Mastering the Maze: Navigating Duplicate Labels in the Pandas User Guide
Have you ever found yourself lost in a labyrinth of labels within your data, unable to find a clear path through? Duplicate labels in Pandas can create such a maze, confusing your analysis and leading to erroneous results. This comprehensive guide will serve as your map, helping you navigate and master the complexities of duplicate labels in the Pandas library. From understanding the issue to implementing practical solutions, we'll cover everything you need to ensure your data analysis is both accurate and efficient.
Understanding the Challenge
Duplicate labels in a DataFrame or Series can occur more often than one might expect. Whether it's due to data entry errors, merging datasets without proper cleaning, or simply overlooking the uniqueness of identifiers, these duplicates can wreak havoc on your data analysis processes. Understanding the nature of these duplicates and their potential impact is the first step towards mastering them.
Identifying Duplicate Labels
Before you can tackle the problem, you need to know how to identify duplicate labels. Pandas offers several tools to detect duplicates, such as the duplicated()
method for Series and DataFrame objects. This method returns a boolean Series, marking duplicates as True
except for the first occurrence. However, when dealing with index labels specifically, a more manual approach using the index.duplicated()
method might be necessary.
import pandas as pd
# Example DataFrame with duplicate labels
df = pd.DataFrame({'Data': [10, 20, 30, 40]}, index=['a', 'b', 'a', 'c'])
# Identifying duplicate index labels
print(df.index.duplicated())
Strategies for Handling Duplicate Labels
Once you've identified duplicate labels in your dataset, the next step is to decide on a strategy for handling them. There are several approaches, each with its own advantages and disadvantages:
- Removing Duplicates: In cases where duplicate labels represent truly redundant data, simply removing them might be the best approach. The
drop_duplicates()
method can be used for this purpose, though care should be taken to ensure that no critical information is lost. - Aggregating Data: When duplicates represent valid but repeated measurements, aggregating this data (e.g., taking the mean, sum, or another statistical operation) can provide a way to consolidate the information meaningfully.
- Rename Labels: Renaming one or all of the duplicate labels to ensure uniqueness is another strategy. This can be particularly useful in datasets where each entry should be distinct but was improperly labeled.
Practical Tips and Insights
Dealing with duplicate labels requires a keen eye and a careful hand. Here are some practical tips to help you navigate this challenge:
- Always verify the uniqueness of your index: After cleaning or manipulating your dataset, verify that your index or columns are unique to prevent unforeseen issues in analysis.
- Use aggregation wisely: When choosing to aggregate duplicate data, consider the implications of the method you choose. The mean might be appropriate for some datasets, while the sum or median might be better for others.
- Consistency is key: Ensure that your approach to handling duplicates is consistent across your dataset to maintain the integrity of your analysis.
Conclusion
Navigating the maze of duplicate labels in Pandas can be daunting, but with the right knowledge and tools, it's a challenge that can be overcome. By understanding how to identify and handle duplicate labels, you can ensure that your data analysis is both accurate and efficient. Remember, the goal is not just to find a way through the maze but to master it, turning potential obstacles into opportunities for deeper insight into your data. So, take these strategies and tips, and apply them to your datasets. With practice, you'll become adept at managing duplicate labels, making your data analysis process smoother and more reliable.
As you continue your journey through data analysis with Pandas, keep exploring, keep learning, and most importantly, keep experimenting. The path to mastery is through continuous improvement and adaptation. Happy data wrangling!
Recent Posts
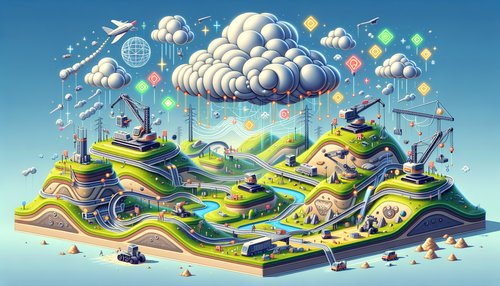
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
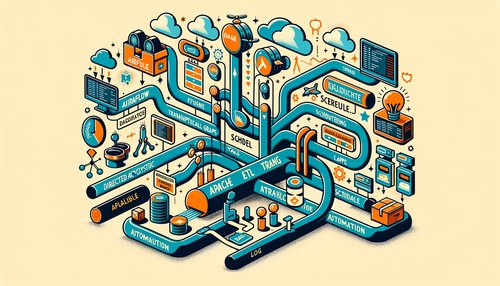
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow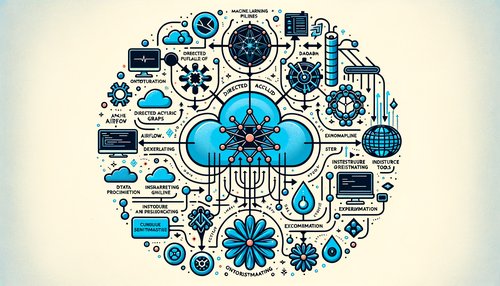
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow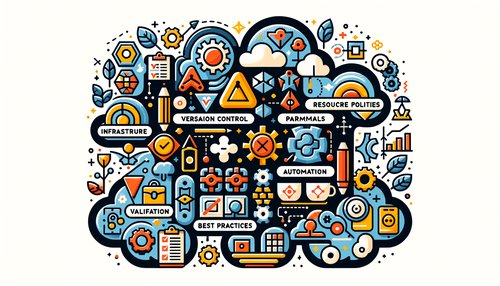
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
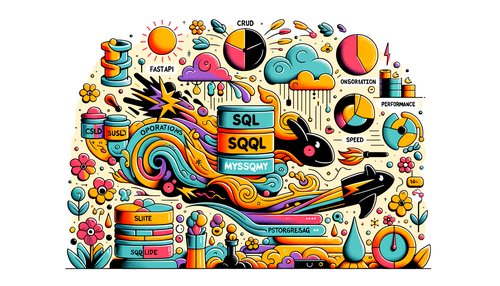