Mastering the Art of Automation: Unlocking the Full Potential of GitLab CI/CD for Streamlined DevOps Workflows
Automation is the beating heart of any efficient DevOps practice, and GitLab CI/CD is a powerful tool that helps developers automate the software development process, from code integration to delivery and deployment. In this post, we'll explore how to master GitLab CI/CD to streamline your DevOps workflows, with practical code snippets to get you started.
Understanding GitLab CI/CD
Continuous Integration (CI) and Continuous Delivery/Deployment (CD) are practices that enable developers to merge code changes more frequently and deliver quality software quickly. GitLab CI/CD is an integrated feature within GitLab that provides a framework to automate these practices within your development lifecycle.
Setting Up Your .gitlab-ci.yml File
The .gitlab-ci.yml
file is where you define your CI/CD pipeline. This YAML file specifies the stages of your pipeline and the jobs that will be executed.
stages:
- build
- test
- deploy
build_job:
stage: build
script:
- echo "Building the project..."
- make build
test_job:
stage: test
script:
- echo "Running tests..."
- make test
deploy_job:
stage: deploy
script:
- echo "Deploying to production..."
- make deploy
Leveraging Docker for Consistent Environments
Using Docker with GitLab CI/CD can ensure that your builds are run in a consistent environment. You can specify a Docker image in your .gitlab-ci.yml
file to use as the base for your jobs.
image: python:3.9
test_job:
stage: test
script:
- pip install -r requirements.txt
- python -m unittest discover
Optimizing with Caching and Artifacts
Caching dependencies and saving artifacts are essential for optimizing your pipeline. Caching can reduce the time spent on downloading and installing dependencies, while artifacts can be used to pass data between stages or jobs.
build_job:
stage: build
script:
- npm install
- npm run build
cache:
paths:
- node_modules/
test_job:
stage: test
script:
- npm test
artifacts:
paths:
- test_report.xml
expire_in: 1 week
Automating Deployment with Environments
GitLab CI/CD allows you to automate your deployment process. You can define different environments, such as staging and production, and deploy your application accordingly.
deploy_staging:
stage: deploy
script:
- echo "Deploying to staging..."
- deploy_to_staging.sh
environment:
name: staging
url: https://staging.example.com
deploy_production:
stage: deploy
script:
- echo "Deploying to production..."
- deploy_to_production.sh
environment:
name: production
url: https://example.com
only:
- master
Securing Your Pipeline
Security is crucial in CI/CD pipelines. GitLab provides features such as Secret Variables to store sensitive information, and Review Apps to preview changes securely.
deploy_review:
stage: deploy
script:
- echo "Deploying review app..."
- deploy_review_app.sh
environment:
name: review/$CI_COMMIT_REF_NAME
on_stop: stop_review
only:
- branches
except:
- master
stop_review:
stage: deploy
variables:
GIT_STRATEGY: none
script:
- echo "Stopping review app..."
- stop_review_app.sh
when: manual
environment:
name: review/$CI_COMMIT_REF_NAME
action: stop
Conclusion
Mastering GitLab CI/CD is about understanding how to configure your pipeline to match your workflow. By utilizing Docker, caching, artifacts, and environments, you can create a pipeline that is efficient, consistent, and secure. Remember to keep your .gitlab-ci.yml
file clean and readable, and always test your pipeline configurations before implementing them. With these practices, you'll be well on your way to unlocking the full potential of automation with GitLab CI/CD.
Recent Posts
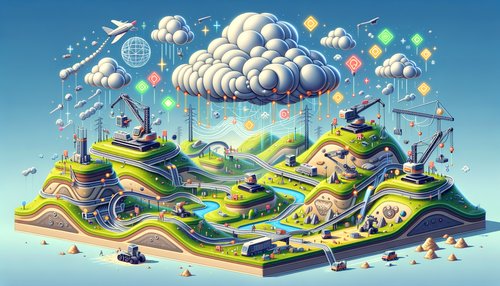
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
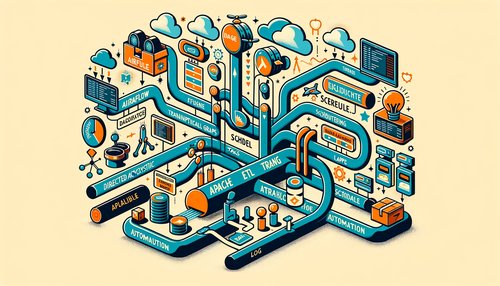
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow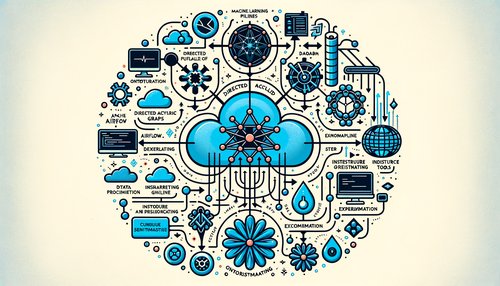
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow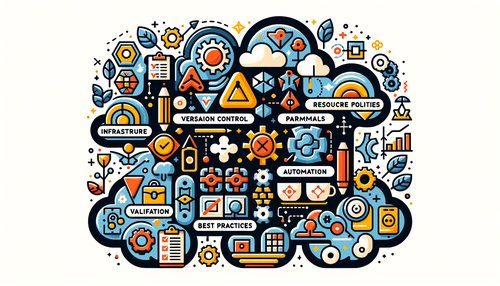
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
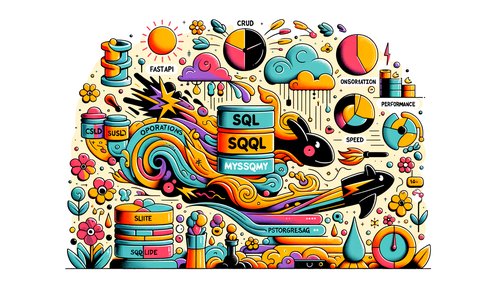