Unlock the Power of Angular Routing for Your Web App!
Angular routing is a powerful tool for creating dynamic web applications. It enables developers to create and manage multiple views within a single page application (SPA). With Angular routing, developers can easily and quickly switch between different views without having to reload the entire page. This makes for a faster and more efficient user experience.
Angular routing consists of two parts: the router and the routes. The router is responsible for managing the routes and deciding which view to display. The routes are defined in the router configuration, which consists of a list of paths and associated components. When a user navigates to a route, the router will display the associated component.
To get started with Angular routing, you will first need to set up the router configuration. This can be done with the RouterModule.forRoot()
method. The method takes an array of routes as its argument. Each route consists of a path and a component. The path is the URL that the router will match against and the component is the view that will be displayed.
For example, if you wanted to set up two routes: /home
and /about
, you could do so as follows:
const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent }
];
@NgModule({
imports: [
RouterModule.forRoot(routes)
],
exports: [
RouterModule
]
})
export class AppRoutingModule { }
Once you have set up the router configuration, you can use the <router-outlet>
directive to tell the router where to render the component associated with the matched route. This directive should be placed in the component template where the component should be rendered.
For example, if you wanted to render the HomeComponent
when the /home
route is matched, you could do so as follows:
<router-outlet></router-outlet>
Angular routing also provides additional features such as route guards, which can be used to protect certain routes from being accessed by unauthorized users. Route guards are implemented as services and can be used to restrict access to certain routes.
In conclusion, Angular routing is a powerful tool for creating dynamic web applications. It enables developers to easily and quickly switch between different views without having to reload the entire page. With the router configuration and the <router-outlet>
directive, developers can quickly set up routes and render the associated component. Additionally, route guards can be used to protect certain routes from being accessed by unauthorized users.
Recent Posts
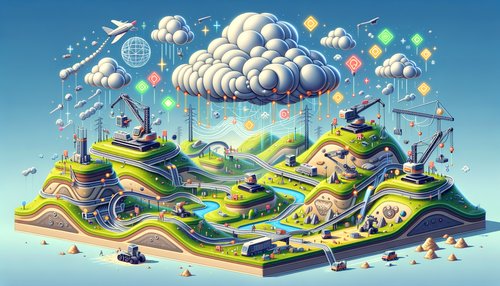
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
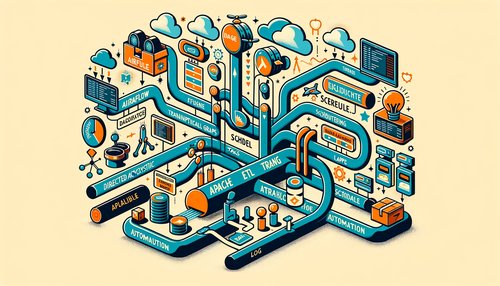
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow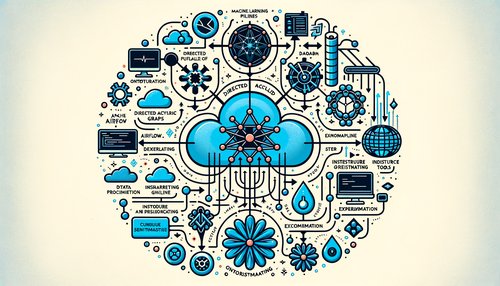
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow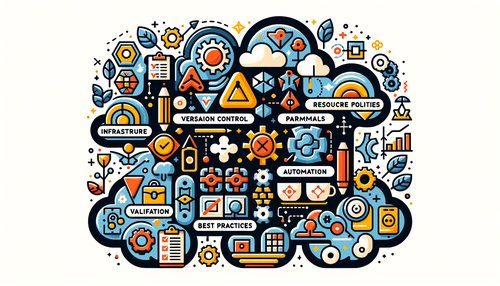
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
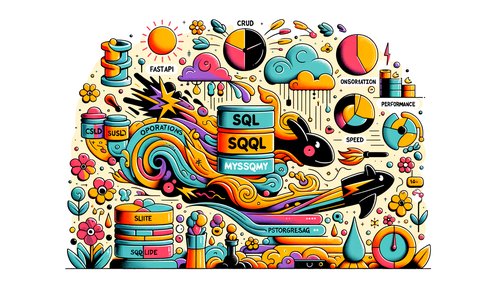