Pioneering the Cloud: Mastering Terraform Plugin Development for Customized Infrastructure Solutions
In the ever-evolving landscape of cloud computing, Terraform has emerged as a powerful tool for infrastructure as code (IaC). One of the most compelling features of Terraform is its plugin system, which allows for extensive customization and flexibility. In this blog post, we'll explore how you can master Terraform plugin development to create bespoke infrastructure solutions tailored to your unique needs.
Introduction to Terraform and Its Plugin Architecture
Terraform, developed by HashiCorp, is widely used for defining and provisioning cloud infrastructure. Its strength lies in its extensible plugin architecture, which consists of providers and provisioners. Providers interact with APIs to manage resources, such as AWS EC2 instances or Azure VMs, while provisioners handle the post-creation configuration.
By developing custom plugins, you can extend Terraform's capabilities beyond the default sets, enabling you to manage any infrastructure, no matter how niche or specialized. This blog post will guide you through understanding, developing, and integrating your custom Terraform plugins.
Understanding the Anatomy of a Terraform Plugin
A Terraform plugin is essentially a Go application that interfaces with Terraform core. There are two types of plugins: providers and provisioners.
- Providers: These manage the lifecycle of resources: creating, reading, updating, and deleting resources via API interactions.
- Provisioners: These perform tasks on the resource after it's created, like configuration management and running scripts.
Setting Up Your Development Environment
Before you start coding your Terraform plugin, you need to set up your development environment. Here's a step-by-step guide:
- Install Go, the programming language used to write Terraform plugins.
- Install Terraform to test and run your plugin.
- Set up your workspace and get familiar with the Terraform plugin documentation.
Developing Your First Custom Provider
Creating a custom provider involves implementing several key components:
- Provider Schema: This defines the configuration and resources your provider will support.
- Resource Schema: These define the attributes and lifecycle methods for each resource.
- Client Setup: This involves setting up a client to interact with the API of the service you're managing.
Here’s a simplified example in Go:
func Provider() *schema.Provider {
return &schema.Provider{
Schema: map[string]*schema.Schema{
"api_key": &schema.Schema{
Type: schema.TypeString,
Required: true,
DefaultFunc: schema.EnvDefaultFunc("API_KEY", nil),
},
},
ResourcesMap: map[string]*schema.Resource{
"example_resource": resourceExample(),
},
}
}
Debugging and Testing Your Plugin
Testing is crucial in plugin development. Use the following techniques to ensure your plugin works correctly:
- Unit Testing: Write unit tests for individual components using Go’s testing package.
- Integration Testing: Verify that your plugin works with Terraform by creating real-world scenarios.
- Logging: Use detailed logging to diagnose issues during development.
Publishing and Maintaining Your Plugin
Once your plugin is developed and tested, you can publish it. Register your provider with the Terraform Registry to make it available to the community. Ensure you maintain and update your plugin regularly to handle API changes and add new features.
Practical Tips for Mastering Plugin Development
Here are some practical tips to help you become proficient in Terraform plugin development:
- Start Small: Begin with a simple resource and gradually add more complexity.
- Read the Source: Study existing plugins to learn best practices.
- Engage with the Community: Join Terraform forums and GitHub discussions to share knowledge and get feedback.
Conclusion
Mastering Terraform plugin development opens up a world of possibilities for customized infrastructure management. By understanding the plugin architecture, setting up your environment, and following best practices, you can create powerful and flexible solutions tailored to your needs. Dive into the community, start building, and transform your infrastructure management with Terraform.
Ready to get started? Explore the Terraform Plugin Development Documentation and begin your journey towards becoming a Terraform expert today!
Recent Posts
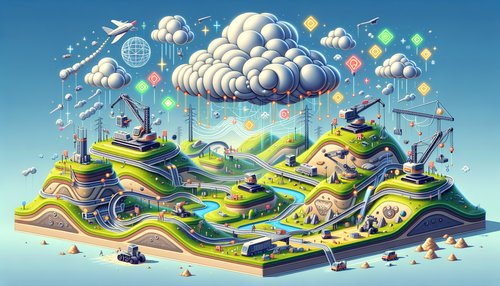
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
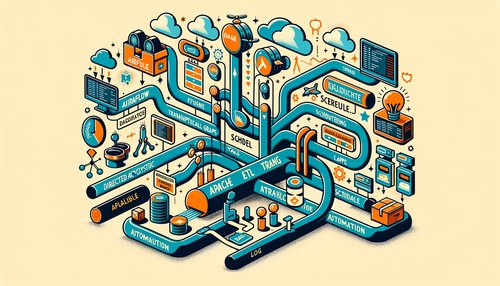
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow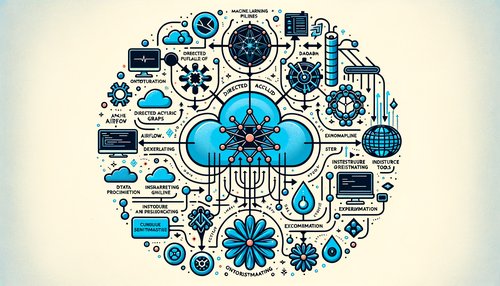
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow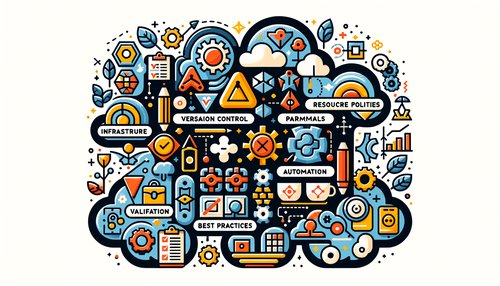
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
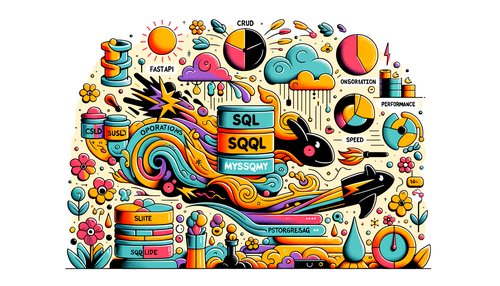