Mastering Path Operation Configuration: Your Ultimate Guide to FastAPI's Power Features
Welcome to your definitive guide on leveraging FastAPI to its fullest potential! Whether you're a seasoned developer or just starting out, understanding the intricacies of path operation configuration in FastAPI is crucial for building efficient and scalable web applications. In this comprehensive blog post, we'll dive deep into the power features of FastAPI, providing you with practical tips, detailed examples, and valuable insights to master this modern, fast (high-performance) web framework for building APIs with Python 3.7+.
Understanding Path Operations in FastAPI
Before we delve into the advanced features, let's establish a solid understanding of what path operations are in FastAPI. A path operation is essentially a Python function that is linked to a specific URL path, responsible for handling requests and returning responses. FastAPI simplifies the process of defining these operations, making your code cleaner and more intuitive.
For instance, creating a basic HTTP GET operation in FastAPI is as straightforward as:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def read_root():
return {"Hello": "World"}
This simplicity, however, belies the framework's power to configure and customize path operations extensively.
Advanced Path Operation Configuration
FastAPI offers a plethora of configuration options to enhance your API's functionality and performance. Let's explore some of these features:
Path Parameters and Validation
FastAPI allows for easy definition of path parameters and automatic request validation. This ensures that the data your API receives is correct and safe, significantly reducing the amount of boilerplate code you need to write for error handling.
from fastapi import FastAPI, Path
app = FastAPI()
@app.get("/items/{item_id}")
async def read_item(item_id: int = Path(..., title="The ID of the item to get")):
return {"item_id": item_id}
In this example, FastAPI automatically validates that item_id
is an integer, and provides descriptive error messages for the client if the validation fails.
Query Parameters and String Validations
Beyond path parameters, FastAPI also simplifies the handling of query parameters, allowing for detailed string validations using Pydantic models. This feature is particularly useful for filtering data or implementing search functionalities in your API.
from fastapi import FastAPI, Query
app = FastAPI()
@app.get("/items/")
async def read_items(q: str = Query(None, min_length=3, max_length=50)):
return {"q": q}
This snippet demonstrates how FastAPI ensures the query parameter q
adheres to the specified length constraints.
Dependency Injection
One of FastAPI's most powerful features is its dependency injection system. This allows you to share logic (such as database connections or security schemes) across multiple path operations, fostering code reusability and maintainability.
from fastapi import Depends, FastAPI
def common_parameters(q: str = None, skip: int = 0, limit: int = 100):
return {"q": q, "skip": skip, "limit": limit}
app = FastAPI()
@app.get("/items/")
async def read_items(commons: dict = Depends(common_parameters)):
return commons
Here, the common_parameters
function is used as a dependency in read_items
, demonstrating how to efficiently reuse common request parameters.
Securing Your API
Security is paramount in web development, and FastAPI provides robust solutions to protect your API. From OAuth2 with Password (and hashing), to JWT tokens and HTTP Basic Auth, FastAPI's security utilities are both flexible and powerful.
Conclusion
We've only scratched the surface of what's possible with FastAPI's path operation configuration. By leveraging path parameters, query string validations, dependency injection, and FastAPI's comprehensive security utilities, you can build highly efficient, secure, and maintainable APIs. The key is to experiment with these features, consult the FastAPI documentation for deeper insights, and continuously refine your approach to API development.
Remember, mastering FastAPI is not just about understanding its features, but also about embracing the mindset of writing clean, efficient, and scalable code. So, take these insights, apply them to your projects, and watch your APIs transform into powerful web services that stand the test of time.
Happy coding!
Recent Posts
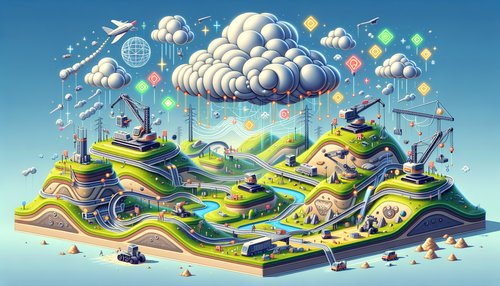
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
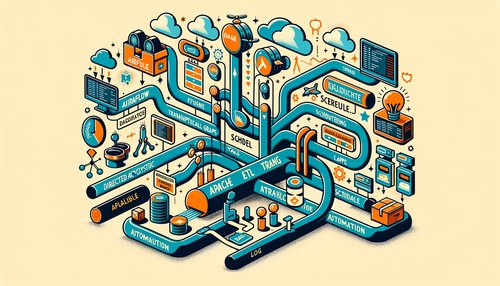
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow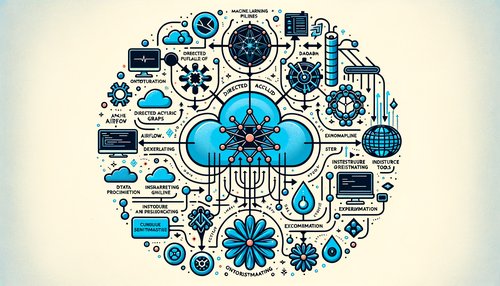
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow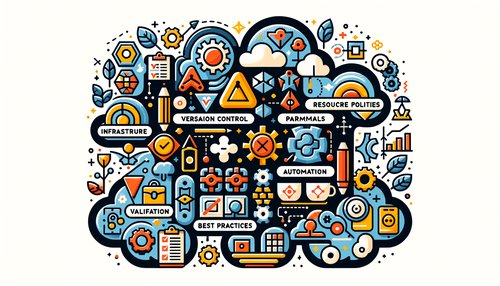
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
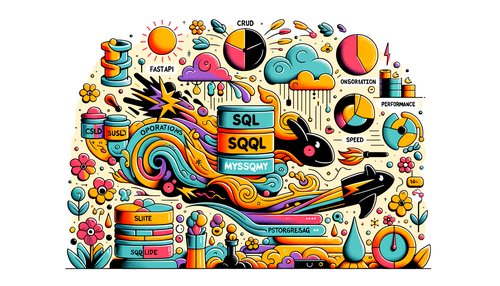