Mastering Terraform: A Guide to Publishing and Managing Reusable Modules
Are you looking to streamline your infrastructure as code (IaC) practices? Terraform modules are the key to reusable, maintainable, and efficient code. This guide will help you master Terraform by exploring how to publish and manage reusable modules effectively. We'll cover everything from the basics to advanced tips and examples, ensuring you come away with practical knowledge to improve your projects.
Introduction to Terraform Modules
Terraform modules are self-contained packages of Terraform configurations that can be reused across different projects. They allow you to encapsulate and share best practices, making your infrastructure more consistent and easier to manage. In this section, we'll discuss what Terraform modules are and why they are essential.
What is a Terraform Module?
At its core, a Terraform module is a collection of configuration files located in the same directory. Even a single .tf file in a directory can be considered a module. Modules can be nested, called, and reused, facilitating a DRY (Don't Repeat Yourself) approach.
Why Use Terraform Modules?
- Reusability: Avoid code duplication by reusing modules across multiple environments or projects.
- Maintainability: Improve the maintainability of your code by isolating its parts into focused, manageable units.
- Consistency: Ensure all environments adhere to best practices and organizational standards.
Creating Your First Terraform Module
Creating a Terraform module is straightforward. Start by organizing your configuration files and defining the inputs and outputs. Here's a step-by-step guide:
Step 1: Organize Your Files
Create a new directory for your module. Place the main configuration file (main.tf
), variables file (variables.tf
), and outputs file (outputs.tf
) in this directory.
module/
├── main.tf
├── variables.tf
├── outputs.tf
Step 2: Define Inputs
Use the variables.tf
file to define the inputs for your module. This makes your module flexible and customizable.
variable "region" {
description = "The AWS region to deploy resources"
type = string
}
Step 3: Define Resources in main.tf
In main.tf
, define the resources your module will create. Reference input variables to make your configuration dynamic.
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
region = var.region
}
Step 4: Set Outputs
Use the outputs.tf
file to define what your module will output, making it easier for other configurations to use its results.
output "instance_id" {
description = "The ID of the AWS instance"
value = aws_instance.example.id
}
Publishing Terraform Modules
Once you've created a module, you can publish it for others to use. This is often done via the Terraform Registry or a Version Control System (VCS) like GitHub.
Using Terraform Registry
The Terraform Registry is a public repository of modules that anyone can access. To publish a module:
- Ensure your module follows the Terraform Registry requirements.
- Version your module using Git tags (e.g.,
v1.0.0
). - Push your repository to GitHub, GitLab, or Bitbucket.
- Follow the instructions on the Terraform Registry to publish your module.
Version Control Systems
Alternately, you can share modules using a VCS by providing the repository URL when you call the module.
module "example" {
source = "github.com/yourusername/repo//path/to/module"
version = "v1.0.0"
}
Managing Module Versions
Managing module versions is crucial for maintaining stability and compatibility. Here are some best practices:
Semantic Versioning
Use semantic versioning (semver) to version your modules, indicating backward-compatible changes, and breaking changes.
module "example" {
source = "github.com/yourusername/repo//path/to/module"
version = "~> 1.0"
}
The ~> 1.0
version constraint ensures only minor and patch updates are allowed.
Locking Versions
Always lock module versions in your Terraform configurations to prevent unexpected changes.
module "example" {
source = "github.com/yourusername/repo//path/to/module"
version = "= 1.0.0"
}
Best Practices for Managing Reusable Modules
To get the most out of your reusable modules, follow these best practices:
Keep It Simple
Avoid overcomplicating modules. Focus on single responsibilities, and avoid heavy logic within modules.
Documentation
Document your modules thoroughly. Include input variables, outputs, and usage examples to make it easy for others to use them.
Testing
Test your modules extensively. Use tools like Terratest to automate testing and ensure reliability.
Conclusion
Mastering Terraform modules can significantly enhance your infrastructure management capabilities. By creating, publishing, and managing reusable modules effectively, you'll streamline your IaC workflows, improve consistency, and make your code more maintainable. Start building and sharing your Terraform modules today, and watch your productivity soar!
Want to dive deeper? Explore the official Terraform module documentation for more advanced features and best practices.
Recent Posts
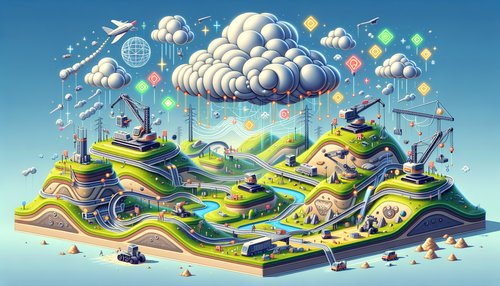
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
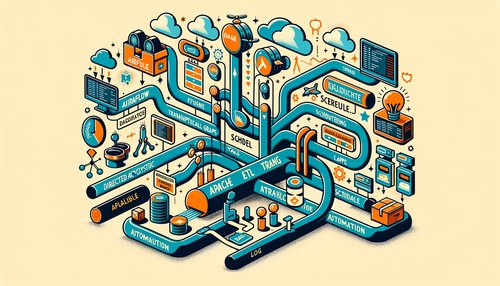
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow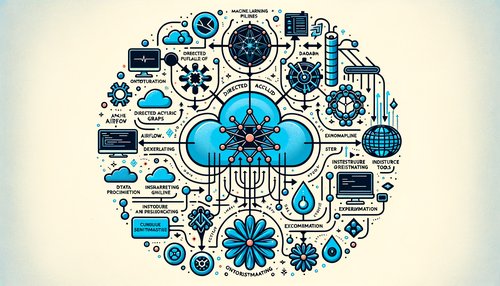
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow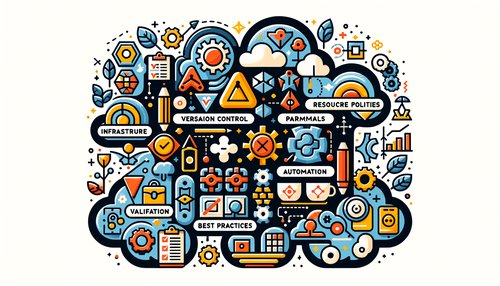
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
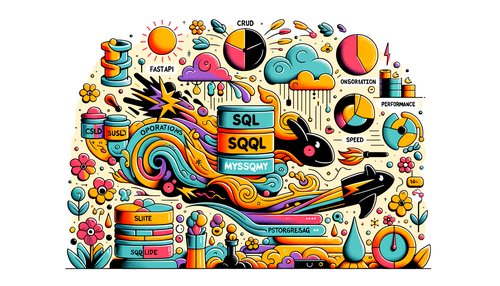