Unlock the Power of Data in Just 10 Minutes: A Quickstart Guide to Pandas!
Welcome to the fast lane of data analysis! If you've ever felt overwhelmed by data or thought that manipulating and analyzing big datasets was the exclusive domain of seasoned data scientists, this guide is for you. In the next 10 minutes, you're not just going to learn about Pandas; you're going to experience the sheer power and simplicity it brings to data analysis. Whether you're a beginner looking to dip your toes into the data world or a seasoned professional aiming to brush up on your skills, this guide promises to equip you with the tools you need to start leveraging data like never before.
Why Pandas?
Before diving into the how, let's talk about the why. Pandas is an open-source, BSD-licensed library providing high-performance, easy-to-use data structures and data analysis tools for the Python programming language. It's the Swiss Army knife for data scientists, allowing for the cleaning, transforming, and analyzing of data all within one powerful and intuitive framework. From merging and reshaping data sets to time-series analysis, Pandas turns complex operations with data into one-liners. So, why Pandas? Because it makes data analysis fast, efficient, and accessible to everyone.
Getting Started with Pandas
First things first, you need to have Pandas installed. If you haven't done so yet, open up your terminal or command prompt and type:
pip install pandas
With Pandas installed, let's dive into some basics. The heart of Pandas is its DataFrame—a 2-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns). Think of it like an Excel spreadsheet that's turbocharged for data analysis.
Creating Your First DataFrame
Let's create a simple DataFrame from a Python dictionary:
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 34, 29, 32],
'City': ['New York', 'Paris', 'Berlin', 'London']}
df = pd.DataFrame(data)
print(df)
This snippet creates a DataFrame with names, ages, and cities. The print(df)
command will display your DataFrame in a neat, table-like format.
Basic Data Manipulation
Now that you have a DataFrame, what can you do with it? Pandas offers a plethora of functions for manipulating data. Here are a few essentials:
Accessing Data
You can access specific columns using their names:
print(df['Name'])
Or rows using their index:
print(df.iloc[0]) # Accesses the first row
Filtering Data
Want to see only the records of people older than 30? No problem:
print(df[df['Age'] > 30])
Adding and Removing Columns
Adding a new column is as simple as assigning it to the DataFrame:
df['Profession'] = ['Doctor', 'Artist', 'Engineer', 'Writer']
print(df)
And removing one is just as easy:
df.drop('Age', axis=1, inplace=True)
print(df)
Analysis and Visualization
Pandas isn't just for manipulating data; it's also incredibly powerful for analysis. With built-in functions for descriptive statistics, you can easily get a high-level overview of your data:
print(df.describe())
Moreover, Pandas seamlessly integrates with Matplotlib, a plotting library for Python, allowing for easy data visualization. Here's how to create a simple plot:
import matplotlib.pyplot as plt
df['Age'].plot(kind='hist')
plt.show()
This code snippet will generate a histogram of ages from your DataFrame, providing a visual insight into the distribution of ages within your dataset.
Conclusion
Congratulations! In just 10 minutes, you've unlocked the basics of using Pandas for data analysis. You've learned how to create DataFrames, manipulate data, and even visualize it. While this guide is just the tip of the iceberg, it's a solid foundation that you can build upon. The world of data analysis is vast and exciting, filled with opportunities to uncover insights and make data-driven decisions.
Remember, the best way to learn is by doing. So, I encourage you to start experimenting with your datasets, explore the comprehensive Pandas documentation, and join the vibrant community of data enthusiasts. Unlock the power of data with Pandas, and let your data analysis journey begin!
Recent Posts
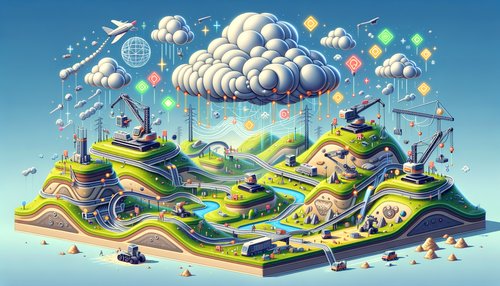
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
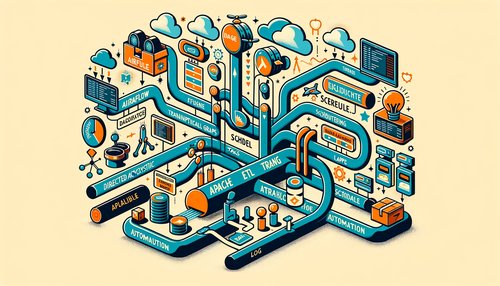
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow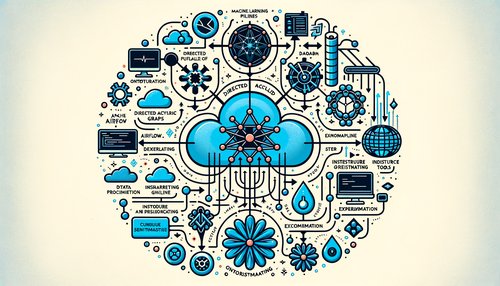
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow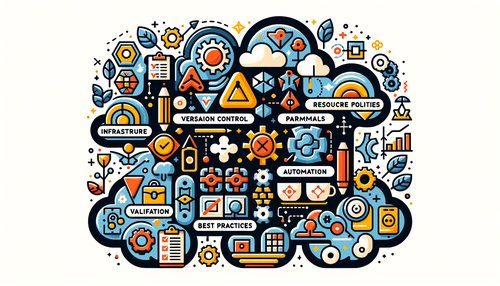
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
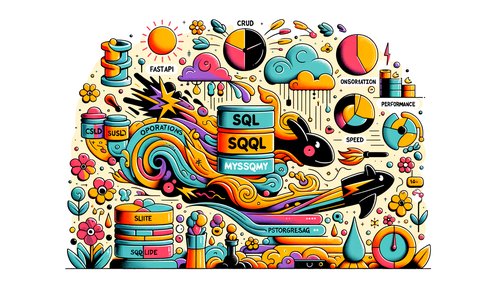