Mastering Pandas in a Flash: Your Ultimate Guide to Navigating Data in Just 10 Minutes!
Welcome to the fast track to becoming a Pandas pro! Whether you're a budding data scientist, a data analyst in the making, or simply curious about handling data efficiently, you're in the right place. In the next 10 minutes, we will embark on a whirlwind tour of Pandas, the powerhouse Python library that has revolutionized data manipulation and analysis. From the basics of series and dataframes to advanced data munging techniques, get ready to unlock the full potential of your data with ease and confidence.
Why Pandas?
Before diving into the how, let's talk about the why. Pandas stands at the heart of data analysis in Python, offering a rich set of functionalities that turn data manipulation from a daunting task into a seamless experience. Its intuitive design and extensive documentation have made it the go-to library for anyone looking to analyze, clean, explore, and visualize data. By mastering Pandas, you're not just learning a library; you're unlocking a gateway to data-driven insights.
Getting Started with Pandas
First things first, let's set up our environment. Installing Pandas is as simple as running pip install pandas
in your terminal. Once installed, you can import Pandas along with NumPy, its numerical mathematics extension, to start manipulating arrays and tables of data. Here's how:
import pandas as pd
import numpy as np
This is your key to the kingdom of data manipulation. With just these two lines of code, you're ready to dive into the world of Pandas.
Understanding Series and DataFrames
At the heart of Pandas are two fundamental data structures: Series and DataFrames. A Series is a one-dimensional array-like object capable of holding any data type, while a DataFrame is a two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns). Grasping these two concepts is crucial for navigating the Pandas library.
Creating Your First DataFrame
Let's jump right in with an example. Imagine you have a dataset of fruit sales. Here's how you can represent this data using a DataFrame:
data = {'Fruits': ['Apples', 'Oranges', 'Bananas'],
'Sales': [100, 150, 200]}
df = pd.DataFrame(data)
print(df)
This simple example illustrates how you can create a DataFrame from a dictionary, with keys as column names and values as data lists. DataFrames can also be created from lists, other DataFrames, or even loaded directly from files, making them incredibly versatile.
Data Manipulation and Analysis
Now that you have your data in a DataFrame, what's next? Pandas offers a plethora of functions for data manipulation and analysis. Here are a few to get you started:
- Indexing and Selection: Use
loc
andiloc
to select rows and columns. - Data Cleaning: Handle missing data with
dropna()
orfillna()
. - Data Aggregation: Summarize data using functions like
groupby()
andaggregate()
. - Data Merging: Combine data from different sources with
merge()
andconcat()
.
Each of these functions opens up new possibilities for data analysis, allowing you to slice, dice, and examine your data from every angle.
Visualization: Bringing Data to Life
What's data analysis without the ability to visualize your findings? Pandas seamlessly integrates with Matplotlib, a powerful plotting library, enabling you to bring your data to life. Here's a simple example:
import matplotlib.pyplot as plt
df.plot(kind='bar', x='Fruits', y='Sales')
plt.show()
This code snippet creates a bar chart of fruit sales, turning your data into an easily digestible visual format. Visualization is key to uncovering patterns, trends, and outliers in your data.
Conclusion: Your Data, Your Way
In just 10 minutes, you've taken a whirlwind tour of Pandas, from installation and basic concepts to data manipulation, analysis, and visualization. While we've only scratched the surface, you now have the foundation to explore the vast capabilities of Pandas and apply them to your own data projects. Remember, the journey to mastering Pandas is a marathon, not a sprint. With practice and persistence, you'll soon be navigating data with ease and uncovering insights that can transform your understanding of the world around you. So, dive in, get your hands dirty with data, and let your data journey begin!
Happy data analyzing!
Recent Posts
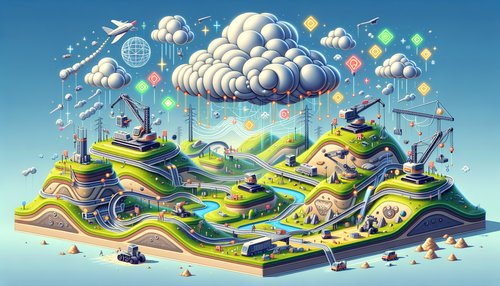
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
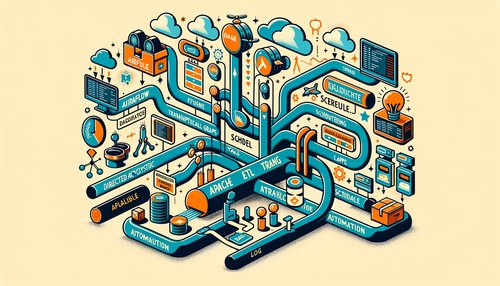
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow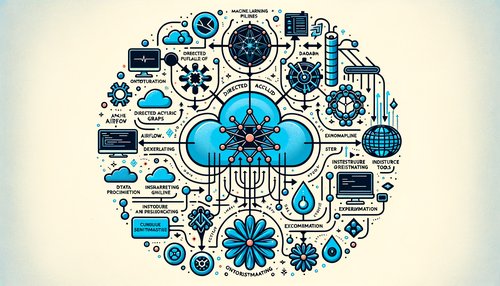
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow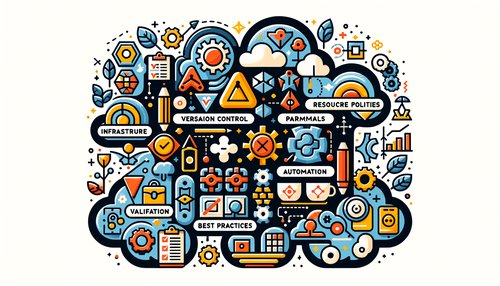
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
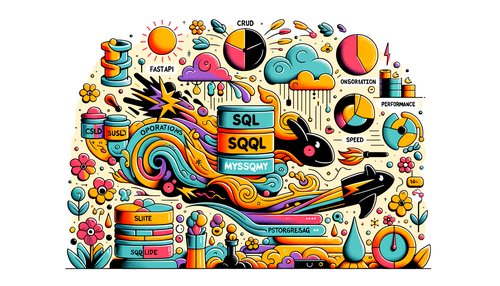