Mastering FastAPI: Your Ultimate Guide to Structuring Bigger Applications Across Multiple Files
Welcome to the world of FastAPI, where speed meets simplicity in the realm of web development. If you've dipped your toes into the waters of FastAPI and are looking to wade deeper, you're in the right place. Building bigger applications requires a robust structure to ensure maintainability, scalability, and simplicity in collaboration. This guide will walk you through the essential steps and strategies to structure your FastAPI applications across multiple files effectively. Get ready to elevate your FastAPI skills and create applications that are not only fast but also well-organized and easy to manage.
Understanding the Basics of FastAPI
Before diving into structuring applications, let's quickly revisit what makes FastAPI stand out. FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. The key features include:
- Automatic Swagger UI documentation
- Data validation and serialization
- Asynchronous request handling
- Dependency injection
These features make FastAPI an excellent choice for building efficient and scalable web applications.
Why Structure Matters in FastAPI Applications
As your application grows, so does the complexity. A monolithic file structure quickly becomes unmanageable, making it difficult to understand, maintain, and extend the application. A well-thought-out structure allows for:
- Separation of concerns
- Easier collaboration among developers
- Improved maintainability and scalability
- More straightforward navigation and organization
Starting with a Project Skeleton
Creating a project skeleton lays the foundation for your application. Begin by organizing your FastAPI application into directories and files. A typical structure might look like this:
project_name/ │ ├── app/ │ ├── __init__.py │ ├── main.py │ ├── dependencies.py │ ├── routers/ │ │ ├── __init__.py │ │ ├── items.py │ │ └── users.py │ └── models/ │ ├── __init__.py │ ├── item.py │ └── user.py ├── tests/ │ └── test_main.py └── requirements.txt
This structure separates your application's components into manageable pieces, focusing on modularity and reusability.
Routing and Endpoints in Separate Files
One of FastAPI's strengths is its easy-to-use router. As your application grows, keeping all your routes in a single file becomes unwieldy. Splitting them into separate files helps keep your code clean and manageable. In the 'routers' directory, you can create files for different categories of routes, such as 'items.py' and 'users.py'. Use FastAPI's include_router method to add these routes to your application:
# In main.py from fastapi import FastAPI from .routers import items, users app = FastAPI() app.include_router(items.router) app.include_router(users.router)
Utilizing Models and Schemas
For data validation and serialization, FastAPI uses Pydantic models. Defining these models in separate files within a 'models' directory helps maintain a clean and organized codebase. This approach also simplifies the reuse of models across your application. Similarly, you might want to define Pydantic schemas for request and response payloads in a 'schemas' directory, mirroring your models' structure.
Managing Dependencies
Dependencies, such as database connections or OAuth2 utilities, can be centralized in a 'dependencies.py' file or broken down further into a 'dependencies' directory for larger applications. FastAPI's dependency injection system allows you to abstract and reuse parts of your logic, such as:
# In dependencies.py from fastapi import Depends, HTTPException def get_token_header(x_token: str = Header(...)): if x_token != "fake-super-secret-token": raise HTTPException(status_code=400, detail="X-Token header invalid")
Conclusion
Structuring your FastAPI applications across multiple files is not just about keeping your codebase clean; it's about ensuring that your application can grow and evolve without becoming a maintenance nightmare. By following the practices outlined in this guide, you'll be well on your way to building scalable, maintainable, and efficient FastAPI applications. Remember, the key to mastering FastAPI lies not only in understanding its features but also in organizing your code effectively. Happy coding!
As you continue to build and structure your FastAPI applications, always look for ways to refine and improve your project's organization. The beauty of FastAPI is that it provides the flexibility to structure your applications in a way that best suits your project's needs. Keep experimenting, keep learning, and most importantly, keep building amazing applications with FastAPI.
Recent Posts
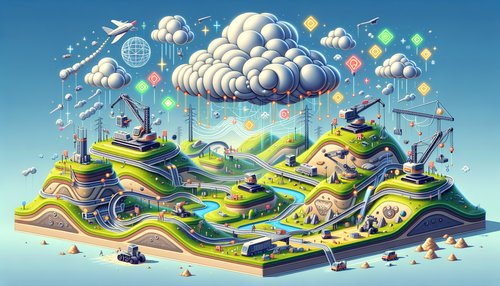
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
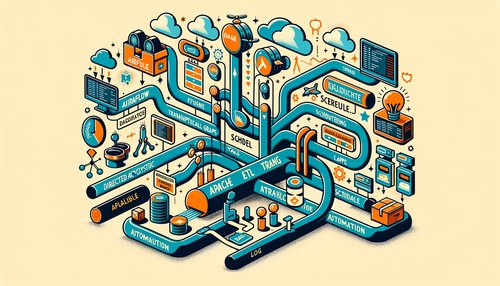
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow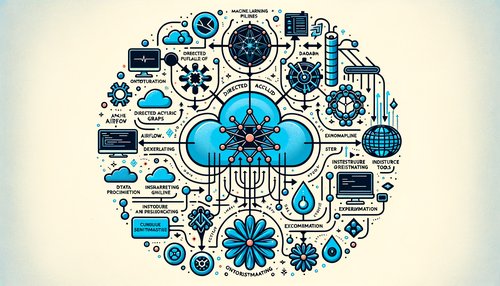
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow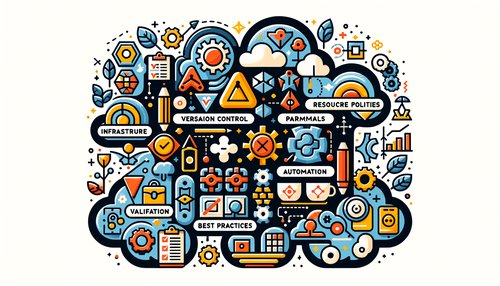
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
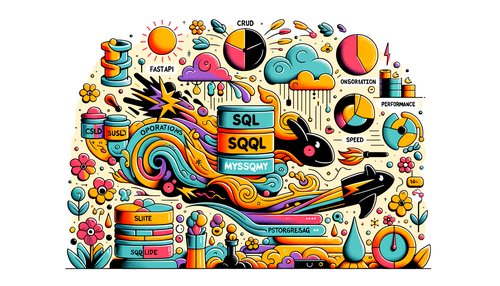