Mastering Modular Magic: Advanced Techniques in Terraform Module Composition
Welcome to the world of advanced Terraform techniques! If you're reading this, you likely already have a basic understanding of Terraform and its capabilities. But have you ever wondered how to elevate your infrastructure as code to new heights? In this post, we'll delve into the intricacies of Terraform module composition, reveal powerful strategies for making your modules more scalable and reusable, and share practical tips to enhance your Terraform projects.
Why Modularize Your Terraform Code?
Modularizing your Terraform code is a game-changer. Not only does it promote reusability and maintainability, but it also allows for easier collaboration within teams. By breaking down your infrastructure code into smaller, self-contained, and reusable modules, you can manage complex setups more efficiently.
Best Practices for Module Composition
Before we dive into advanced techniques, let's quickly recap the best practices for creating Terraform modules:
- Keep modules focused
- Use versioning wisely
- Publish modules to a registry
- Document thoroughly
Advanced Techniques for Effective Module Composition
1. Composition Over Inheritance
When building complex modules, prefer composition over inheritance. Instead of creating one massive "super-module" with countless parameters, break it down into smaller, more manageable components. Each component should serve a single responsibility.
2. Use Meta-Arguments Intelligently
Meta-arguments like count
, for_each
, and depends_on
provide powerful ways to loop over resources, conditionally create resources, and manage dependencies. Using these wisely can help make your modules more flexible and powerful:
resource "aws_instance" "example" {
count = var.create_instance ? 1 : 0
// other arguments here
}
3. Leverage Data Sources
Data sources allow your modules to fetch information from existing infrastructure. This can be particularly useful when you need to dynamically reference resources managed outside your modules:
data "aws_vpc" "selected" {
filter {
name = "tag:Name"
values = [var.vpc_name]
}
}
4. Nesting Modules
Modules can reference other modules. This hierarchical composition can be a neat way to encapsulate complex logic and make your high-level configuration simpler and cleaner.
module "network" {
source = "./modules/network"
}
module "compute" {
source = "./modules/compute"
network_id = module.network.id
}
Practical Tips for Managing Module Complexity
1. Use Local Modules
During development, refer to local paths for modules. This speeds up iteration as changes are immediately reflected:
module "example" {
source = "../modules/example"
}
2. Module Testing
Test each module independently for better stability and faster CI/CD pipelines. Tools like Terratest provide a great framework for this purpose.
3. Contextual Documentation
Keep your module documentation close to your code. Tools like tfdocs can generate module documentation based on the comments and descriptions in your code automatically.
Conclusion
Mastering modular magic in Terraform is no small feat, but it is incredibly rewarding. By applying these advanced techniques in module composition, we can create scalable, maintainable, and reusable infrastructure code. Remember to start small, refactor regularly, and embrace the modular philosophy. Happy terraforming!
Recent Posts
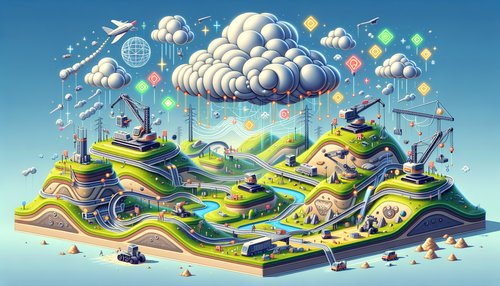
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
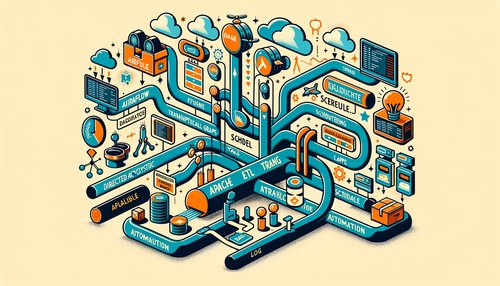
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow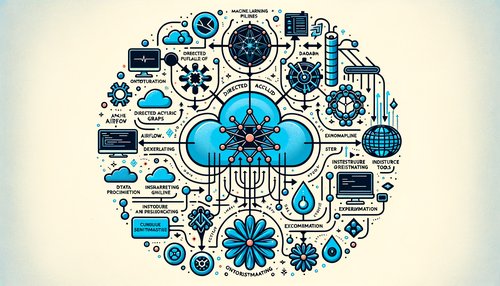
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow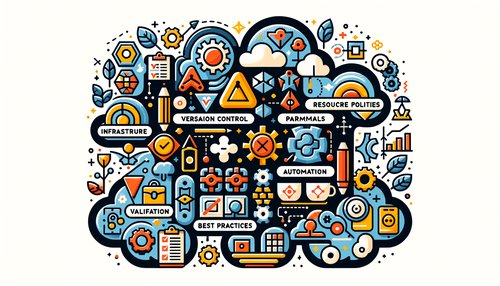
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
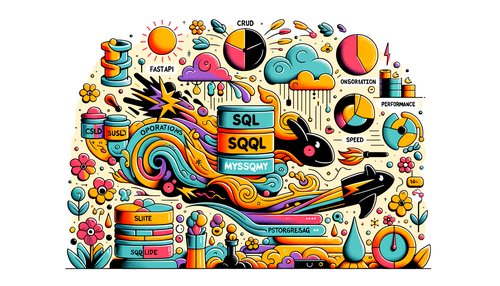