Revolutionizing Web Forms: How React Hook Form is Changing the Game for Developers
In the dynamic world of web development, efficiency and simplicity often dictate the success of a project. Enter React Hook Form, a lightweight library that is turning heads and transforming the way developers handle form validation and management in React applications. This blog post dives deep into the mechanics of React Hook Form, exploring its features, benefits, and how it stands out from other form libraries. Prepare to discover a tool that not only simplifies your code but also enhances your development workflow.
Understanding React Hook Form
At its core, React Hook Form is designed to reduce the amount of code needed to create complex forms while boosting performance. It leverages React hooks, enabling you to manage forms with minimal re-renders. This section will break down the key concepts and functionalities of React Hook Form, giving you a solid foundation to start integrating it into your projects.
Key Features and Advantages
React Hook Form stands out for its performance and developer-friendly approach. Some of its standout features include:
- Easy Integration: Seamlessly works with existing React projects, requiring minimal setup.
- Less Code: Simplifies form handling by minimizing the amount of boilerplate code.
- Better Performance: Utilizes uncontrolled components and native HTML inputs, reducing the number of re-renders.
- Powerful Validation: Supports simple to complex validation rules, including custom, asynchronous, and schema-based validation.
Getting Started with React Hook Form
Integrating React Hook Form into your project is straightforward. Begin by installing the library using npm or yarn:
npm install react-hook-form
Or:
yarn add react-hook-form
Then, use the useForm
hook to create a form instance and apply it to your form elements. This section will guide you through creating a basic form with validation.
Building a Form with React Hook Form
Creating forms is an integral part of developing web applications. React Hook Form makes this process intuitive and efficient. Here, we'll walk through the steps to create a user registration form with basic validation.
import React from 'react';
import { useForm } from 'react-hook-form';
function RegistrationForm() {
const { register, handleSubmit, errors } = useForm();
const onSubmit = data => console.log(data);
return (
);
}
This example demonstrates the ease of setting up a form with validation. Notice how we use the register
function to connect each input to the React Hook Form logic.
Advanced Techniques and Custom Validation
React Hook Form goes beyond basic form handling, offering powerful tools for custom and asynchronous validation, nested forms, and more. This section delves into advanced techniques, showcasing how to leverage React Hook Form's full potential.
For instance, custom validation allows you to define complex rules that go beyond the default constraints:
const { register, handleSubmit, errors } = useForm();
const validateUserName = async (value) => {
const userExists = await checkUserExists(value); // Assume this function checks the username against a database
return userExists ? 'Username is already taken' : true;
};
{errors.username && {errors.username.message}
}
This example highlights how you can incorporate asynchronous checks, such as verifying a username's uniqueness, directly into your form validation logic.
Why Developers Are Embracing React Hook Form
The adoption of React Hook Form among developers is growing rapidly, and for good reason. It offers a blend of performance, simplicity, and flexibility that is hard to find in other form libraries. By reducing the need for external state management and minimizing re-renders, React Hook Form delivers a smoother user experience and a more enjoyable development process.
Conclusion
React Hook Form represents a significant leap forward in the way developers handle forms in React applications. Its efficiency, ease of use, and robust validation capabilities make it an invaluable tool in any developer's arsenal. Whether you're building simple contact forms or complex multi-step forms, React Hook Form provides the functionality and flexibility needed to get the job done with less code and better performance.
As web development continues to evolve, tools like React Hook Form play a crucial role in streamlining workflows and enhancing user experiences. Embrace the change and consider integrating React Hook Form into your next project. The difference will be clear from the start.
Recent Posts
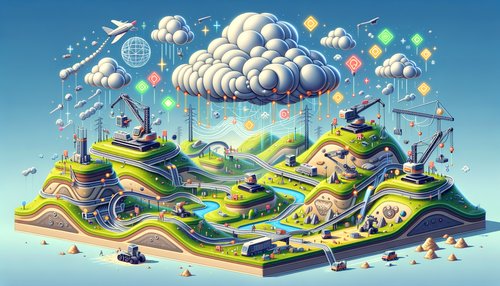
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
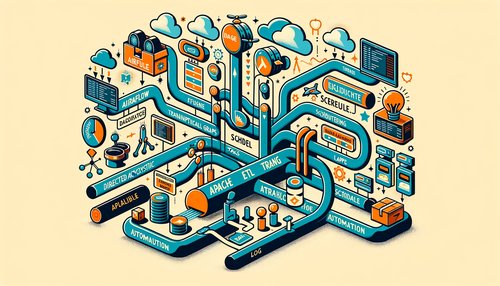
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow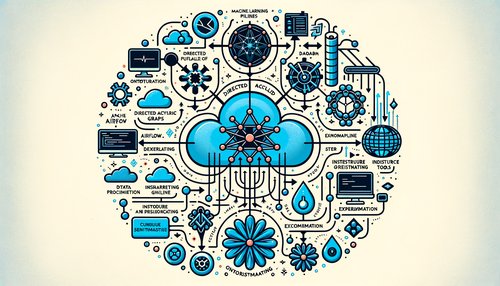
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow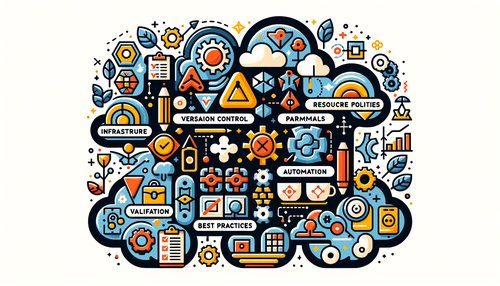
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
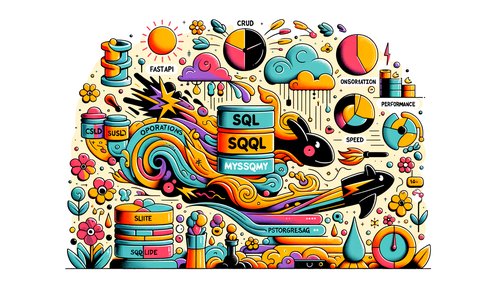