Using the Power of Django Rest Framework to Implement Seamless Pagination
Pagination is an essential component of web development, allowing developers to limit the amount of data that is returned to a user in a single request. This helps improve performance and usability by reducing the amount of data that needs to be transferred and processed at any given time. Using the Django Rest Framework (DRF), developers can quickly and easily implement pagination for their applications. DRF provides powerful tools for creating paginated APIs that are easy to use and highly customizable. In this blog post, we'll look at how to use DRF's built-in pagination classes to create a seamless pagination experience for users. The first step in creating a paginated API is to create a custom pagination class. DRF provides several built-in pagination classes that can be used to create a basic paginated API. For example, the LimitOffsetPagination class can be used to limit the number of results returned in a single request.# Custom pagination class class CustomPagination(LimitOffsetPagination): default_limit = 10 max_limit = 20Once the custom pagination class is created, it needs to be registered with the viewset. This can be done by setting the pagination_class attribute of the viewset to the custom pagination class.
# Register custom pagination class class MyViewSet(viewsets.ModelViewSet): pagination_class = CustomPaginationNext, the API endpoint needs to be configured to use the custom pagination class. This can be done by setting the pagination_class attribute of the API view to the custom pagination class.
# Configure API endpoint @api_view(['GET']) def my_view(request): paginator = CustomPagination() data = paginator.paginate_queryset(MyModel.objects.all(), request) serializer = MyModelSerializer(data, many=True) return paginator.get_paginated_response(serializer.data)Finally, the API response needs to be configured to provide pagination information to the user. DRF provides a built-in PaginationSerializer that can be used to add pagination information to the response.
# Configure API response @api_view(['GET']) def my_view(request): paginator = CustomPagination() data = paginator.paginate_queryset(MyModel.objects.all(), request) serializer = MyModelSerializer(data, many=True) response = paginator.get_paginated_response(serializer.data) response.data['pagination'] = PaginationSerializer(paginator, context={'request': request}).data return responseBy using the power of the Django Rest Framework, developers can easily create a seamless pagination experience for their users. With just a few lines of code, you can create a custom paginated API that is easy to use and highly customizable.
Recent Posts
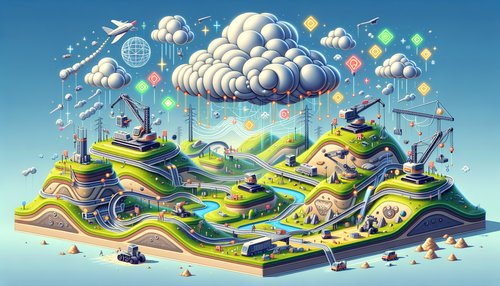
7 months ago
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
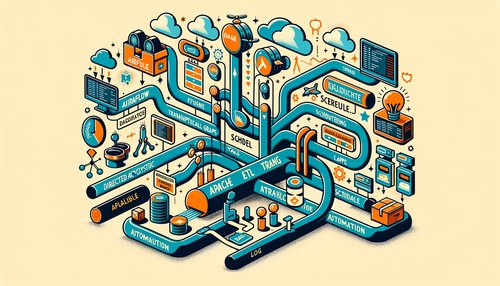
7 months, 1 week ago
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow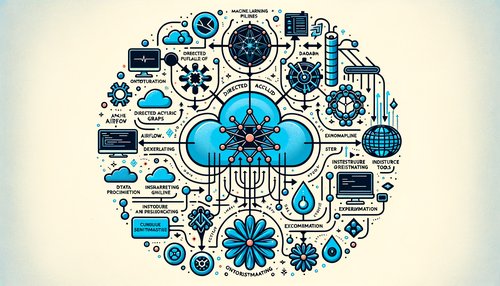
7 months, 1 week ago
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow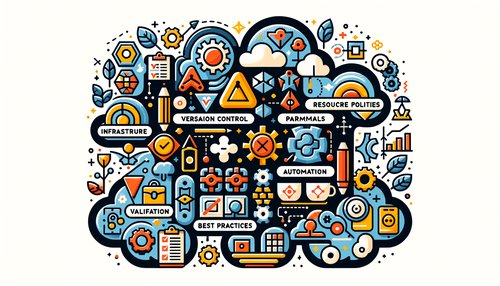
7 months, 1 week ago
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
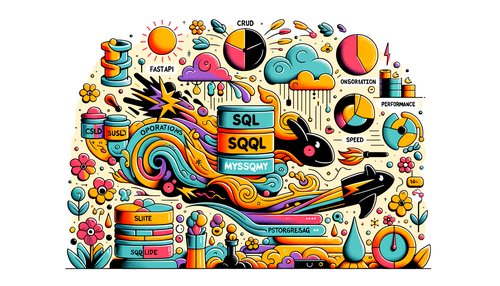
7 months, 1 week ago
Show All