Unleashing the Power of FastAPI: Mastering Dependencies and Elevating Your Projects with Classes as Dependencies - A Comprehensive User Guide
Welcome to the world where speed meets reliability in web development. FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+, has been a game-changer for many developers. In this comprehensive guide, we delve into one of FastAPI's most powerful features—dependencies, with a special focus on using classes as dependencies. By the end of this journey, you'll not only understand the concept but also master the skills needed to elevate your projects to new heights.
Understanding Dependencies in FastAPI
Dependencies in FastAPI are a way to share logic between different parts of your application, such as database connections, authentication, and more. They allow you to write reusable components that can be injected into your path operations (routes). This not only promotes code reusability but also enhances testability and maintenance.
Why Use Classes as Dependencies?
Using classes as dependencies in FastAPI projects brings structure and scalability. It encapsulates related functions and data in a single, coherent unit, making your code cleaner and more Pythonic. Moreover, class-based dependencies leverage the power of dependency injection to provide flexibility and decoupling of components, leading to more robust and easily testable code.
Implementing Class-Based Dependencies
Implementing class-based dependencies involves creating a class that contains the logic or data you want to share across your application. FastAPI's dependency injection system will then take care of providing an instance of this class wherever it's needed.
Step-by-Step Guide
- Define a Class: Start by defining a class that encapsulates the functionality you wish to reuse.
- Declare Dependency Functions: Within the class, declare methods that will be used as dependencies. These methods can access the class's attributes and other methods, providing a rich context for your route operations.
- Inject Class Instances: Use FastAPI's Depends utility to inject instances of your class into your path operations. This is where the magic of dependency injection shines, allowing your routes to access shared logic and data effortlessly.
Practical Example
Let's consider a simple example to illustrate this concept. Imagine you're building an API for a blog, and you need to authenticate users across multiple routes:
from fastapi import FastAPI, Depends
app = FastAPI()
class UserAuth:
def __init__(self, db):
self.db = db
async def verify_user(self, username: str, password: str):
user = self.db.get_user(username)
if user and user.check_password(password):
return True
return False
# Dependency
def get_user_auth(db=Depends(get_db)):
return UserAuth(db)
@app.post("/login")
async def login(username: str, password: str, auth: UserAuth = Depends(get_user_auth)):
if await auth.verify_user(username, password):
return {"message": "User authenticated"}
return {"message": "Authentication failed"}
This example demonstrates how a class (UserAuth) encapsulating authentication logic can be injected into routes, making the code modular and reusable.
Best Practices and Tips
- Keep Dependencies Lean: Ensure your dependency classes are focused and lean. Avoid bloating them with unrelated functionality.
- Use Dependency Injection Wisely: While dependency injection is powerful, overuse can make your code harder to follow. Use it where it truly benefits your application's architecture.
- Test Your Dependencies: Leverage FastAPI's testing capabilities to test your dependencies thoroughly. This ensures they work as expected and helps maintain a stable codebase.
Conclusion
Mastering the art of using classes as dependencies in FastAPI can significantly enhance the quality and maintainability of your projects. By encapsulating shared logic and data, you promote reusability, scalability, and testability throughout your application. Remember, the goal is to write code that's not only functional but also clean and maintainable. Embrace these practices, and watch your FastAPI projects soar to new heights.
Now that you're equipped with this knowledge, it's time to put it into action. Experiment with class-based dependencies in your next FastAPI project and unleash the full potential of this powerful feature. Happy coding!
Recent Posts
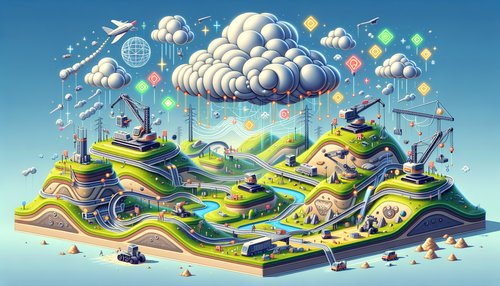
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
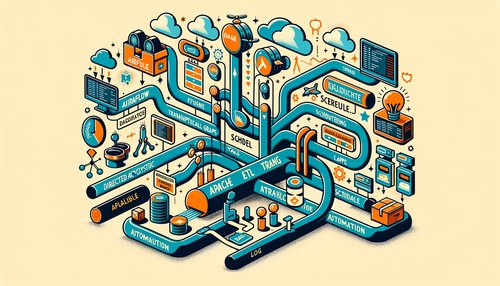
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow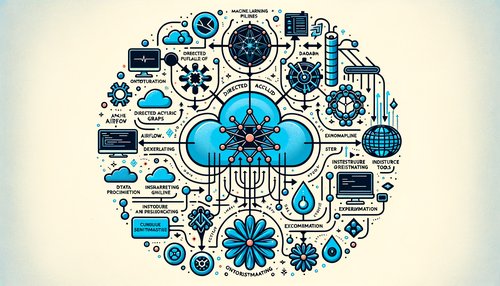
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow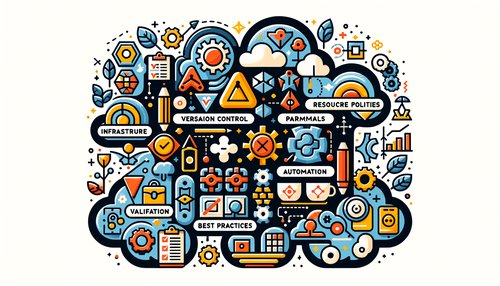
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
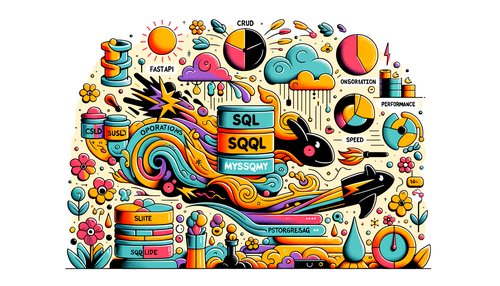