Mastering Pandas: A Comprehensive Guide to Merging, Joining, Concatenating, and Comparing Data Like a Pro
When it comes to data manipulation and analysis in Python, Pandas is the go-to library. It offers extensive capabilities for preparing, cleaning, and transforming data, making it easier for data scientists and analysts to extract insights and make data-driven decisions. However, mastering Pandas requires understanding its core functionalities, including merging, joining, concatenating, and comparing datasets. This guide will take you through these essential operations, providing practical tips, examples, and insights to elevate your data manipulation skills.
Merging DataFrames
Merging is a powerful feature in Pandas that allows you to combine different datasets based on common columns, similar to SQL joins. The merge()
function is versatile, supporting inner, outer, left, and right joins. An inner join combines rows from different DataFrames based on a key and includes only the rows with matching keys in both DataFrames. Conversely, an outer join includes all rows from both DataFrames, filling in missing values with NaNs where necessary.
Practical Tip: Always specify the type of join you need explicitly by using the how
parameter to avoid unexpected results.
import pandas as pd # Sample DataFrames df1 = pd.DataFrame({'Key': ['A', 'B', 'C'], 'Value1': [1, 2, 3]}) df2 = pd.DataFrame({'Key': ['B', 'C', 'D'], 'Value2': [4, 5, 6]}) # Merging DataFrames merged_df = pd.merge(df1, df2, on='Key', how='inner') print(merged_df)
Joining DataFrames
Joining is similar to merging but is based on the index rather than columns. The join()
method is ideal for combining data when you have one DataFrame's index that matches another DataFrame's column. By default, join()
performs a left join, but you can specify other types of joins using the how
parameter.
Example: Joining two DataFrames on their indexes.
# Assume df1 and df2 are defined as above but with indexes set to 'Key' df1.set_index('Key', inplace=True) df2.set_index('Key', inplace=True) joined_df = df1.join(df2, how='outer') print(joined_df)
Concatenating DataFrames
Concatenation is the process of appending one DataFrame to another. The concat()
function in Pandas can concatenate along a particular axis, either stacking DataFrames vertically (axis=0) or horizontally (axis=1). This operation is useful when you have data in similar structures and need to combine them into a single DataFrame.
Insight: Use the ignore_index=True
parameter to reindex the new DataFrame if the index doesn’t matter after concatenation.
# Concatenating df1 and df2 vertically concatenated_df = pd.concat([df1, df2], axis=0, ignore_index=True) print(concatenated_df)
Comparing DataFrames
Pandas also provides tools for comparing DataFrames, which is especially useful for identifying differences after data transformations or when validating data. The compare()
method returns a new DataFrame showing the changes from one DataFrame to another. It highlights what has been added, removed, or changed between the two DataFrames.
Example: Comparing two similar DataFrames to find differences.
df1_modified = df1.copy() df1_modified.loc[0, 'Value1'] = 100 # Change a value comparison_df = df1.compare(df1_modified) print(comparison_df)
Summary
Mastering the art of merging, joining, concatenating, and comparing data is crucial for any data professional working with Pandas. These operations form the backbone of data manipulation, enabling you to prepare, clean, and transform datasets effectively. By understanding and applying these techniques, you can unlock the full potential of your data, uncovering valuable insights and making informed decisions. Remember, practice is key to becoming proficient in these operations, so experiment with different datasets and scenarios to hone your skills.
Final Thought: Always strive to write clean and efficient code, leveraging the power of Pandas to simplify your data analysis tasks. Happy data wrangling!
Recent Posts
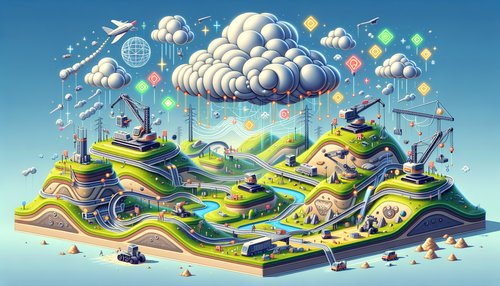
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
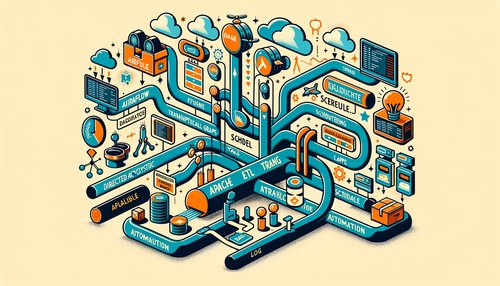
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow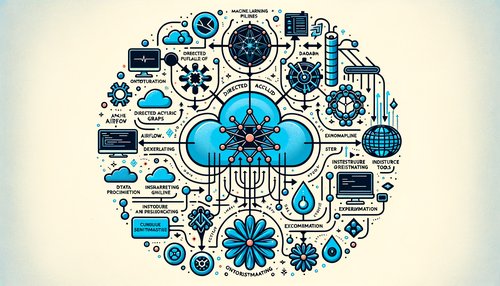
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow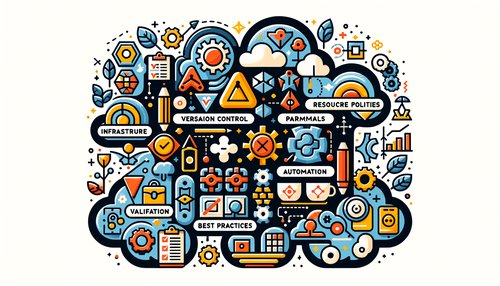
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
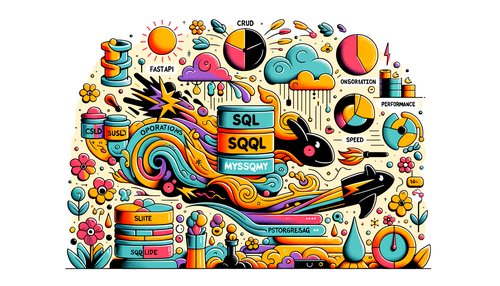