Mastering Python Datetime: Seamlessly Converting AM/PM to German 24-Hour Format
In the world of programming, handling dates and times is a common yet often tricky challenge. One specific task that developers frequently encounter is converting time from the 12-hour AM/PM format to the 24-hour format used in many countries, including Germany. This blog post will guide you through the process of mastering Python's datetime module to achieve this conversion seamlessly. By the end of this article, you'll have a solid understanding of how to work with these formats and the tools to implement the conversion in your projects.
Understanding Python's datetime Module
Before diving into the conversion process, it's essential to understand Python's datetime module. This module supplies classes for manipulating dates and times in both simple and complex ways. datetime allows you to create date objects, extract various components, and perform arithmetic. The key classes in this module are:
datetime.date
- For manipulating dates (year, month, day).datetime.time
- For manipulating time (hours, minutes, seconds, microseconds).datetime.datetime
- Combines date and time information.datetime.timedelta
- For arithmetic with dates and times.
Converting AM/PM to 24-Hour Format
Let's tackle the main task: converting a time from the 12-hour AM/PM format to the 24-hour format. This conversion is straightforward with the help of Python's datetime
and strftime
methods. Here's a step-by-step guide:
Step 1: Import Required Modules
from datetime import datetime
Step 2: Parse the 12-Hour Time String
Assume we have a time string in the 12-hour format:
time_str = '02:30 PM'
We need to parse this string into a datetime
object using strptime
:
time_obj = datetime.strptime(time_str, '%I:%M %p')
The '%I:%M %p'
format code tells strptime
to expect an hour (01-12), minutes, and AM/PM designation.
Step 3: Convert to 24-Hour Format
Once parsed, we can convert the datetime
object to a 24-hour format string using strftime
:
time_24hr_format = time_obj.strftime('%H:%M')
Here, '%H:%M'
specifies a 24-hour time format.
Complete Conversion Code
Bringing it all together, the complete code looks like this:
from datetime import datetime
time_str = '02:30 PM'
time_obj = datetime.strptime(time_str, '%I:%M %p')
time_24hr_format = time_obj.strftime('%H:%M')
print('24-hour format:', time_24hr_format)
Practical Tips for Conversions
When working with time conversions, consider the following practical tips:
- Validate Input: Ensure that the input strings are in the expected format to avoid parsing errors.
- Use Exception Handling: Implement try-except blocks to handle potential errors gracefully.
- Time Zones: Be mindful of time zone differences, especially when converting between formats for international applications.
- Test Extensively: Test the conversions with various time inputs to confirm the accuracy.
Conclusion
Converting time from the AM/PM format to the German 24-hour format is a valuable skill, especially for developers working with international applications. By leveraging Python's robust datetime module, you can perform these conversions seamlessly. Remember to validate inputs, handle exceptions, consider time zones, and test thoroughly. With these tips and techniques, you'll be well-equipped to manage time formats in your future projects. Happy coding!
Ready to take your datetime skills to the next level? Start experimenting with Python's datetime module today and see the difference it can make in your applications.
Recent Posts
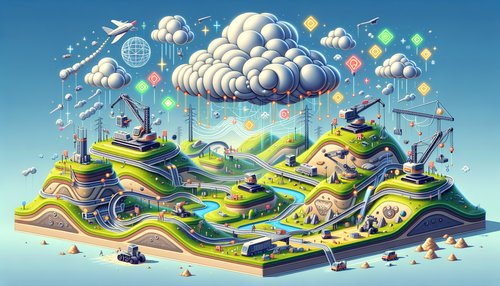
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
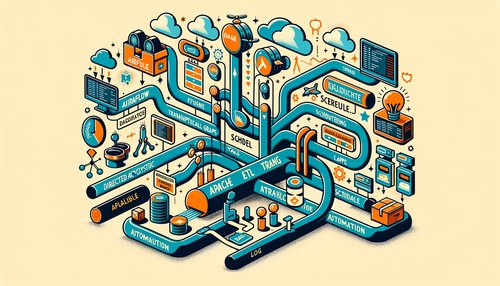
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow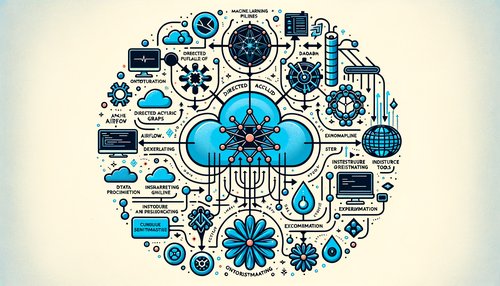
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow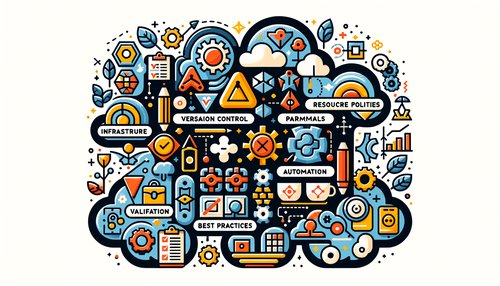
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
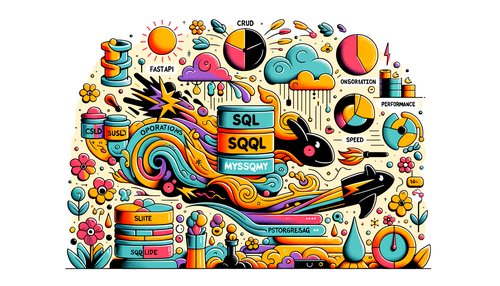