Mastering Terraform: Unleashing the Power of Arithmetic and Logical Operators in Expressions
Welcome to your guide on harnessing the full potential of Terraform, particularly focusing on the power of arithmetic and logical operators within its expressions. Whether you're a DevOps engineer, a hobbyist, or someone in between, understanding how to manipulate these operators effectively can enhance your Terraform configurations significantly. This blog post will delve into the fundamentals, practical applications, and advanced tips for optimizing your configurations.
Understanding Terraform Expressions
Terraform expressions are the basis of dynamic configurations in your infrastructure. They allow you to perform calculations, manipulate data, and define conditions. At the core of these expressions are operators, which can be broadly categorized into arithmetic, logical, comparison, and others like concatenation.
Arithmetic Operators
Arithmetic operators in Terraform perform basic math operations, which are essential when you need to manipulate numbers directly within your configuration. Here's a quick overview:
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus
For example, if you're defining a resource count based on desired redundancy, you can perform inline calculations:
resource "aws_instance" "example" {
count = var.desired_copies + 1
# other arguments
}
This simple expression ensures that you always deploy at least one extra instance beyond what is specified.
Logical Operators
Logical operators help you evaluate conditions, enabling you to make decisions directly in your configuration files. Key logical operators include:
==
and!=
: Equal to and not equal to>
,<
,>=
,<=
: Greater than, less than, greater than or equal to, and less than or equal to&&
: Logical AND||
: Logical OR!
: Logical NOT
Consider a scenario where you need to validate input variables, ensuring they meet your conditions before provisioning resources:
variable "instance_type" {
type = string
default = "t2.micro"
}
locals {
valid_instance = var.instance_type == "t2.micro" || var.instance_type == "t2.small"
}
resource "aws_instance" "example" {
count = local.valid_instance ? 1 : 0
# other arguments
}
The logical OR operator checks if the instance type is valid for provisioning, and the ternary operator (condition ? true_val : false_val
) determines the count of resources to deploy.
Combining Arithmetic and Logical Operators
Combining arithmetic and logical operators allows more complex decision-making capabilities. For instance, suppose you want to scale your infrastructure based on usage statistics; you could use both operator types to set thresholds and auto-adjust resources.
variable "current_cpu_load" {
type = number
}
locals {
should_scale = var.current_cpu_load > 75.0
}
resource "aws_autoscaling_group" "example" {
desired_capacity = local.should_scale ? var.current_capacity + 1 : var.current_capacity
# other settings
}
This configuration dynamically adjusts your autoscaling group's desired capacity based on the CPU load.
Practical Tips and Insights
- Debugging Expressions: Use the Terraform console to test and debug expressions. It helps in validating logic before deployment.
- Keep It Readable: While chaining operators can be powerful, make sure expressions remain understandable, using local variables or modules where necessary.
- Performance Considerations: Avoid overly complex logic in expressions that can slow down configuration updates.
Conclusion
Mastering arithmetic and logical operators in Terraform not only optimizes your infrastructure management but also empowers you to create more adaptive and intelligent configurations. As you continue to explore Terraform's capabilities, consider experimenting with complex use cases and share those learnings with the broader community. Happy provisioning!
Recent Posts
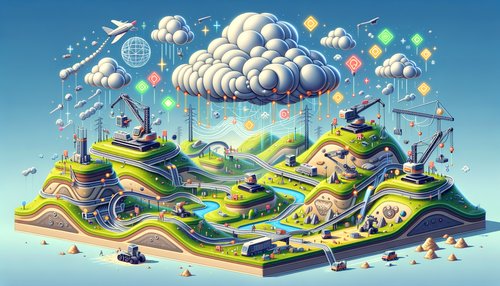
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
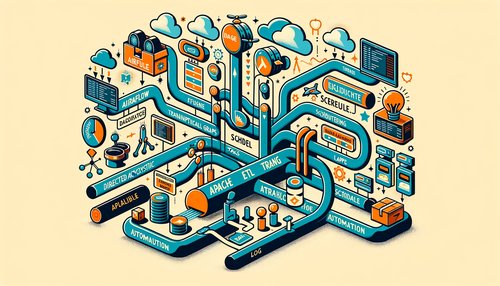
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow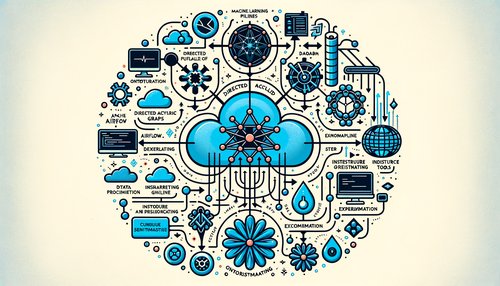
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow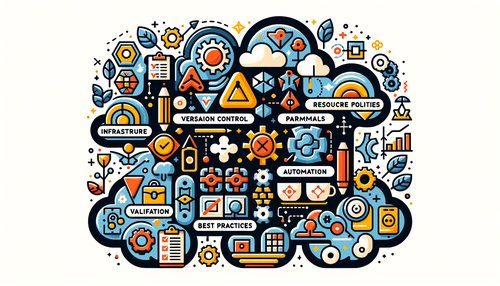
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
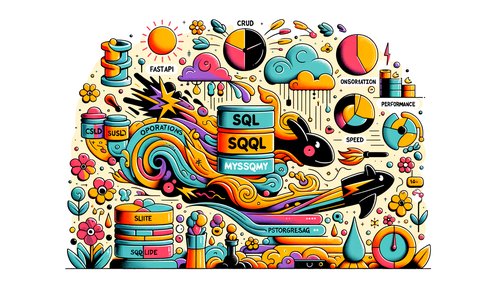