Mastering Terraform: Unlock the Power of Providers Within Modules
Welcome to our comprehensive guide on mastering Terraform, particularly focusing on the power of providers within modules. If you've ever struggled with Terraform's provider configurations or wondered how to better structure your modules to utilize providers effectively, you're in the right place. This post will cover the fundamentals of providers, best practices for managing them within modules, and practical examples to illustrate their powerful capabilities.
Understanding Providers in Terraform
Providers are a critical component of Terraform. They act as the bridge between Terraform and the APIs of the services you want to use. Whether you're provisioning resources on AWS, setting up a firewall rule in GCP, or creating a virtual machine on Azure, providers make these interactions possible.
The Basics
At its core, a provider in Terraform is configured with credentials and other necessary settings to interact with the target API:
provider "aws" {
region = "us-west-2"
access_key = "your-access-key"
secret_key = "your-secret-key"
}
This snippet sets up an AWS provider with specific credentials and region settings.
Using Providers Within Modules
Modules are reusable, configurable, and shareable Terraform configurations. However, managing providers within modules can be tricky. Proper handling of providers enables more flexible and maintainable code.
Dynamic Provider Configuration
One way to handle providers within modules is by allowing the root module to pass provider configurations into child modules:
module "network" {
source = "./modules/network"
providers = {
aws = aws
}
}
This ensures that the 'network' module uses the same AWS provider configuration as defined in the root module.
Alias and Multiple Provider Instances
Sometimes you'll need multiple configurations of the same provider, for example, to work with multiple AWS regions. Terraform's provider alias functionality makes this possible:
provider "aws" {
alias = "us_east"
region = "us-east-1"
}
provider "aws" {
alias = "us_west"
region = "us-west-2"
}
module "network" {
source = "./modules/network"
providers = {
aws.us_east = aws.us_east
aws.us_west = aws.us_west
}
}
In this example, the 'network' module is configured to use two distinct AWS provider instances, allowing the creation of resources in two different regions.
Practical Tips for Mastering Providers in Modules
Tip #1: Explicitly Pass Providers
Always pass providers explicitly to ensure that modules use the correct configurations, especially when dealing with multiple providers or aliases.
Tip #2: Centralize Provider Configuration
Define provider configurations in a central place (such as the root module) and propagate them to child modules. This approach maintains consistency and simplifies management.
Tip #3: Use Versions and Constraints
Specify provider versions and constraints to prevent unexpected changes from breaking your Terraform code. For example:
provider "aws" {
version = "~> 3.0"
}
Examples of Advanced Provider Usage
Let's look at some more advanced examples to deepen your understanding:
Multi-Provider Module
variable "region" {
description = "Region to deploy resources"
}
provider "aws" {
alias = "main"
region = var.region
}
provider "aws" {
alias = "backup"
region = "us-east-1"
}
module "main_resources" {
source = "./modules/main_resources"
providers = {
aws = aws.main
}
}
module "backup_resources" {
source = "./modules/backup_resources"
providers = {
aws = aws.backup
}
}
In this scenario, you declare a region
variable and use it to configure the main provider instance, while also setting up a secondary provider for backup resources.
Inherited Providers
Modules can inherit providers from the root module, making it simpler to use a unified configuration:
module "compute" {
source = "./modules/compute"
region = var.region
}
Ensure your child module does not hard-code provider configurations but uses inherited values.
Conclusion
Mastering providers within Terraform modules is crucial for creating scalable, maintainable, and efficient cloud infrastructure. By understanding the basics, utilizing dynamic provider configurations, employing multiple aliases, and following best practices, you can unlock the full power of Terraform providers. Now it’s your turn to put these insights into action. Start refining your modules today and experience the difference in your Terraform workflows!
Thank you for reading. Don't forget to share this post with others who might find it helpful and leave your comments or questions below!
Recent Posts
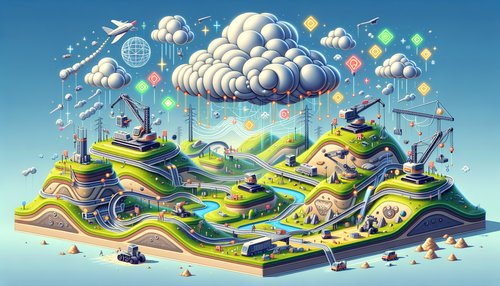
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
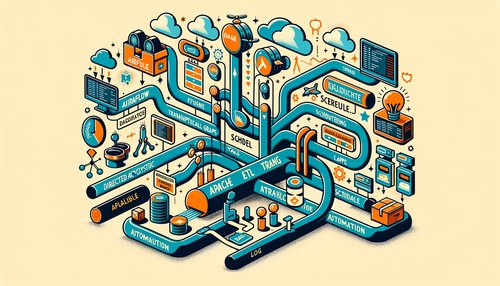
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow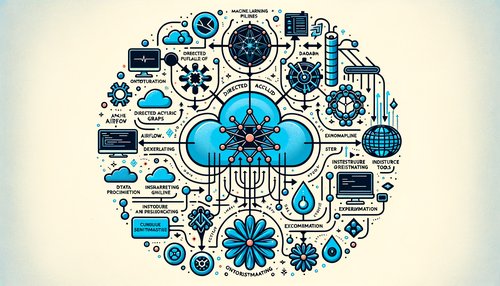
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow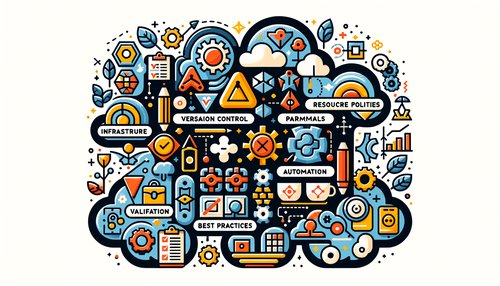
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
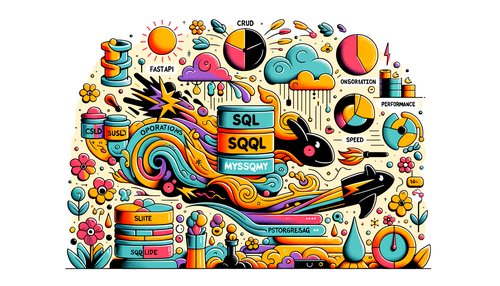