Unlock the Power of Django REST API: Create Robust Web Applications with Ease
Django is a powerful web framework for building robust web applications. One of the best features of Django is its Django REST API, which allows developers to quickly and easily create robust web applications. With the REST API, developers can easily create, configure, and deploy web applications that are secure, reliable, and scalable.
In this blog post, we'll discuss how to use the Django REST API to create robust web applications with ease. We'll also look at some code snippets to help you get started.
Create a REST API
The first step in creating a Django REST API is to create a new application. To do this, open up a terminal window and run the following command:
$ django-admin startproject myapp
This will create a new Django project called myapp
. Next, you'll need to create a new app within the project. To do this, run the following command from the project directory:
$ python manage.py startapp myapp_api
This will create a new app called myapp_api
.
Configure the REST API
Once the app has been created, you'll need to configure the REST API. To do this, open up the settings.py
file and add the following line to the INSTALLED_APPS
list:
INSTALLED_APPS = [
...
'rest_framework',
...
]
This will enable the Django REST framework.
Create a Serializer
Once the REST framework is enabled, you'll need to create a serializer. A serializer is a class that converts a model into a JSON or XML format. To create a serializer, create a new file called serializers.py
in the myapp_api
directory. Then, add the following code to the file:
from rest_framework import serializers
from myapp.models import MyModel
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = ('field_1', 'field_2', 'field_3')
This will create a serializer for the MyModel
model.
Create a View
Now that the serializer has been created, the next step is to create a view. A view is a class that handles the request and response for a particular URL. To create a view, create a new file called views.py
in the myapp_api
directory. Then, add the following code to the file:
from rest_framework import viewsets
from myapp.models import MyModel
from .serializers import MyModelSerializer
class MyModelViewSet(viewsets.ModelViewSet):
queryset = MyModel.objects.all()
serializer_class = MyModelSerializer
This will create a view for the MyModel
model.
Configure the URLs
Finally, you'll need to configure the URLs for the API. To do this, open up the urls.py
file and add the following code:
from django.conf.urls import include, url
from rest_framework import routers
from myapp_api.views import MyModelViewSet
router = routers.DefaultRouter()
router.register(r'mymodel', MyModelViewSet)
urlpatterns = [
url(r'^api/', include(router.urls)),
]
This will enable the API and configure the URLs.
Conclusion
In this blog post, we've discussed how to use the Django REST API to create robust web applications with ease. We've looked at how to create a new application, configure the REST API, create a serializer, create a view, and configure the URLs. With this knowledge, you should be able to quickly and easily create robust web applications using the Django REST API.
Recent Posts
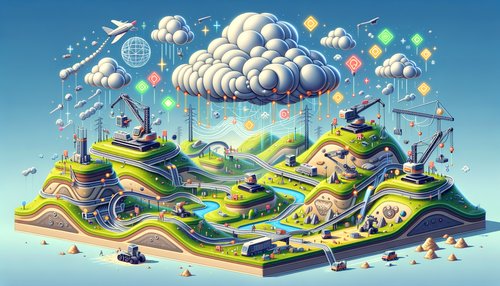
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
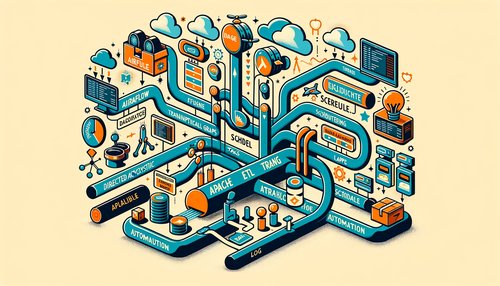
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow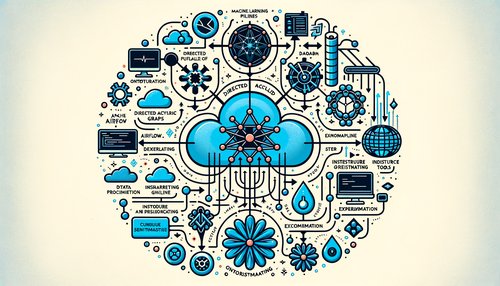
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow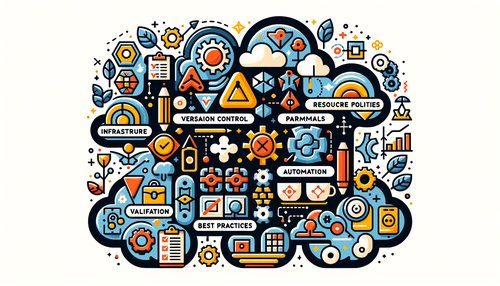
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
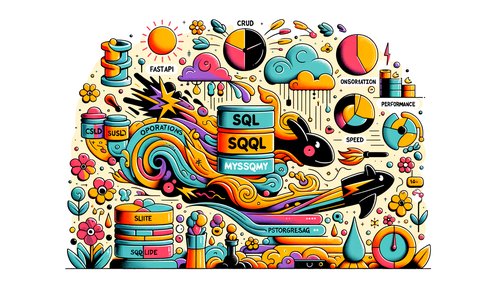