Unlock Lightning Speed: The Ultimate Guide to Enhancing Performance with Pandas!
Welcome to the fast lane! If you're on a quest to supercharge your data analysis projects, you've come to the right place. This guide is your ticket to unlocking lightning speed with Pandas, the powerful Python library that has become the gold standard for data manipulation and analysis. Whether you're a data science enthusiast, a professional analyst, or anyone in between, enhancing your Pandas performance can dramatically improve your productivity and efficiency. In this comprehensive guide, we'll dive into a series of strategies and tips to help you make the most out of Pandas. So buckle up, and let's boost your data processing to unprecedented speeds!
Understanding Pandas Performance
Before we turbocharge your Pandas skills, it's crucial to understand what affects its performance. Pandas is designed for ease of use and flexibility, but certain operations can be computationally expensive or memory-intensive. Knowing how Pandas handles data under the hood will empower you to make informed decisions about optimizing your code.
Choosing the Right Data Types
One of the simplest yet most effective ways to enhance Pandas performance is by being mindful of data types. Pandas defaults to using higher memory data types, but often, your data doesn't require that much precision. For instance, switching from the default float64
to float32
or choosing appropriate integer types can significantly reduce memory usage and speed up computations.
# Example: Optimizing data types
import pandas as pd
df = pd.DataFrame({
'A': pd.Series([1, 2, 3], dtype='int32'),
'B': pd.Series([4.0, 5.5, 6.1], dtype='float32')
})
Leveraging Categorical Data
When working with string data that takes on a limited set of values, converting it to a categorical type can yield substantial performance gains. Categorical data requires less memory and makes operations like sorting and grouping much faster.
# Example: Converting to categorical
df['Category'] = df['Category'].astype('category')
Using Vectorized Operations
One of Pandas' strengths is its ability to perform operations on entire arrays of data without explicit Python loops. This is known as vectorization, and it's a key to achieving high performance. Whenever possible, use Pandas' built-in functions or numpy arrays for computations instead of iterating over DataFrame rows.
Minimizing Chain Indexing
Chain indexing (e.g., df[a][b]
) can lead to performance issues due to its potential to return a copy rather than a view of the DataFrame. This not only slows down your operations but can also lead to unexpected results. Opt for using .loc
, .iloc
, or .at
methods to access DataFrame elements more efficiently and safely.
Efficiently Reading and Writing Data
The way you read and write data can also impact performance. When dealing with large datasets, consider using pd.read_csv()
with the chunksize
parameter to process data in manageable chunks. Similarly, when writing data, using the to_csv()
method with the compression='gzip'
parameter can save both disk space and time.
Conclusion
Unlocking lightning speed in Pandas is all about understanding its inner workings and applying best practices to your data manipulation and analysis tasks. By choosing the right data types, leveraging categorical data, using vectorized operations, minimizing chain indexing, and efficiently reading and writing data, you can achieve significant performance gains. Remember, the key to speed is not just about writing faster code, but writing smarter code. Now that you're armed with these tips and strategies, it's time to put them into action and watch your Pandas projects soar to new heights of efficiency and speed!
Happy data wrangling!
Recent Posts
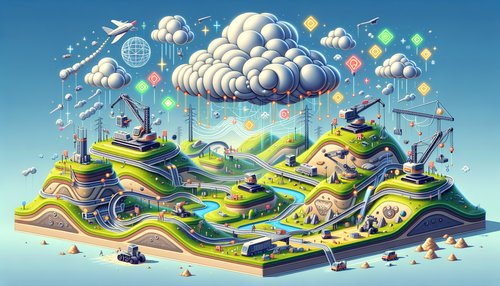
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
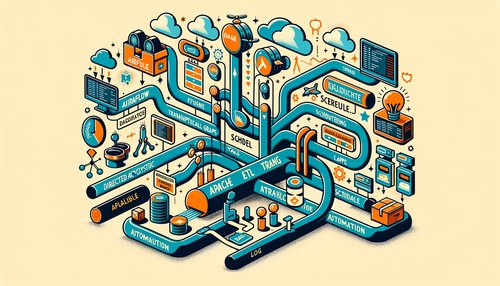
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow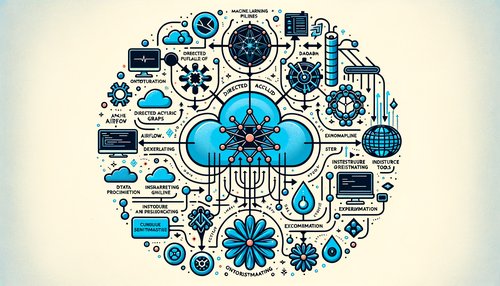
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow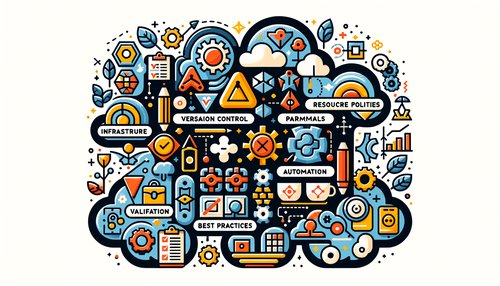
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
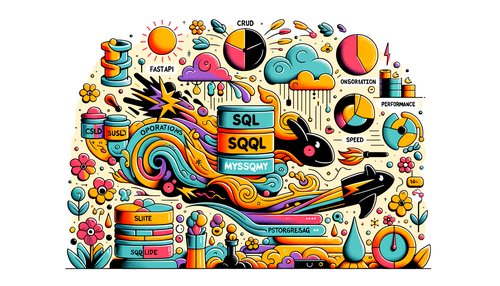