides several tools and mechanisms to implement authentication, from basic authentication to more complex OAuth2 strategies. Understanding how to leverage these tools is the first step in securing your application.
Practical Tip: Start by defining your security scheme using FastAPI's Security
dependencies. It's a straightforward way to integrate authentication into your endpoints.
Example:
from fastapi import Depends, FastAPI, HTTPException, status from fastapi.security import OAuth2PasswordBearer oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token") app = FastAPI() @app.get("/users/me") async def read_users_me(token: str = Depends(oauth2_scheme)): return {"token": token}
Securing Endpoints
After setting up authentication, the next step is securing your endpoints. This involves setting up permissions and ensuring that only authenticated users can access certain parts of your application.
Insight: Utilize FastAPI's dependency injection system to create reusable dependencies that can extract and verify user information from the token.
Example:
from fastapi import Depends, Security from yourapp import get_current_active_user @app.get("/items/") async def read_items(current_user: User = Security(get_current_active_user)): return [{"item_id": "Foo", "owner": current_user.username}]
Retrieving Current User Data
Retrieving current user data is a frequent requirement. FastAPI makes it seamless to access this data securely, ensuring that you can personalize the user experience without compromising on security.
Practical Tip: Create a utility function that decodes the token and fetches the user from the database. Then, use FastAPI's dependency injection to integrate this function into your routes.
Example:
from fastapi import Depends from sqlalchemy.orm import Session from yourapp import get_db, get_current_user @app.get("/users/me") async def read_user_me(current_user: User = Depends(get_current_user)): return current_user
Best Practices for User Data Security
While FastAPI provides the tools, following best practices ensures that your application remains secure. Always use HTTPS for communication, keep your dependencies updated, and regularly review your authentication mechanisms.
Insight: Regularly audit your security practices and update them in accordance with the latest vulnerabilities and patches.
Conclusion
In conclusion, mastering FastAPI for securing and retrieving current user data involves a deep understanding of authentication mechanisms, securing endpoints, and efficiently retrieving user data. By following the guidelines and best practices outlined in this guide, developers can create secure, efficient, and user-friendly web applications. Remember, the security of your application is an ongoing process that requires constant vigilance and updating. Let's strive to build secure and robust applications that respect and protect user data.
Final Thought: Consider contributing to the FastAPI community or sharing your insights by writing tutorials, as sharing knowledge helps in strengthening security practices across the board.
Recent Posts
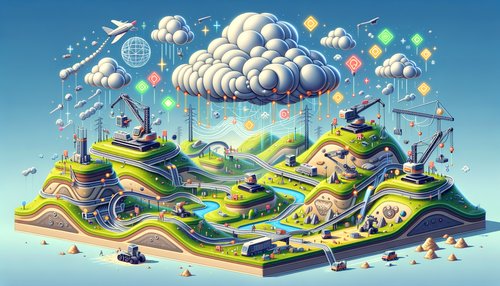
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
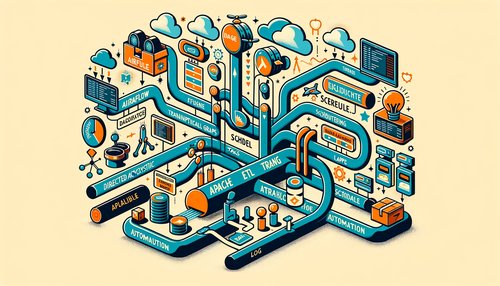
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow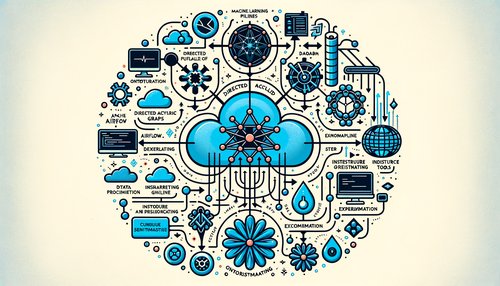
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow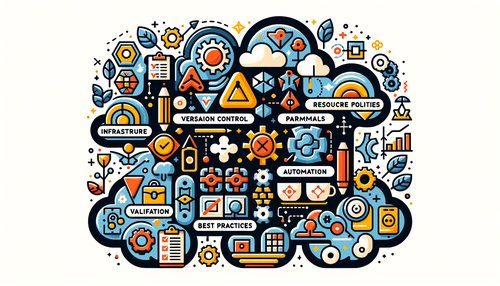
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
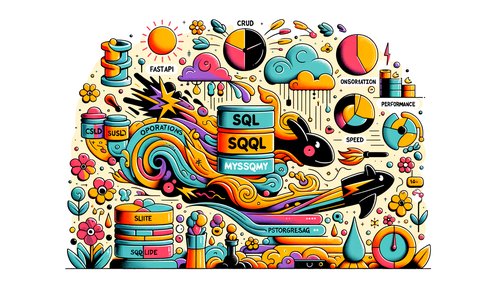