Securing Your First Steps: A Beginner's Guide to Implementing Security in FastAPI Applications
When stepping into the world of web development with FastAPI, a cutting-edge, high-performance web framework for building APIs with Python 3.7+, it's crucial to prioritize security from the get-go. In this comprehensive guide, we'll walk through the essential steps and best practices to secure your FastAPI applications, ensuring they're robust against common vulnerabilities and threats. Whether you're a beginner or looking to brush up on your security skills, this guide has got you covered.
Understanding the Basics of FastAPI Security
Before diving into the practical steps, it's essential to understand what makes FastAPI a unique choice for web development and how it handles security. FastAPI leverages modern security standards, including OAuth2 and OpenID Connect, offering built-in support for secure authentication and authorization mechanisms. By grasping these concepts early, you'll be better equipped to implement effective security measures in your applications.
Authentication vs. Authorization
Two fundamental aspects of web security are authentication and authorization. Authentication verifies the identity of a user, while authorization determines what an authenticated user is allowed to do. FastAPI provides tools and techniques to implement both, ensuring that you can control access to your application's resources effectively.
Securing Your API with HTTPS
One of the first steps in securing your FastAPI application is to enable HTTPS, which encrypts data in transit, protecting it from interception or tampering. While FastAPI itself does not enforce HTTPS, you can easily set it up using a reverse proxy like Nginx or by deploying your app on a platform that handles HTTPS for you, such as Heroku or AWS.
Using SSL/TLS Certificates
To implement HTTPS, you'll need an SSL/TLS certificate. There are several options available, including free certificates from Let's Encrypt, which are perfect for most applications. Configuring your server or deployment platform to use an SSL/TLS certificate is a critical step in safeguarding your API's data.
Implementing User Authentication
User authentication is a cornerstone of web application security. FastAPI provides several ways to implement it, from simple token-based authentication to more complex OAuth2 flows. Here's a basic example of how to set up token-based authentication in your FastAPI app:
from fastapi import FastAPI, Depends, HTTPException, status
from fastapi.security import OAuth2PasswordBearer
app = FastAPI()
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
async def get_current_user(token: str = Depends(oauth2_scheme)):
if token != "secrettoken":
raise HTTPException(
status_code=status.HTTP_401_UNAUTHORIZED,
detail="Invalid authentication credentials",
headers={"WWW-Authenticate": "Bearer"},
)
return {"user": "john doe"}
@app.get("/users/me")
async def read_users_me(current_user: dict = Depends(get_current_user)):
return current_user
This example demonstrates a simple way to protect an endpoint, requiring a valid token to access the user information. While this is a basic implementation, it's a solid starting point for understanding how authentication can be integrated into your FastAPI applications.
Authorization and Role-Based Access Control
Once you've authenticated users, the next step is to authorize them to access only the resources they're permitted to. Implementing role-based access control (RBAC) allows you to define roles for your users and permissions for those roles, ensuring users can only access the data and actions appropriate for their role. FastAPI's dependency system can be leveraged to create reusable dependencies that check a user's role before allowing access to an endpoint.
Securing Against SQL Injection and XSS Attacks
SQL Injection and Cross-Site Scripting (XSS) are common web security vulnerabilities that you must guard against. When using databases with FastAPI, ensure you're using ORM libraries like SQLAlchemy that automatically handle parameterized queries, significantly reducing the risk of SQL Injection attacks. To protect against XSS, sanitize any user input that will be rendered in the browser, and use templating engines that automatically escape variables.
Conclusion
Securing your FastAPI applications is an ongoing process that starts from the very first line of code. By implementing HTTPS, adding user authentication and authorization, and protecting against common vulnerabilities like SQL Injection and XSS, you're laying a strong foundation for a secure application. Remember, security is not a one-time task but a continuous effort to protect your application and its users. Stay informed about the latest security best practices and update your applications accordingly. Happy coding, and stay secure!
As you embark on your journey to build secure FastAPI applications, keep this guide as a handy reference. With each step you take, you're not only enhancing the security of your applications but also ensuring a safer experience for your users. Let's build with security in mind, right from the start!
Recent Posts
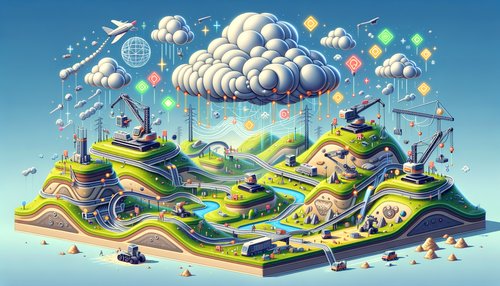
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
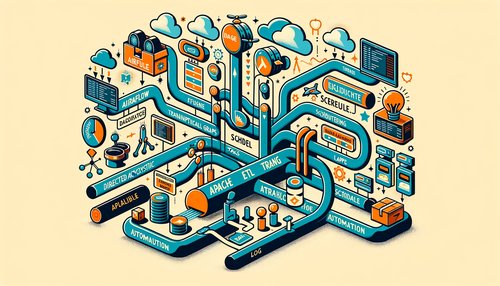
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow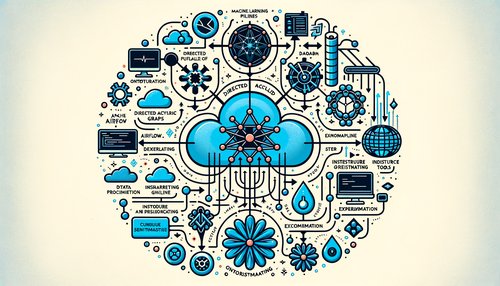
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow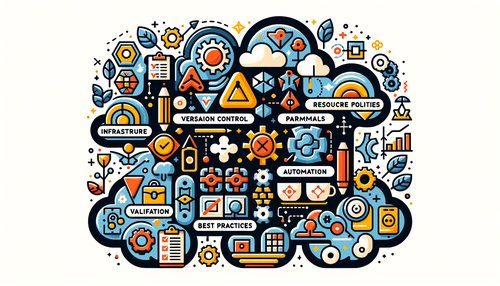
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
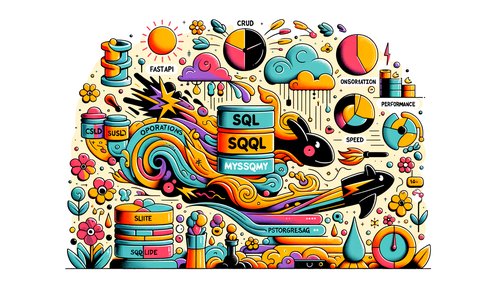