Unlock the Power of Data: Mastering the Art of 'Split-Apply-Combine' with Pandas User Guide
Welcome to the journey of mastering one of the most efficient data manipulation techniques in Python's Pandas library: the 'Split-Apply-Combine' strategy. This powerful approach is essential for anyone looking to delve deep into data analysis, offering a structured method to dissect complex data, perform specific operations, and reassemble the results. Whether you're a data science enthusiast, a budding analyst, or a seasoned professional, understanding this technique will significantly enhance your data manipulation and analysis skills. In this guide, we'll explore the nuances of 'Split-Apply-Combine', complete with practical examples and tips to help you become proficient in handling your data with Pandas.
Understanding the 'Split-Apply-Combine' Strategy
Before diving into the technicalities, let's demystify the 'Split-Apply-Combine' strategy. Coined by Hadley Wickham in his paper, this approach is a three-step process used to analyze data:
- Split: The dataset is divided into smaller pieces based on certain criteria.
- Apply: A function is applied to each piece independently.
- Combine: The results are merged back into a single dataset.
This methodology is particularly useful for grouped operations and aggregations, allowing for complex data transformations and analysis with relative ease.
Implementing 'Split-Apply-Combine' in Pandas
Pandas, a cornerstone library for data analysis in Python, implements the 'Split-Apply-Combine' strategy through its groupby()
method. Let's break down each step with examples to illustrate how it works in practice.
Splitting the Data
To split your data, you'll use the groupby()
function, specifying the column(s) you want to group by. For instance:
import pandas as pd
# Sample data
df = pd.DataFrame({
'Category': ['A', 'B', 'A', 'B'],
'Values': [10, 20, 30, 40]
})
# Splitting the data by 'Category'
grouped = df.groupby('Category')
This will create a GroupBy
object which can be thought of as a collection of groups waiting to have a function applied to them.
Applying a Function
Next, you can apply a function to each group. This can be anything from aggregation functions like sum()
or mean()
to more complex custom functions. Continuing from the previous example:
# Applying the sum function to each group
summed = grouped.sum()
print(summed)
This will output the sum of 'Values' for each 'Category', effectively applying the desired operation to each split group.
Combining the Results
The beauty of Pandas is that it automatically combines the results of the applied function into a new DataFrame. The index of this DataFrame corresponds to the unique values of the column you grouped by, providing a neatly organized result of your applied function.
Advanced 'Split-Apply-Combine' Operations
While aggregation is a common use case, the 'Split-Apply-Combine' strategy can also be used for more advanced operations, such as:
- Filtering groups based on certain criteria.
- Transforming data within each group.
- Applying custom functions to each group.
For example, to filter groups with a sum of 'Values' greater than 30:
def filter_func(x):
return x['Values'].sum() > 30
filtered = grouped.filter(filter_func)
print(filtered)
This will return a DataFrame containing only the rows belonging to groups that meet the specified condition.
Practical Tips and Insights
When working with 'Split-Apply-Combine', keep the following in mind:
- Ensure your data is properly cleaned and structured before splitting. This will save you from unexpected results or errors.
- Utilize the power of custom functions to perform complex operations on your groups. This extends the capability of your data analysis significantly.
- Explore the various built-in aggregation functions Pandas offers, such as
mean()
,std()
, andcount()
, to streamline your analysis.
Conclusion
The 'Split-Apply-Combine' strategy is a cornerstone technique in data analysis, offering a flexible approach to handling and analyzing large datasets. By mastering this method with Pandas, you unlock a new level of data manipulation capabilities, enabling you to extract meaningful insights from your data efficiently. Remember, the key to proficiency is practice, so don't hesitate to experiment with different datasets and operations to hone your skills. Happy analyzing!
As a final thought, consider exploring the extensive documentation and user guide provided by Pandas to further deepen your understanding and capabilities with the library. The more you explore, the more proficient you'll become in navigating the vast landscape of data analysis.
Recent Posts
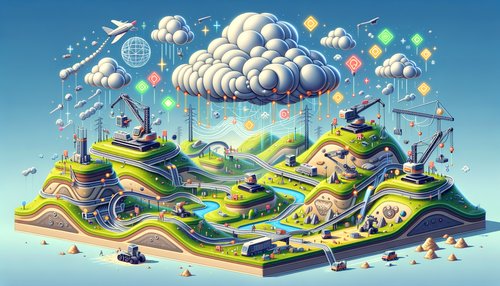
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
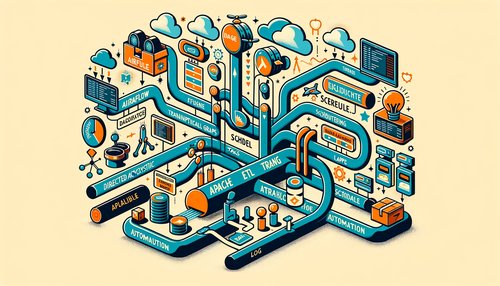
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow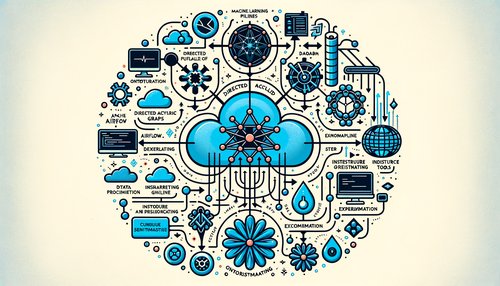
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow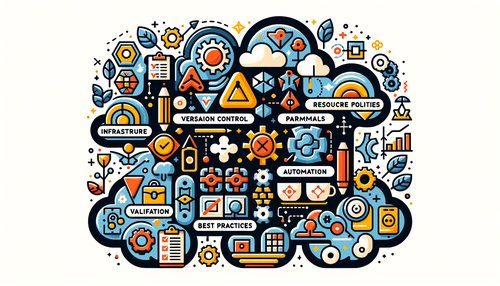
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
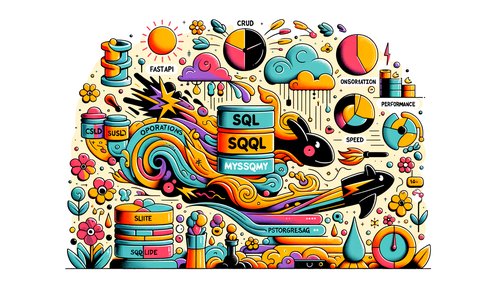