Supercharging Your React App with the Power of Hooks
React is a powerful JavaScript library for creating user interfaces for web applications. With React, developers can create high-performing, feature-rich applications that are easy to maintain. One of the greatest features of React is its ability to use hooks to easily access and manipulate data. Hooks are functions that allow developers to access and manipulate data in the React application without having to write complex code. In this blog post, we’ll explore how to use hooks to supercharge your React app.
What are Hooks?
Hooks are functions that allow developers to access and manipulate data in the React application without having to write complex code. They are used to access state, lifecycle methods, and other data within the React application. Hooks are a great way to make your React code more efficient and maintainable. They can be used to access data such as state, lifecycle methods, and other data without having to write complex code.
How to Use Hooks
Using hooks is relatively straightforward. First, you’ll need to import the hook from the React library. Then, you’ll need to create a function that will call the hook. Finally, you’ll need to call the hook in the component where you want to use it. Here’s a simple example of how to use a hook to access state in a React component:
import React, { useState } from 'react';
function MyComponent() {
const [count, setCount] = useState(0);
return (
<div>
<h1>{count}</h1>
<button onClick={() => setCount(count + 1)}>
Increment
</button>
</div>
);
}
In this example, we’re using the useState hook to access and manipulate the count state in our React component. We’re also using the setCount function to update the count state when the button is clicked.
Benefits of Using Hooks
Using hooks has several benefits over writing complex code. First, hooks make it easier to access and manipulate data in a React application. Second, hooks can help make your code more maintainable and efficient. Finally, hooks can help reduce the amount of code you need to write, making it easier to debug and test your application.
Conclusion
Hooks are a powerful feature of React that can help you supercharge your application. By using hooks, you can easily access and manipulate data in your React application without having to write complex code. With hooks, you can make your code more efficient and maintainable, as well as reduce the amount of code you need to write. Try using hooks in your React projects today and see how they can help you create amazing applications.
Recent Posts
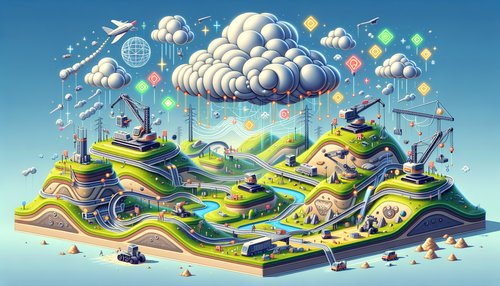
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
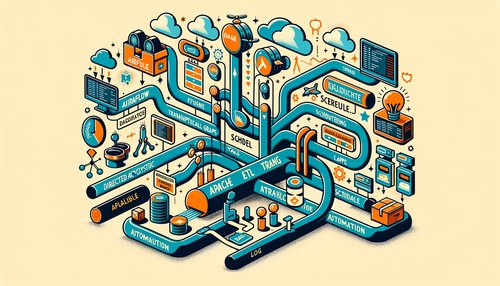
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow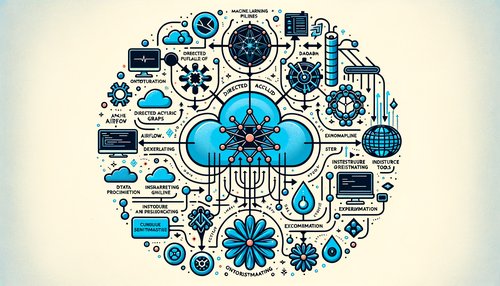
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow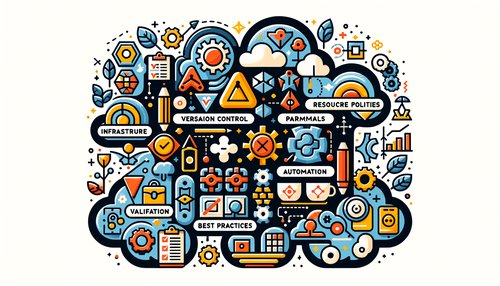
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
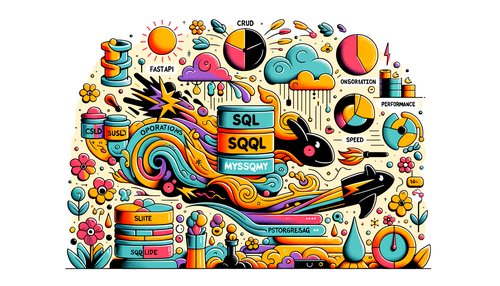