Mastering the Bamboo Forest: Essential Basic Functionality for Navigating the Pandas User Guide
Welcome to the dense and intricate bamboo forest of data manipulation: the Pandas library in Python. Just as a bamboo forest is full of pathways and hidden gems, the Pandas library offers a rich environment for data analysis and manipulation. This blog post is your compass, guiding you through the essential basic functionalities of Pandas, as outlined in its comprehensive user guide. Whether you're a novice data analyst or a seasoned data scientist looking to brush up on your skills, this post will provide you with the knowledge and tools to navigate the Pandas landscape with ease.
Getting Started with Pandas
Before diving into the functionalities of Pandas, it's essential to understand what Pandas is and why it's a critical tool for data analysis. Pandas is an open-source data analysis and manipulation tool built on top of the Python programming language. It offers data structures and operations for manipulating numerical tables and time series, making data cleaning, analysis, and visualization easier and more intuitive.
To get started with Pandas, you'll first need to install it using pip:
pip install pandas
Once installed, you can import Pandas in your Python script alongside NumPy, a library that adds support for large, multi-dimensional arrays and matrices, which Pandas uses under the hood:
import pandas as pd
import numpy as np
Understanding Pandas Data Structures
At the heart of Pandas are two primary data structures: Series and DataFrames. A Series is a one-dimensional array-like structure designed to hold any data type, while a DataFrame is a two-dimensional, table-like structure designed to hold multiple series of different data types. Understanding these structures is crucial for effective data manipulation.
Series
A Series can be created from a list, array, or dictionary. Here’s a simple example:
data = pd.Series([1, 3, 5, np.nan, 6, 8])
This creates a Series with an index automatically assigned.
DataFrames
DataFrames can be thought of as dictionaries of Series. They can be created in multiple ways, but one common method is from a dictionary:
df = pd.DataFrame({
'A': 1.,
'B': pd.Timestamp('20130102'),
'C': pd.Series(1, index=list(range(4)), dtype='float32'),
'D': np.array([3] * 4, dtype='int32'),
'E': pd.Categorical(["test", "train", "test", "train"]),
'F': 'foo'
})
This creates a DataFrame with various data types, showcasing the flexibility of Pandas DataFrames.
Basic Functionality
With a basic understanding of Series and DataFrames, let's explore some fundamental functionalities of Pandas that are essential for data analysis.
Viewing Data
To quickly inspect your data, you can use:
df.head() # View the first 5 rows
df.tail(3) # View the last 3 rows
Indexing and Selecting Data
Pandas provides multiple methods for indexing and selecting data, such as:
df['A']
- Selects a single column, which yields a Series, equivalent todf.A
.df[0:3]
- Selects rows using slicing.df.loc[:, ['A', 'B']]
- Selects on a multi-axis by label.
Data Cleaning
Data cleaning is a critical step in data analysis. Pandas offers several functionalities for handling missing data, dropping entries, filling gaps, and more. For example, to drop any rows that contain missing data:
df.dropna(how='any')
To fill missing data:
df.fillna(value=5)
Summary
In this blog post, we journeyed through the bamboo forest of Pandas, exploring its essential functionalities as outlined in the Pandas user guide. We started with an introduction to Pandas and its core data structures, Series and DataFrames, and then delved into basic functionalities like viewing data, indexing, selecting, and cleaning data.
Mastering these basic functionalities is like finding your path through a dense forest. It equips you with the knowledge and tools to perform efficient data analysis and manipulation, paving the way for more advanced data science tasks. Keep exploring, practicing, and remember, the Pandas user guide is your map in this vast bamboo forest of data analysis.
As you continue your journey, don't hesitate to refer back to the Pandas user guide for deeper exploration of its functionalities. Happy data analyzing!
Recent Posts
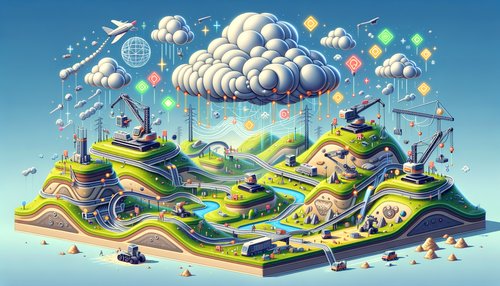
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
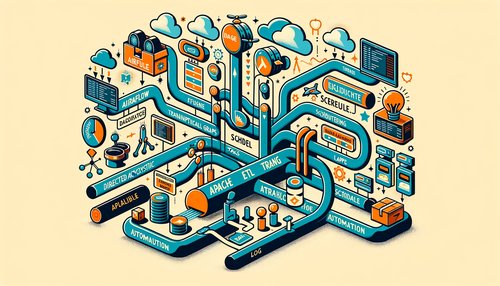
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow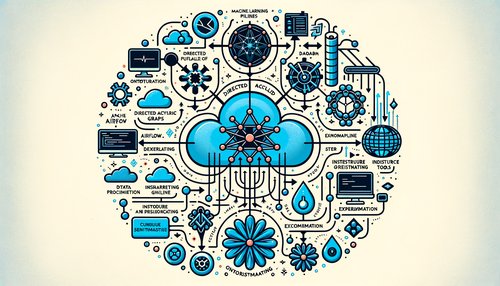
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow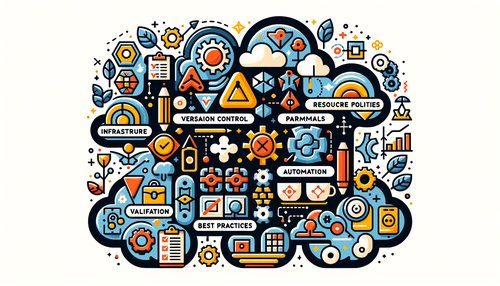
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
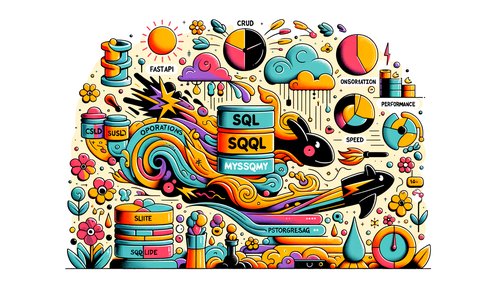