Mastering File Handling in FastAPI: The Ultimate User Guide to Managing Request Files Efficiently
In the modern era of web development, efficiently managing file uploads and downloads becomes crucial for ensuring a seamless user experience. FastAPI, the high-performance web framework for building APIs with Python 3.6+ types, offers robust solutions for handling files. This guide will dive into the nuances of managing request files in FastAPI, providing you with the knowledge to implement file handling seamlessly in your projects.
Understanding File Handling in FastAPI
Before we delve into the specifics, it's important to understand the foundation of file handling in FastAPI. FastAPI uses Form
and File
parameters for uploading files, making it straightforward to integrate file uploads into your API endpoints. This approach not only simplifies the development process but also ensures type safety and validation, thanks to Pydantic models.
Setting Up Your FastAPI Project for File Uploads
To get started with file handling in FastAPI, you first need to set up your project environment. Ensure you have FastAPI and Uvicorn installed. Create a new FastAPI instance and define an endpoint that accepts file uploads using the File
parameter. Here's a simple example:
from fastapi import FastAPI, File, UploadFile
from fastapi.responses import HTMLResponse
app = FastAPI()
@app.post("/upload/")
async def upload_file(file: UploadFile = File(...)):
return {"filename": file.filename}
This code snippet creates an endpoint that accepts a single file upload and returns the file's name. It's a basic example to get you started with handling file uploads.
Handling Multiple File Uploads
FastAPI makes it easy to handle multiple file uploads in a single request. By simply changing the parameter type to a list of UploadFile
instances, you can accept multiple files. Here's how:
from typing import List
@app.post("/upload/multiple/")
async def upload_multiple_files(files: List[UploadFile] = File(...)):
return {"filenames": [file.filename for file in files]}
This enables your endpoint to handle multiple files, enhancing the flexibility of your API.
Working with Uploaded Files
Once you've received the uploaded files, you might want to save them to disk, process them, or perform any number of operations. FastAPI's UploadFile
object provides several useful methods for working with the uploaded files, such as .save()
, which allows you to save the file directly to your server's filesystem.
Advanced File Handling Techniques
For more advanced scenarios, such as streaming large files or handling file downloads, FastAPI provides comprehensive solutions. Streaming files can reduce memory usage on your server, making your application more efficient. Here's an example of how to stream a file upload:
@app.post("/upload/stream/")
async def stream_upload(file: UploadFile = File(...)):
contents = await file.read()
# Process file contents
return {"message": "File processed successfully"}
This approach reads the file content asynchronously, ideal for handling large files or performing real-time processing.
Securing Your File Uploads
Security is paramount when handling file uploads. Always validate the file type and size before processing the uploaded files. FastAPI allows you to set maximum file sizes and use Pydantic models for validation, ensuring that only valid files are processed by your application.
Conclusion
Mastering file handling in FastAPI is essential for developing robust web applications that efficiently manage file uploads and downloads. By following the guidelines and examples provided in this guide, you can implement advanced file handling features in your FastAPI projects, ensuring a secure and seamless user experience. Remember to always validate file uploads and consider streaming for large files to optimize your application's performance.
Now that you're equipped with the knowledge of file handling in FastAPI, it's time to put it into practice. Experiment with different file handling techniques, and don't hesitate to explore FastAPI's documentation for more advanced features and best practices. Happy coding!
Recent Posts
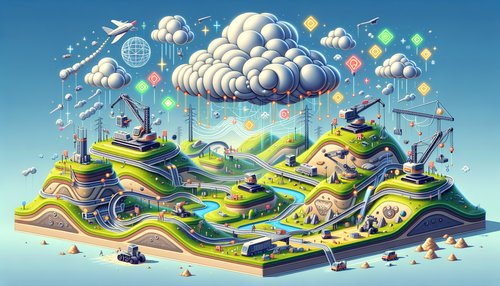
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
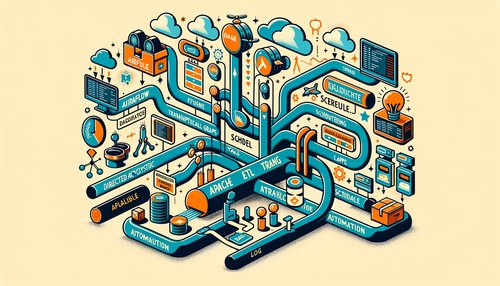
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow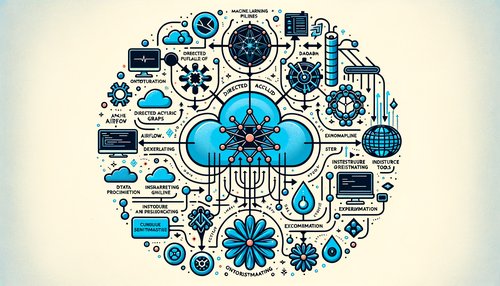
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow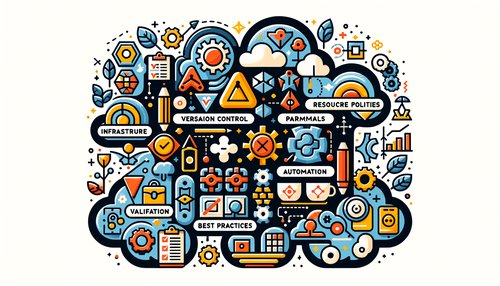
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
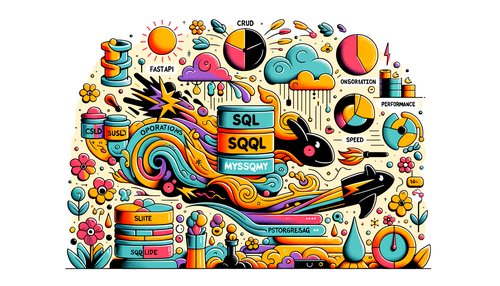