Mastering FastAPI: Unleash the Power of Classes as Dependencies for a Flawless User Guide Experience
Welcome to the world of FastAPI, a modern, fast web framework for building APIs with Python 3.7+ that's based on standard Python type hints. The speed of FastAPI, both in performance and development time, has been a game-changer for many developers. But there's one feature that's particularly powerful and yet underutilized: using classes as dependencies. This blog post will dive deep into how leveraging this feature can significantly enhance your project's structure, scalability, and overall user experience.
The Basics of Dependency Injection in FastAPI
Before we delve into the specifics of using classes as dependencies, let's understand what dependency injection is. In FastAPI, dependency injection is a design pattern for handling dependencies across your application. It allows you to pass dependencies as parameters to your path operation functions. This technique decouples the execution of services from your logic, making your code cleaner, more scalable, and easier to test.
Why Use Classes as Dependencies?
Classes offer a structured way to encapsulate logic and data. By using classes as dependencies in FastAPI, you can take advantage of object-oriented programming to organize your application's components more effectively. This approach allows for:
- Improved Code Reusability: Encapsulate common logic in classes and reuse them across your application.
- Better Organization: Group related functionalities within classes for better modularity and readability.
- Easier Testing: Mock dependencies in your unit tests by replacing class instances with test doubles.
Implementing Classes as Dependencies
To use a class as a dependency in FastAPI, you first define a class that encapsulates your logic. Then, you create an instance of this class or use the class itself as a dependency in your path operation functions. FastAPI's dependency injection system takes care of the rest.
Example: User Authentication
Let's look at a practical example: user authentication. Imagine you have a class UserAuth
that handles authentication logic. You can use an instance of this class as a dependency in your endpoint to authenticate users:
from fastapi import Depends, FastAPI, HTTPException
from pydantic import BaseModel
class UserAuth:
def __init__(self, db_session):
self.db_session = db_session
def authenticate_user(self, username: str, password: str):
# Authentication logic here
return True # Simplified for this example
app = FastAPI()
@app.post("/login")
def login(username: str, password: str, auth: UserAuth = Depends(UserAuth)):
if not auth.authenticate_user(username, password):
raise HTTPException(status_code=400, detail="Incorrect username or password")
return {"message": "User authenticated successfully"}
In this example, UserAuth
is used as a dependency in the login
endpoint. FastAPI takes care of creating an instance of UserAuth
(with any dependencies it might have, such as a database session) and passes it to the function when it's called.
Advanced Use Cases
Using classes as dependencies in FastAPI opens up a myriad of advanced use cases. You can create complex dependency chains, where classes depend on other classes, leading to a well-structured and highly modular application design. Furthermore, you can leverage FastAPI's support for asynchronous functions in your class methods to perform asynchronous operations, such as database queries or HTTP requests, efficiently.
Conclusion
By mastering the use of classes as dependencies in FastAPI, you can significantly enhance the architecture of your applications. This approach promotes code reusability, improves organization, and facilitates testing. Remember, the power of FastAPI coupled with the principles of object-oriented programming can lead to the development of robust, scalable, and maintainable web applications.
As you continue to build and refine your FastAPI applications, consider how you can leverage classes as dependencies to solve common architectural challenges. Experimenting with this powerful feature might just be the key to unlocking the full potential of your projects. Happy coding!
Recent Posts
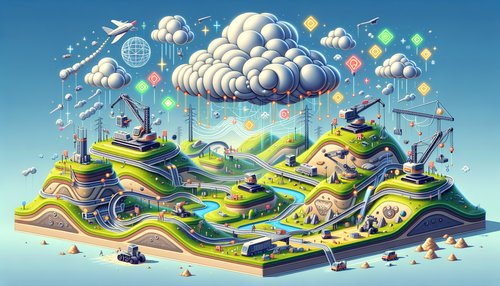
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
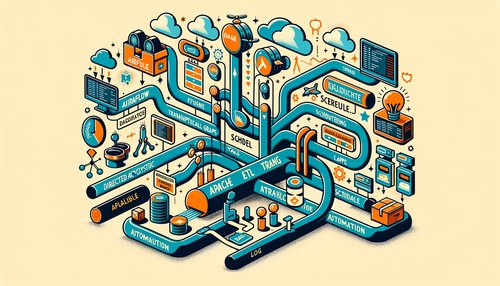
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow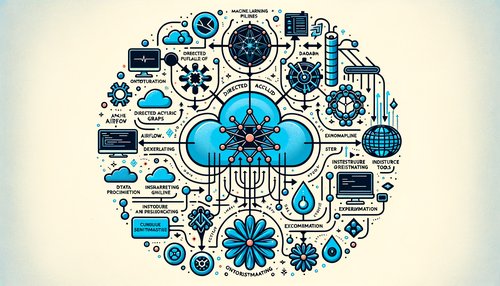
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow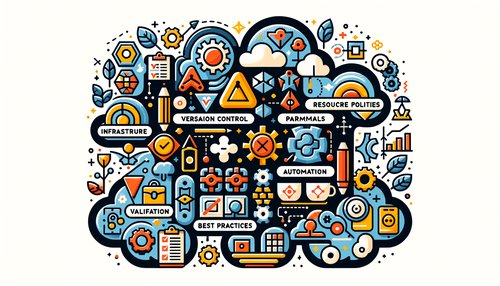
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
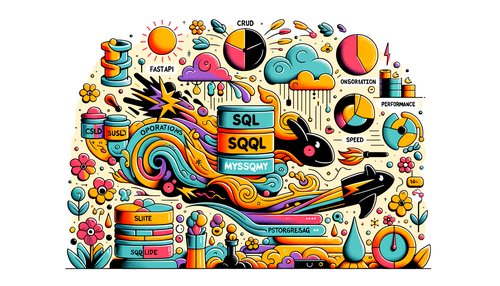