Mastering FastAPI: The Ultimate User Guide to Effective Testing
FastAPI is one of the most powerful and modern web frameworks for building APIs with Python. Its simplicity, performance, and speed are unparalleled, making it an excellent choice for developers looking to create quick and efficient applications. However, as with any web framework, it's crucial to ensure that your application is thoroughly tested. In this blog post, we will explore various strategies and tips for effective testing in FastAPI, ensuring your application is reliable and bug-free.
Why Testing is Crucial in FastAPI
Testing is a vital part of software development, and FastAPI is no exception. Without comprehensive tests, even the most well-structured applications can have hidden bugs and errors that may surface in production, potentially causing significant issues for users. Testing helps to:
- Identify and fix bugs early in the development process
- Ensure code correctness and reliability
- Facilitate code refactoring and optimization
- Provide documentation and examples for new developers on the team
- Increase overall confidence in the codebase
Setting Up Your Testing Environment
Before diving into writing tests, it's essential to set up a proper testing environment. This includes having a dedicated testing database, using appropriate testing tools, and ensuring that your tests are isolated and repeatable.
Required Tools
For FastAPI, you can use several tools for testing:
- Pytest: A powerful testing framework for Python that allows you to write simple and scalable test cases.
- HTTPX: An HTTP client library for Python to make requests to your FastAPI endpoints.
- TestClient: From FastAPI's
starlette.testclient
module, which allows you to test your API with a minimal setup.
To get started, install the required packages:
pip install pytest httpx fastapi
Writing Your First Test Case
Let's start with a simple example. Assume you have a FastAPI application with a basic endpoint:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"message": "Hello World"}
You can write a test case for this endpoint as follows:
import pytest
from fastapi.testclient import TestClient
from main import app # Replace 'main' with the module name where your FastAPI app is defined
client = TestClient(app)
def test_read_root():
response = client.get("/")
assert response.status_code == 200
assert response.json() == {"message": "Hello World"}
Testing Path Parameters and Query Parameters
FastAPI supports both path and query parameters. Here's how you can test endpoints that make use of these parameters:
Path Parameters
Consider this endpoint that takes a path parameter:
@app.get("/items/{item_id}")
def read_item(item_id: int):
return {"item_id": item_id}
And the corresponding test case:
def test_read_item():
item_id = 42
response = client.get(f"/items/{item_id}")
assert response.status_code == 200
assert response.json() == {"item_id": item_id}
Query Parameters
For query parameters, consider this endpoint:
@app.get("/items/")
def read_items(skip: int = 0, limit: int = 10):
return {"skip": skip, "limit": limit}
And the test case:
def test_read_items():
skip = 5
limit = 15
response = client.get(f"/items/?skip={skip}&limit={limit}")
assert response.status_code == 200
assert response.json() == {"skip": skip, "limit": limit}
Handling Database Interactions
Testing endpoints that interact with a database adds another layer of complexity. The key is to ensure that your tests are isolated and do not affect your production data. You can achieve this by using a test database and fixtures.
Here's an example using SQLAlchemy and Pytest fixtures:
import pytest
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
from .database import Base, get_db
from .main import app
from fastapi.testclient import TestClient
SQLALCHEMY_DATABASE_URL = "sqlite:///./test.db"
engine = create_engine(SQLALCHEMY_DATABASE_URL)
TestingSessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base.metadata.create_all(bind=engine)
@pytest.fixture(scope="module")
def db_session():
connection = engine.connect()
transaction = connection.begin()
session = TestingSessionLocal(bind=connection)
yield session
session.close()
transaction.rollback()
connection.close()
@pytest.fixture(scope="module")
def client(db_session):
def override_get_db():
try:
yield db_session
finally:
db_session.close()
app.dependency_overrides[get_db] = override_get_db
return TestClient(app)
You can now write tests that use the client
fixture, ensuring that each test runs in isolation:
def test_create_item(client):
response = client.post("/items/", json={"name": "test item"})
assert response.status_code == 200
assert response.json()["name"] == "test item"
Leveraging Async Tests
FastAPI allows for asynchronous endpoints, which can be tested using pytest-asyncio
:
pip install pytest-asyncio
Here's how to write an async test:
import pytest
import asyncio
from httpx import AsyncClient
from main import app
@pytest.mark.asyncio
async def test_async_endpoint():
async with AsyncClient(app=app, base_url="http://test") as ac:
response = await ac.get("/async-endpoint")
assert response.status_code == 200
assert response.json() == {"message": "Async Hello World"}
Conclusion
Testing is an integral part of developing robust FastAPI applications. By incorporating a structured approach to testing, using the right tools, and following best practices, you can ensure your application remains reliable, maintainable, and bug-free. We hope this guide has provided you with actionable insights and practical tips to master testing in FastAPI.
Happy coding!
Recent Posts
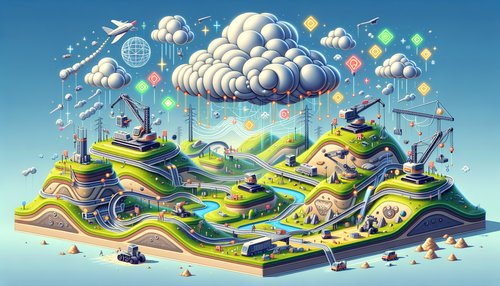
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
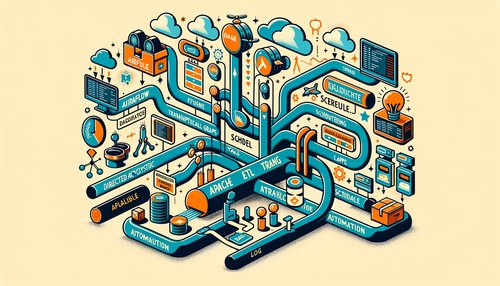
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow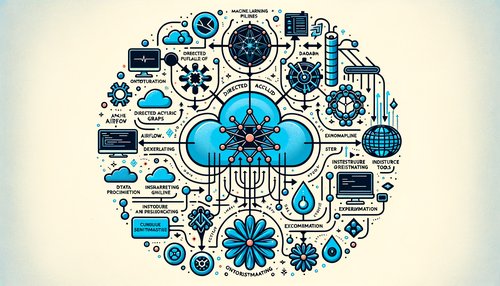
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow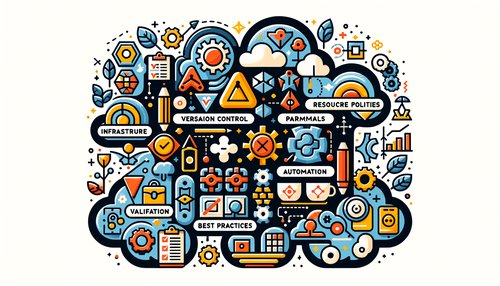
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
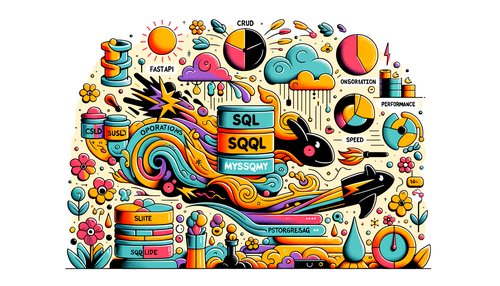