Unleashing the Power of FastAPI Middleware: Boost Your API Performance and Functionality
In the fast-paced world of modern web development, building efficient and scalable APIs is crucial. FastAPI, a modern and high-performance web framework for building APIs with Python 3.6+ based on standard Python type hints, provides developers with the tools they need to create robust applications. One of these powerful tools is middleware, which can enhance the performance and functionality of your API. In this post, we will explore the incredible benefits of FastAPI middleware, illustrate how to implement it effectively, and provide practical tips to boost your API's capabilities.
Understanding FastAPI Middleware
Middleware is software that provides common services and capabilities to applications beyond what's offered by the operating system. In the context of FastAPI, middleware is a feature that allows developers to execute code following every request before it reaches the endpoint or after the response has been sent back. This can be incredibly useful for cross-cutting concerns such as logging, authentication, or error handling.
By incorporating middleware into your FastAPI application, you can streamline processes, reduce code duplication, and enforce policy-based implementations that are always consistent across your applications.
Implementing Middleware in FastAPI
Implementing middleware in FastAPI is incredibly straightforward. You can create a middleware function by defining a new class and using FastAPI's @app.middleware('http')
decorator. This function must accept three parameters: the request, call_next, and response, of which the call_next is used to pass the request onto the next middleware or route handler.
from fastapi import FastAPI
from starlette.requests import Request
from starlette.responses import Response
app = FastAPI()
@app.middleware("http")
async def add_custom_header_middleware(request: Request, call_next):
response: Response = await call_next(request)
response.headers['X-Custom-Header'] = 'Value'
return response
In this example, every response from the server will contain a custom header called 'X-Custom-Header'. Middleware can be tailored as needed to modify requests, responses, or to perform checks before the request reaches the endpoint.
Practical Uses and Tips for FastAPI Middleware
Global Request Logging
Logging is an essential part of any application, and middleware provides a centralized place to implement logging across your application. By capturing and recording every incoming and outgoing request, you can easily gather data for analytics and debugging.
from fastapi import FastAPI
from starlette.requests import Request
app = FastAPI()
@app.middleware("http")
async def log_requests_middleware(request: Request, call_next):
print(f"Incoming request to: {request.url}")
response = await call_next(request)
print(f"Response status code: {response.status_code}")
return response
Cross-origin Resource Sharing (CORS)
FastAPI simplifies handling CORS with the fastapi.middleware.cors.CORSMiddleware
. You can set it up to control which domains are allowed to access resources on the server, along with various methods and headers.
from fastapi.middleware.cors import CORSMiddleware
app.add_middleware(
CORSMiddleware,
allow_origins=["*"],
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"]
)
This configuration permits all origins to make requests, which is handy during development. It is recommended to restrict origins for production environments.
Performance Considerations
While middleware can significantly enhance functionality, inappropriate use can affect performance. It is advisable to:
- Minimize middleware that performs intensive operations.
- Ensure asynchronous operations to avoid blocking the event loop.
- Avoid altering the request or response more than needed.
The Future of APIs with FastAPI Middleware
FastAPI's capabilities with middleware open numerous opportunities for building powerful, scalable, and efficient APIs. As you grow your applications, leveraging middleware will be essential in managing cross-cutting concerns, integrating seamlessly with other frameworks and tools, and maintaining a clean architecture.
Conclusion
Middlewares bring an enormous potential to your FastAPI applications, allowing for flexible, clean, and reusable structures that enhance functionality and performance. With straightforward implementation and significant advantages, they should be a staple in your toolkit when working with FastAPI. Start integrating FastAPI middleware today, and experience the difference it can make to your API development.
If you haven’t yet explored middleware in your projects, take this as a call to action. Experiment with the examples provided and see the powerful benefits middleware can offer in increasing both the capability and performance of your APIs.
Recent Posts
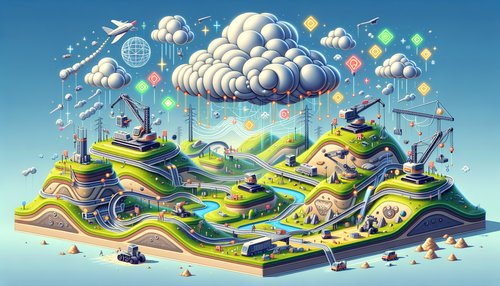
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
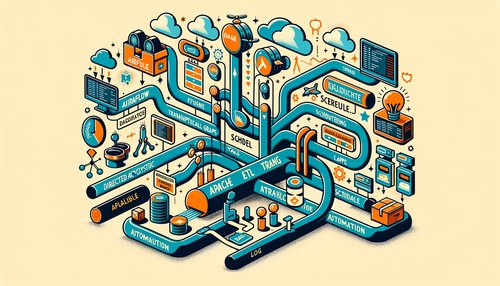
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow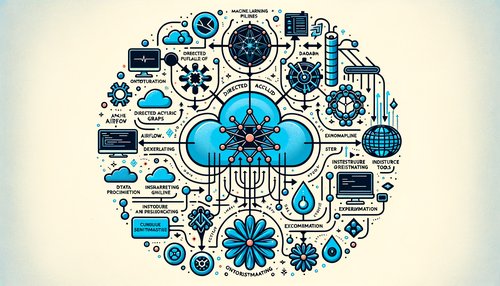
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow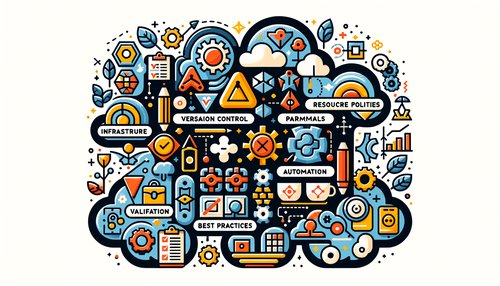
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
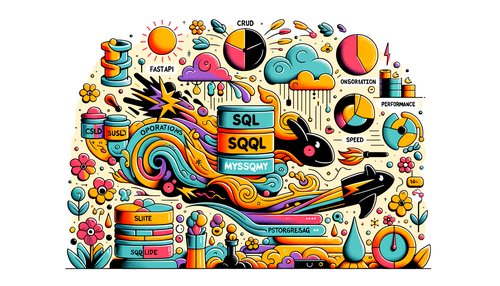