React: The Future of Web Development? Exploring the Advantages of the React Framework
React is a popular JavaScript library used for building user interfaces. It was created by Facebook and is now used by companies like Airbnb, Netflix, and Dropbox. React is known for its speed, scalability, and simplicity, making it an attractive choice for web developers.
In this blog post, we'll explore the advantages of the React framework and why it's becoming increasingly popular among web developers. We'll also look at some code snippets to demonstrate how React makes development easier and faster.
Advantages of React
React is a powerful and versatile framework that makes it easy to create dynamic web applications. It's fast, easy to learn, and has a large community of active users. Here are some of the key advantages of React:
-
Declarative: React is a declarative language, meaning that it uses a simple syntax for creating components. This makes it easy to read and understand, even for inexperienced developers.
-
Components: React components are self-contained pieces of code that can be reused across different projects. This makes it easier to maintain and scale projects without having to rewrite code.
-
Virtual DOM: React uses a virtual DOM, which makes it faster and more efficient than other frameworks. This means that React can quickly render changes to the UI without having to reload the entire page.
-
Reusability: React components can be reused across different projects, making it easy to maintain and scale projects without having to rewrite code.
Code Snippets
Here are some code snippets to illustrate how React makes development easier and faster:
Creating a Component
React components are self-contained pieces of code that can be reused across different projects. Here's an example of how to create a simple React component:
class MyComponent extends React.Component {
render() {
return <div>Hello World!</div>;
}
}
Rendering
React uses a virtual DOM to quickly render changes to the UI without having to reload the entire page. Here's an example of how to render a React component:
ReactDOM.render(<MyComponent />, document.getElementById('root'));
State Management
React provides an easy way to manage and update application state. Here's an example of how to use the useState
hook to manage state in a React component:
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
Conclusion
React is a powerful and versatile framework that makes it easy to create dynamic web applications. It's fast, easy to learn, and has a large community of active users. With its declarative syntax, components, virtual DOM, and reusability, React is becoming increasingly popular among web developers.
Recent Posts
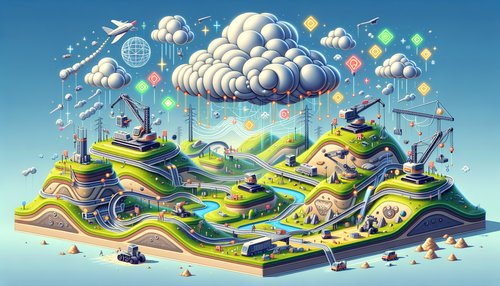
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
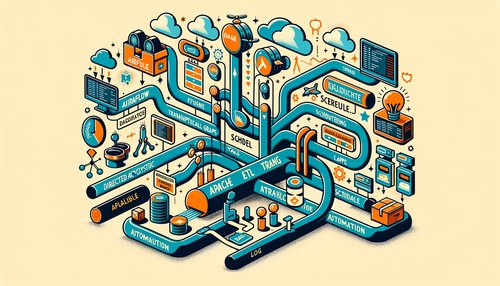
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow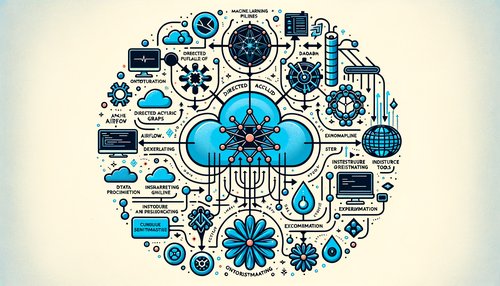
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow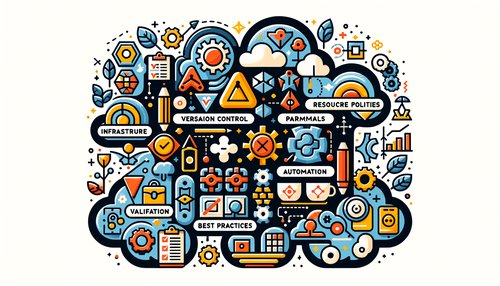
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
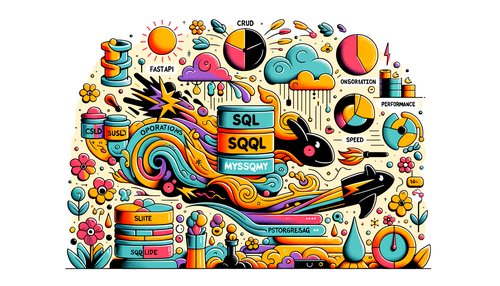