Mastering FastAPI: A Comprehensive Guide to Effortlessly Serving Static Files
Welcome to the ultimate guide on how to effortlessly serve static files with FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.6+ based on standard Python type hints. Whether you're building a complex application or just need a quick way to serve up images, CSS, or JavaScript, understanding how to efficiently manage static files is crucial. In this comprehensive guide, we'll cover everything you need to know to master this aspect of FastAPI, ensuring your web applications are both efficient and scalable.
Understanding Static Files in FastAPI
Before we dive into the how-to, it's important to understand what static files are and why they matter. Static files, unlike dynamic content generated by your application, do not change. They include files like images, CSS stylesheets, and JavaScript files. FastAPI, while primarily an API framework, provides robust support for serving these files, making it an excellent choice for full-stack web development.
Setting Up Your FastAPI Project
First things first, you need to set up a FastAPI project. This involves creating a virtual environment, installing FastAPI, and setting up an ASGI server like Uvicorn for running your application. Once your environment is ready, you can start building your application structure, keeping static files in mind. Here's a quick start:
$ mkdir myfastapiapp
$ cd myfastapiapp
$ python3 -m venv venv
$ source venv/bin/activate
(venv) $ pip install fastapi uvicorn
How to Serve Static Files
Serving static files with FastAPI is straightforward. You need to use the StaticFiles utility from the starlette.staticfiles module, which FastAPI is built upon. This involves mounting a StaticFiles instance to a specific path in your application. Here's how you can do it:
from fastapi import FastAPI
from fastapi.staticfiles import StaticFiles
app = FastAPI()
app.mount("/static", StaticFiles(directory="static"), name="static")
This code snippet mounts all files stored in the "static" directory under the "/static" path. So, if you have an image named "logo.png" in your "static" directory, it will be accessible at "
Best Practices for Managing Static Files
When serving static files, there are several best practices you should follow to ensure your application remains fast and secure:
- Use a dedicated directory: Keep all your static files in a separate directory (commonly named "static") to avoid confusion and potential security issues.
- Consider a CDN: For high-traffic applications, consider serving static files from a Content Delivery Network (CDN) to reduce load on your server and improve load times for users around the globe.
- Cache Control: Implement appropriate caching headers for your static files to reduce unnecessary network requests and speed up your application.
Advanced Techniques
As your FastAPI application grows, you might need to adopt more advanced techniques for serving static files. This could include using a separate static file server in production, integrating with cloud storage services like Amazon S3, or automating the build and deployment of frontend assets with tools like Webpack. Each of these approaches has its benefits and can help you optimize the performance and scalability of your application.
Conclusion
Serving static files is a fundamental aspect of web development, even in API-centric applications built with FastAPI. By following the guidelines and best practices outlined in this guide, you'll be well on your way to mastering this critical skill. Remember, the key to efficiently serving static files lies in understanding the tools and techniques at your disposal and choosing the right approach for your application's needs. Happy coding!
Whether you're a seasoned FastAPI developer or just starting out, mastering the art of serving static files is a valuable skill that will serve you well in all your web development endeavors. Keep experimenting, keep learning, and don't hesitate to dive into the FastAPI documentation for more in-depth information and advanced topics. Your perfect, high-performance web application is just around the corner!
Recent Posts
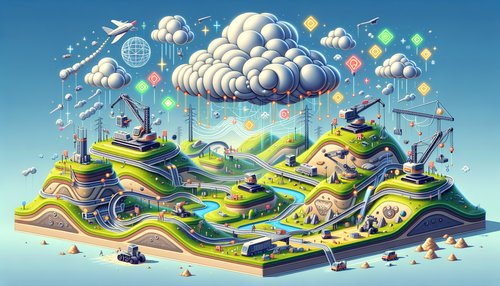
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
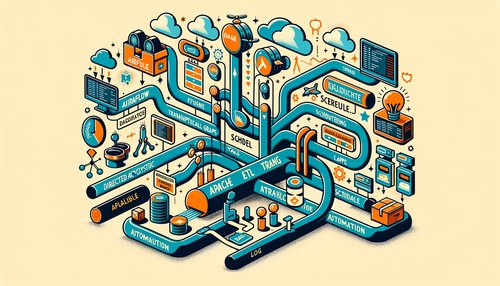
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow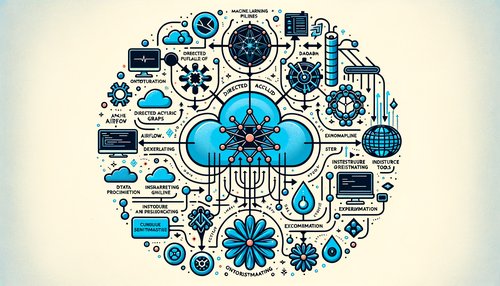
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow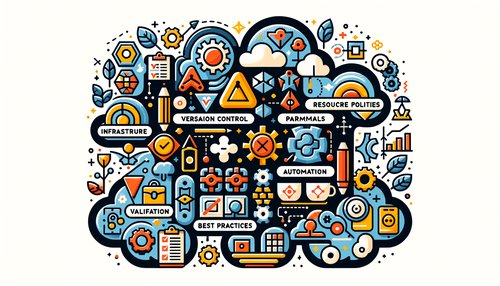
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
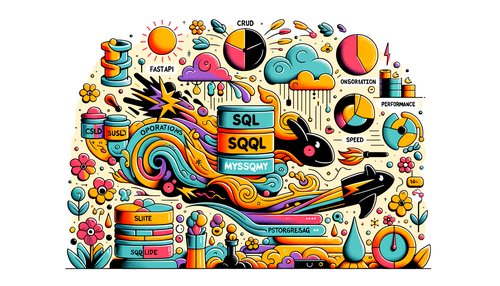