Mastering FastAPI: The Ultimate Guide to Harnessing Global Dependencies for Streamlined User Experiences
Welcome to the comprehensive guide on mastering FastAPI by leveraging global dependencies for creating streamlined user experiences. FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints, has revolutionized how we build and deploy web applications. This guide aims to dive deep into the concept of global dependencies within FastAPI, showcasing how they can be utilized to enhance application scalability, maintainability, and overall performance. Whether you're a seasoned developer or just starting, understanding how to effectively use global dependencies in FastAPI can significantly impact your web development projects. Let's embark on this journey to unlock the full potential of FastAPI together.
Understanding Global Dependencies in FastAPI
Before we delve into the practical applications, it's crucial to understand what global dependencies are in the context of FastAPI. Dependencies in FastAPI allow you to share logic (such as data validation, authentication, and database connections) between different parts of your application. Global dependencies, as the name suggests, are those that are applied to the entire application or a significant part of it. This means you can define common functionalities once and automatically apply them to multiple endpoints or routes, ensuring consistency and reducing redundancy across your codebase.
Benefits of Using Global Dependencies
- DRY Principle: Adhering to the "Don't Repeat Yourself" principle, global dependencies help in minimizing code repetition.
- Consistency: They ensure a uniform implementation of logic across your application.
- Efficiency: Streamlining common functionalities boosts the application's overall efficiency.
Implementing Global Dependencies in Your FastAPI Projects
Implementing global dependencies in FastAPI is straightforward yet powerful. Here's a step-by-step guide to help you get started:
Step 1: Define Your Dependency
Start by defining the functionality you want to apply globally. This could be anything from user authentication, database session management, to rate limiting. Here's a simple example of a dependency that ensures all requests have a valid API key:
from fastapi import Depends, HTTPException, status
def verify_api_key(api_key: str = Header(...)):
if api_key != "expected_api_key":
raise HTTPException(
status_code=status.HTTP_403_FORBIDDEN,
detail="Invalid API Key"
)
Step 2: Apply the Dependency Globally
Once your dependency is defined, you can apply it globally across your FastAPI application. This is done by adding it to the application instance using the .add_middleware()
method or the .dependency_overrides_provider()
for dependencies:
app = FastAPI()
app.dependency_overrides_provider(verify_api_key)
Practical Tips for Working with Global Dependencies
- Use Asynchronous Functions: When possible, define your dependencies as async functions to take advantage of FastAPI's asynchronous capabilities, improving performance.
- Test Thoroughly: Global dependencies affect the entire application. Ensure thorough testing to prevent unintended side effects.
- Document Your Dependencies: Clear documentation of the global dependencies in your project is crucial for maintainability and future development.
Advanced Techniques and Best Practices
Beyond the basics, mastering global dependencies in FastAPI involves understanding advanced techniques and best practices that can take your project to the next level:
Conditional Dependencies
Not all endpoints may require the same level of dependency application. Learn to apply dependencies conditionally to maintain flexibility without compromising on functionality.
Dependency Injection for Testing
Leverage FastAPI's dependency injection system to mock dependencies during testing. This allows for more robust and reliable tests by isolating the components under test.
Conclusion
Mastering global dependencies in FastAPI is a journey that can significantly enhance the quality and efficiency of your web applications. By understanding the core concepts, implementing them in your projects, and following best practices, you can create more maintainable, scalable, and user-friendly APIs. Remember, the key to success with FastAPI and its dependency system lies in thoughtful planning, consistent testing, and continuous learning. So, take these insights, apply them to your work, and watch your FastAPI projects thrive. Happy coding!
Final Thought: As you continue to explore the power of FastAPI, consider contributing to the community. Whether it's by sharing your own insights, contributing to open-source projects, or helping others troubleshoot their challenges, your expertise can help shape the future of FastAPI development.
Recent Posts
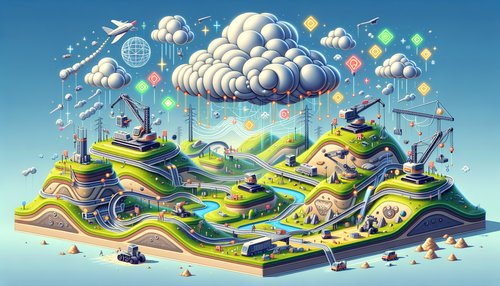
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
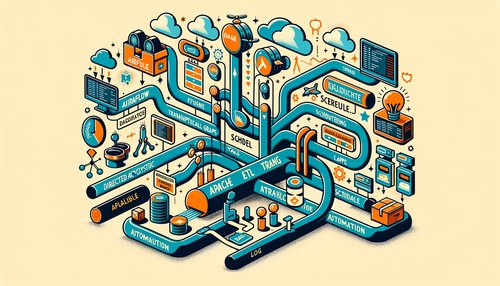
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow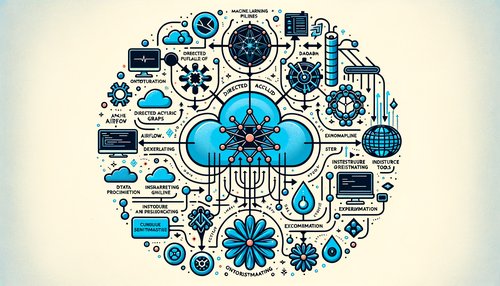
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow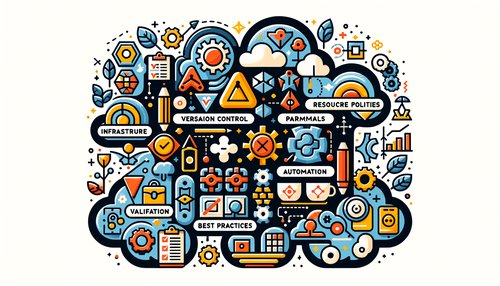
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
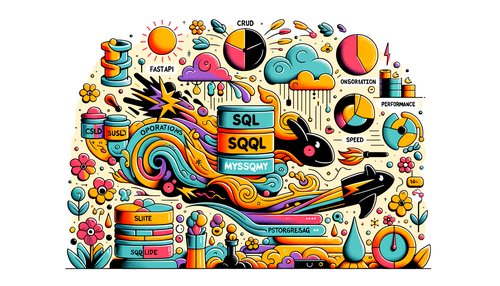