Unlock the Power of Django Receivers: How to Create Custom Signals for Your Application
Do you want to create complex but powerful applications quickly and easily? Django offers a powerful way to do just that. Django receivers allow you to create custom signals that can be used to trigger events and automate actions. In this blog post, we will explore how to use Django receivers to create custom signals for your application.
What are Django Receivers?
Django receivers are functions that are triggered when a signal is sent. You can think of them as event handlers, which are triggered when a certain event occurs. For example, when a user registers on your website, you might want to send an email to welcome them. You can create a Django receiver that will be triggered when the user registers, and send the email automatically.
How to Create a Django Receiver
Creating a Django receiver is a simple process. First, you need to create a signal that will be sent when the event occurs. The signal will contain the data that you want to send. Here is an example of a signal that will be sent when a user registers:
from django.dispatch import Signal
user_registered = Signal(providing_args=['user'])
Next, you need to create a receiver that will be triggered when the signal is sent. The receiver will contain the code that will be executed when the signal is sent. Here is an example of a receiver that will send a welcome email when a user registers:
from django.dispatch import receiver
from django.contrib.auth.models import User
@receiver(user_registered)
def send_welcome_email(sender, **kwargs):
user = kwargs.get('user')
if isinstance(user, User):
# Send welcome email
pass
Finally, you need to connect the signal to the receiver. This is done using the connect
method. Here is an example of how to connect the signal and receiver:
user_registered.connect(send_welcome_email)
Conclusion
Django receivers are a powerful way to create custom signals that can be used to trigger events and automate actions. By creating a signal and connecting it to a receiver, you can easily create powerful applications that can be used to automate tasks and make your life easier.
Recent Posts
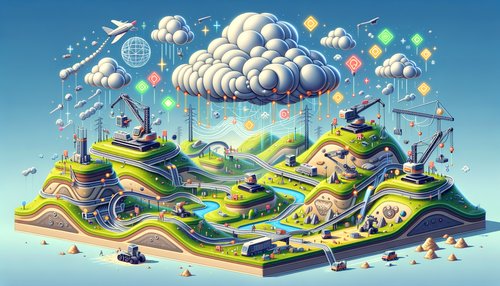
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
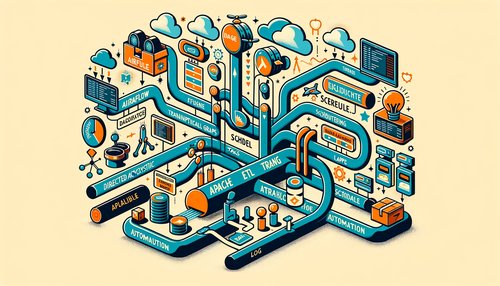
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow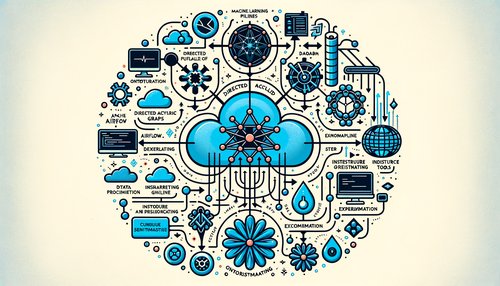
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow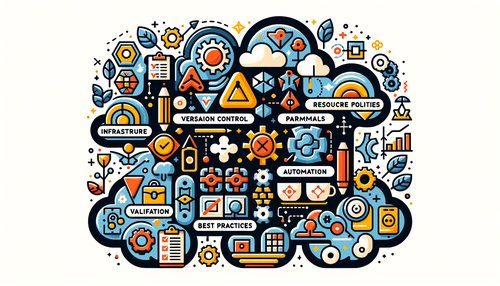
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
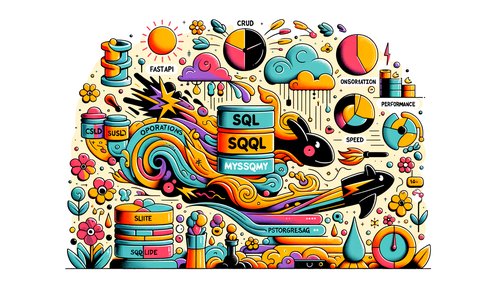