React: The New Kid on the Block? Examining the Latest Developments in the React Framework
React is a JavaScript library that's quickly becoming the go-to solution for web developers everywhere. It's used to create user interfaces (UI) for web and mobile applications and has become popular due to its easy-to-learn syntax, high performance, and flexibility. But what makes React so special? Let's take a look at the latest developments in the React framework to see what makes it stand out from the competition.
React Components
React is all about components. Components are small, reusable pieces of code that can be used to create complex user interfaces. They're like building blocks that allow developers to quickly create user interfaces without having to write a lot of code. React components are also highly customizable, allowing developers to change the look and feel of their applications without having to rewrite the entire codebase.
JSX
JSX is a syntax extension for React that allows developers to write HTML-like code inside of JavaScript. It makes it much easier to create React components and makes the code more readable. JSX is also faster than traditional JavaScript, which makes it an attractive choice for developers who want to create high-performance applications.
React Hooks
React Hooks are a new feature in React that allow developers to use state and other features of React without having to write a class. This makes it much easier to write components, as developers don't have to worry about writing complex classes. React Hooks also make it easier to reuse code and create components that are more maintainable.
React Suspense
React Suspense is a new feature that allows developers to render components asynchronously. This makes it much easier to create applications with faster loading times. React Suspense also makes it easier to create applications with more complex user interfaces, as developers can render components without having to wait for the data to be fetched.
React Native
React Native is a framework that allows developers to create native mobile applications using React. It makes it much easier to create mobile apps with React, as developers don't have to learn a new language. React Native also provides access to native device features, such as GPS and camera, which makes it easier to create more powerful mobile applications.
React is quickly becoming the go-to solution for web and mobile developers. Its components, JSX, React Hooks, React Suspense, and React Native make it an attractive choice for developers who want to create high-performance applications. With its easy-to-learn syntax, high performance, and flexibility, React is sure to remain the new kid on the block for a long time to come.
<div class="myComponent">
<h1>My Component</h1>
<p>This is my React component.</p>
</div>
import React, { useState } from 'react';
const MyComponent = () => {
const [name, setName] = useState('');
const handleChange = (e) => {
setName(e.target.value);
};
return (
<div className="myComponent">
<h1>My Component</h1>
<p>This is my React component.</p>
<input type="text" value={name} onChange={handleChange} />
</div>
);
};
export default MyComponent;
Recent Posts
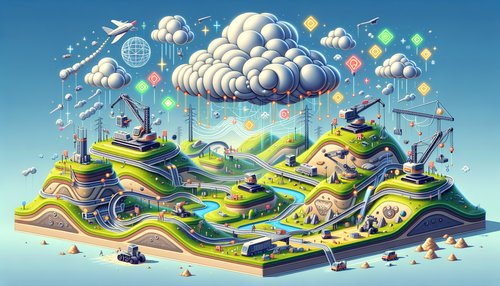
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
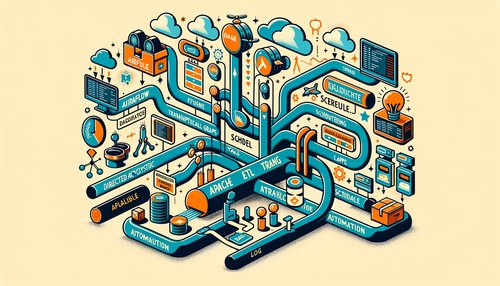
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow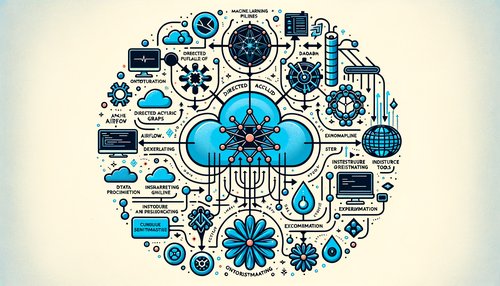
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow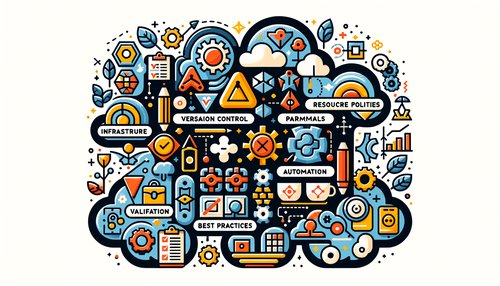
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
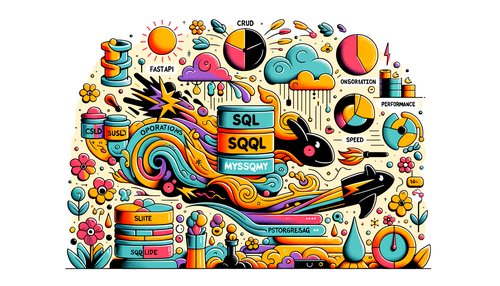