Unleashing Efficiency in Data Analysis: Exploring the Copy-on-Write Feature in the Pandas User Guide
Data analysis is a critical process in the modern world, driving decisions in sectors ranging from business to science. As data sets grow increasingly large and complex, efficiency in data manipulation and analysis becomes paramount. One of the tools at the forefront of addressing these challenges is Pandas, a powerful Python library for data analysis. This post delves into an often-overlooked feature that significantly enhances efficiency: the Copy-on-Write (CoW) mechanism. We'll explore what it is, how it works, and how you can leverage it to speed up your data analysis tasks.
Understanding the Copy-on-Write Mechanism
At its core, the Copy-on-Write mechanism is a resource-management technique used to minimize the overhead of copying data. Instead of creating a full copy of an object every time a modification is needed, CoW defers the copying until the first write operation occurs. This approach means that if the data is never modified, no copy is ever made, saving both time and memory.
How Pandas Implements Copy-on-Write
In the context of Pandas, the CoW feature plays a crucial role in handling DataFrame and Series objects efficiently. When you perform operations that would seemingly duplicate data, Pandas intelligently manages memory by employing CoW, making these operations much faster and less memory-intensive than they might appear at first glance.
For example, when you slice a DataFrame to select a subset of rows, Pandas returns a view on the original data rather than a complete copy. It's only when you modify this subset that Pandas applies the CoW mechanism, creating a copy of the relevant data at that moment.
Practical Tips for Leveraging CoW in Pandas
- Minimize Unnecessary Copies: Be mindful of operations that trigger a copy. Understanding when Pandas is likely to use CoW can help you structure your code to avoid unnecessary data duplication.
- Use Views Wisely: When working with large datasets, consider working with views as much as possible. This approach can significantly reduce memory consumption and speed up your analyses.
- Monitor Memory Usage: Keep an eye on your script's memory usage, especially when working with large DataFrames. Tools like
memory_profiler
can help identify when unexpected copies are being made.
Copy-on-Write in Action: An Example
Let's consider a simple example to illustrate the CoW mechanism in Pandas:
import pandas as pd # Create a large DataFrame df = pd.DataFrame({'A': range(1000000), 'B': range(1000000, 2000000)}) # Select a subset of the DataFrame subset = df[:100] # Modify the subset subset['A'] = subset['A'] * 2
In this example, the modification to subset
does not immediately affect df
. Instead, Pandas applies the CoW mechanism at the point of modification, ensuring that the original DataFrame remains unchanged while efficiently managing memory usage.
Conclusion: The Power of Copy-on-Write in Pandas
The Copy-on-Write feature in Pandas is a powerful yet underappreciated tool that can significantly enhance the efficiency of your data analysis workflows. By understanding how and when CoW is applied, you can write more efficient, faster, and memory-friendly code. As we've seen, leveraging CoW effectively requires a mix of strategic coding practices and a keen awareness of your data manipulation processes.
In conclusion, whether you're a seasoned data scientist or just starting out, taking the time to understand and leverage the Copy-on-Write mechanism in Pandas can be a game-changer for your data analysis projects. So, dive into your data, apply these insights, and unlock new levels of efficiency in your analyses.
Recent Posts
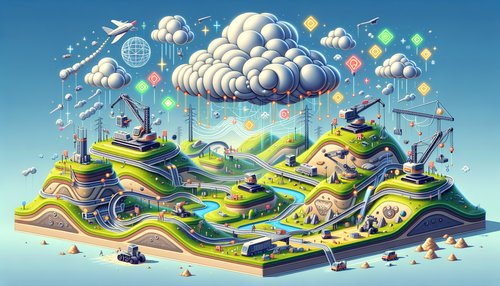
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
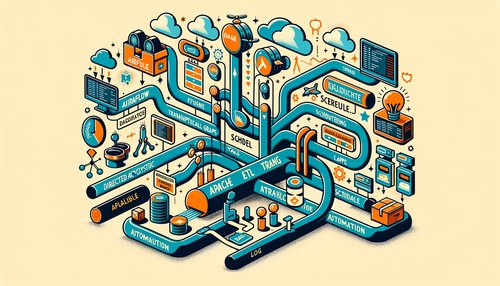
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow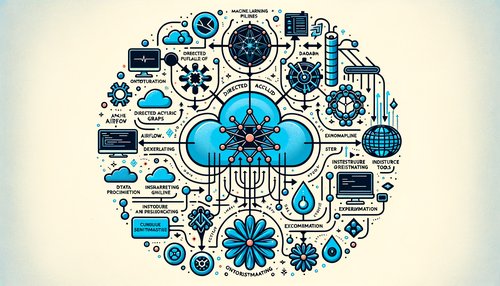
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow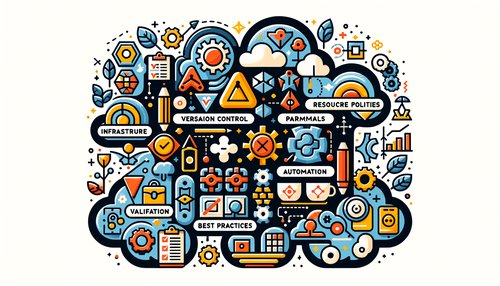
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
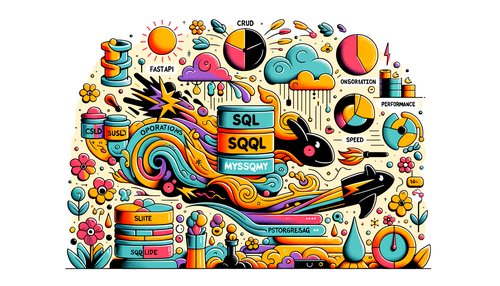