Using Django Forms to Streamline Data Collection and Processing
Django forms are a powerful tool for collecting and processing data. They provide a way to quickly create forms, capture and validate user input, and store the data in a database. With Django forms, developers can quickly create forms with minimal effort and provide users with an easy way to submit and manage their data.
Benefits of using Django forms
Using Django forms can help developers to save time and effort when creating forms. It also offers a number of benefits to users, including:
- Easy data capture - Django forms make it easy for users to submit their data quickly and accurately.
- Data validation - Django forms can be configured to validate user input, ensuring that the data entered is valid and accurate.
- Data storage - Django forms can be used to store data in a database, making it easy for developers to access and process the data.
Creating a Django Form
Creating a Django form is simple and straightforward. First, you need to create a form class that inherits from Django's built-in Form
class. This form class will contain the fields and validation rules for the form. Here is an example of a basic form class:
from django import forms
class ContactForm(forms.Form):
name = forms.CharField(max_length=100)
email = forms.EmailField()
message = forms.CharField(widget=forms.Textarea)
This form class defines three fields: name
, email
, and message
. Each field is defined using a built-in field type and can be configured with additional options, such as max_length
for the name
field and widget
for the message
field.
Submitting a Django Form
Once the form class has been created, the next step is to create a view that will handle the form submission. This view will need to be configured with a POST
method and should contain code to process the form data. Here is an example of a basic form submission view:
def contact_form_view(request):
if request.method == 'POST':
form = ContactForm(request.POST)
if form.is_valid():
# Process the form data
name = form.cleaned_data['name']
email = form.cleaned_data['email']
message = form.cleaned_data['message']
# Store the data in a database
# Send an email to the user
# Redirect to a success page
else:
form = ContactForm()
return render(request, 'contact_form.html', {'form': form})
This view first checks to see if the request is a POST
request. If it is, the form is instantiated with the submitted data and validated. If the form is valid, the data is processed and stored in a database. The view can also be used to send an email to the user and redirect to a success page.
Conclusion
Django forms are a great way to quickly and easily collect and process data. They provide a way to quickly create forms, validate user input, and store the data in a database. With Django forms, developers can save time and effort and provide users with an easy way to submit and manage their data.
Recent Posts
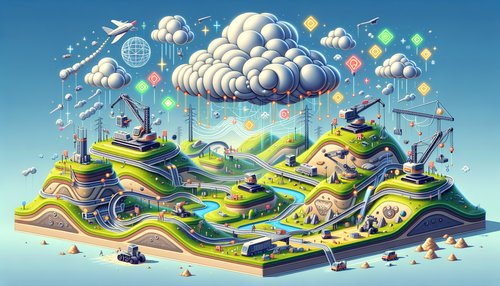
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
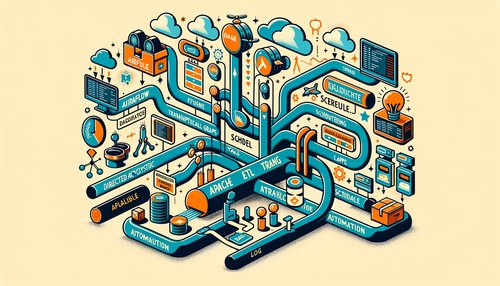
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow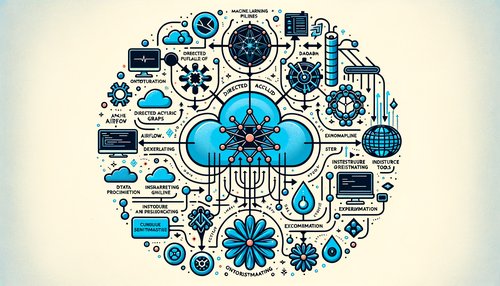
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow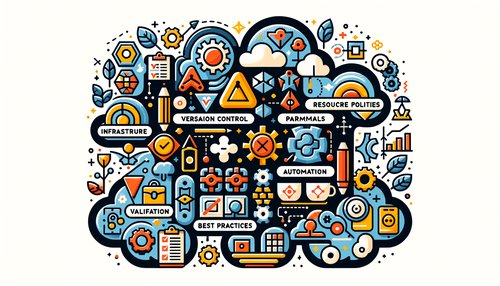
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
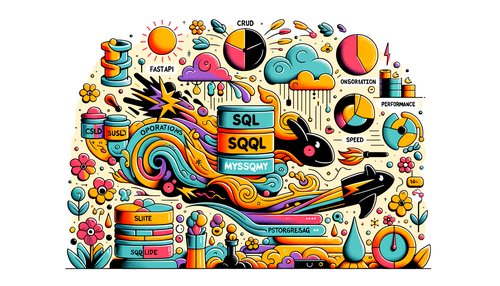