Supercharging Your FastAPI Applications: An In-Depth Guide to Background Tasks
FastAPI is already renowned for its speed and efficiency in building APIs. But did you know that you can make your FastAPI applications even more powerful by leveraging background tasks? Whether you're looking to offload intensive computations or perform recurring tasks, this guide will walk you through everything you need to know to manage background tasks effectively in FastAPI.
Introduction to Background Tasks in FastAPI
Background tasks allow you to execute logic in the background without blocking the main request loop. This can be a game-changer for applications that require high performance and scalability. In this section, we'll cover why background tasks are important and provide a brief overview of how FastAPI implements them.
Why Use Background Tasks?
Some common scenarios for using background tasks include:
- Sending emails after user registration
- Processing large data files
- Integrating with third-party APIs
- Running scheduled maintenance tasks
Background tasks can help improve user experience by freeing up the main thread to handle more user requests.
Setting Up Background Tasks in FastAPI
FastAPI makes it extremely simple to set up background tasks. Here is a step-by-step guide:
from fastapi import FastAPI, BackgroundTasks
app = FastAPI()
def background_task(name: str):
with open("text.txt", "a") as f:
f.write(f"Task for {name}\n")
@app.post("/send_notification/{name}")
async def send_notification(name: str, background_tasks: BackgroundTasks):
background_tasks.add_task(background_task, name)
return {"message": "Notification sent in the background"}
Real-World Use Cases
Let’s consider a few real-world applications of background tasks:
1. Sending Email Notifications
After a user registers on your platform, you might want to send a welcome email. Instead of making the user wait while the email is sent, you can use a background task to handle the email sending:
import smtplib
from email.mime.text import MIMEText
# Function to send email
def send_email(email: str):
msg = MIMEText("Welcome to our platform!")
msg["Subject"] = "Welcome"
msg["From"] = "noreply@yourdomain.com"
msg["To"] = email
with smtplib.SMTP("localhost") as server:
server.send_message(msg)
@app.post("/register/{email}")
async def register_user(email: str, background_tasks: BackgroundTasks):
background_tasks.add_task(send_email, email)
return {"message": "User registered successfully"}
2. Data Processing
If your application involves heavy data processing, you wouldn't want to block other user activities. Here's an example:
def process_data(file_path: str):
# Perform intensive data processing
data = perform_heavy_computation(file_path)
with open("processed_data.txt", "w") as f:
f.write(data)
@app.post("/upload_data")
async def upload_data(file: str, background_tasks: BackgroundTasks):
background_tasks.add_task(process_data, file)
return {"message": "Data processing started"}
Practical Tips and Best Practices
- Idempotency: Ensure your background tasks are idempotent. If they're run multiple times, they should produce the same result.
- Error Handling: Implement proper error handling and logging to troubleshoot any issues that arise during background processing.
- Resource Management: Be cautious about resource consumption, as background tasks can consume significant CPU and memory.
Conclusion
Background tasks are a powerful feature in FastAPI that can drastically improve the performance and responsiveness of your applications. By offloading intensive operations to the background, you can ensure a smoother and more efficient user experience. We hope this guide has given you valuable insights into setting up and using background tasks in FastAPI. Start implementing these tips and supercharge your FastAPI applications today!
Recent Posts
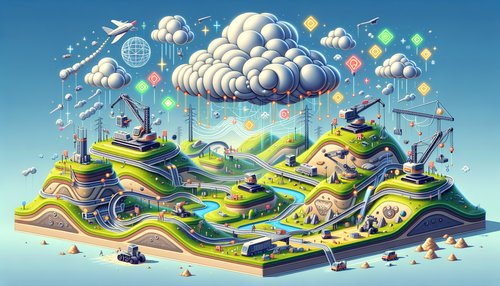
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
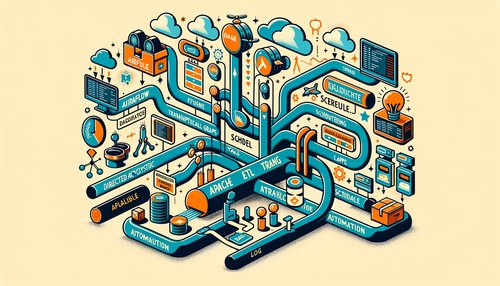
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow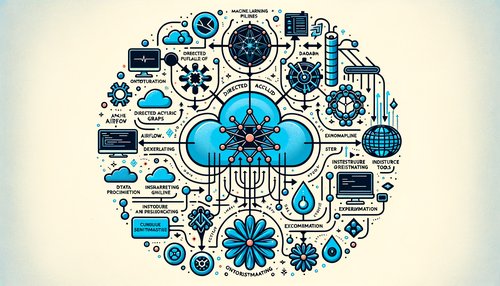
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow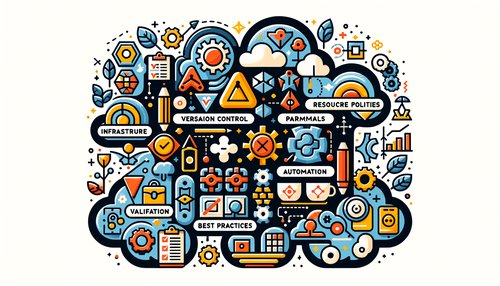
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
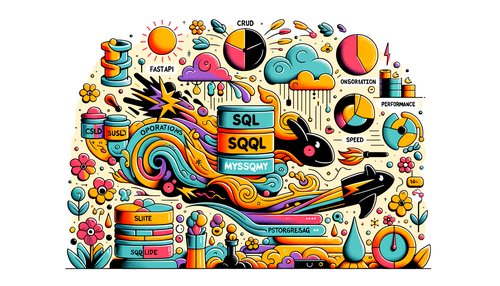