Unlock the Power of Django Signals: A Guide to Utilizing Signals for Improved Web Development
Django signals are a powerful feature that can help you improve the development of your web applications. They provide a way to execute code when certain events occur, and can be used to create custom events for your application. In this guide, we’ll discuss what signals are, how to use them, and the potential benefits of utilizing signals for improved web development.
What are Django Signals?
Django signals are a way to send notifications when certain actions occur in your application. They provide a way to execute code when certain events occur, allowing you to create custom events and provide a way to respond to them. For example, you could create a signal that is triggered when a user registers on your site, and execute code to send a welcome email.
How to Use Django Signals
Using Django signals is fairly straightforward. To create a signal, you simply define a function that takes the sender and the signal instance as arguments. This function will be called when the signal is triggered.
For example, to create a signal that is triggered when a user registers on your site, you would create a function like this:
from django.dispatch import Signal
user_registered = Signal(providing_args=['user'])
def welcome_email(sender, **kwargs):
user = kwargs.get('user')
# Send welcome email
Once you have created the signal, you can connect it to a receiver that will be called when the signal is triggered. For example, to connect your welcome_email
function to the user_registered
signal, you would use the @receiver
decorator like this:
from django.dispatch import receiver
@receiver(user_registered)
def welcome_email(sender, **kwargs):
user = kwargs.get('user')
# Send welcome email
Finally, you can trigger the signal wherever you need to. For example, if you wanted to trigger the user_registered
signal when a user registers on your site, you would do something like this:
from django.dispatch import Signal
user_registered = Signal(providing_args=['user'])
def register(request):
# Register user
user_registered.send(sender=None, user=user)
Benefits of Using Signals
Using Django signals can provide a number of benefits for your web development. Firstly, they provide a way to execute code when certain events occur, allowing you to create custom events and respond to them. This can be particularly useful for responding to user actions, such as sending a welcome email when a user registers on your site.
Secondly, signals allow you to decouple your code, making it easier to maintain and extend. This is because the code that triggers the signal is separate from the code that responds to it. This makes it easier to modify and extend your code, as you can simply add or remove receivers without having to modify the code that triggers the signal.
Finally, signals provide a way to create custom events that can be triggered by various actions in your application. This can be useful for creating notifications or integrating with third-party services. For example, you could create a signal that is triggered when a user registers, and use it to send a notification to a chat service.
Conclusion
Django signals are a powerful feature that can help you improve the development of your web applications. They provide a way to execute code when certain events occur, allowing you to create custom events and respond to them. They also allow you to decouple your code and create custom events that can be triggered by various actions in your application. With this guide, you should now have a better understanding of how to use signals for improved web development.
Recent Posts
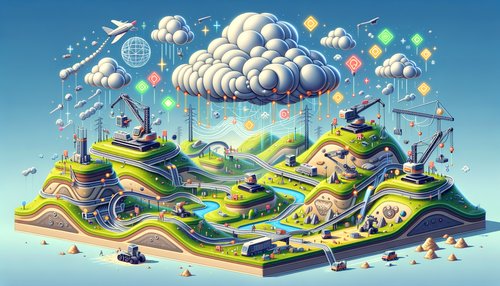
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
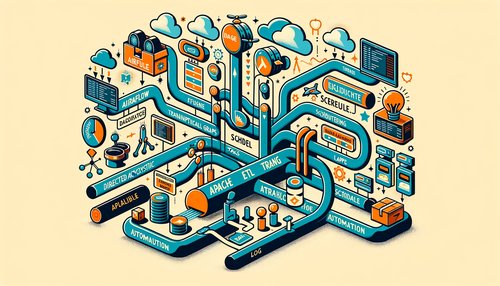
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow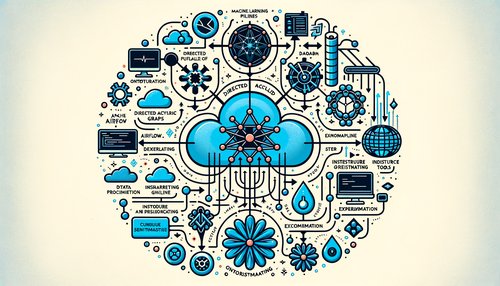
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow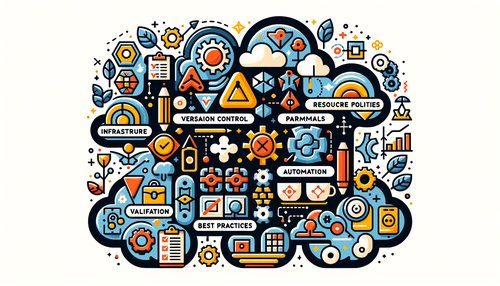
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
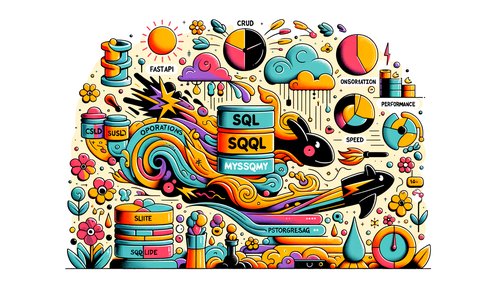