Unlock the Power of React Hooks: A Beginner's Guide to Building Better Apps
React Hooks are an incredibly powerful tool for creating powerful and efficient React applications. In this guide, we will explore the basics of React Hooks, learn how to use them in your own apps, and discuss some of the best practices associated with them. With the help of this guide, you'll be able to unlock the power of React Hooks and create better apps with less code.
What are React Hooks?
React Hooks are a new feature introduced in React 16.8 that allow developers to use state and other React features without writing a class. They are a way to add React features such as state and lifecycle methods to functional components. Hooks are a powerful tool for creating composable and reusable logic, and they are the foundation for many advanced React features, such as the Context API.
How to Use React Hooks
Using React Hooks is simple and straightforward. To use a Hook, you simply call the Hook within a functional component. This will give you access to the state and other React features within the component.
For example, to use the useState Hook, you can add the following code to your component:
const [count, setCount] = useState(0);
This will create a piece of state in the component called count
and a function called setCount
that can be used to update the state.
Best Practices for Using React Hooks
Using React Hooks correctly and efficiently is key to creating powerful and efficient applications. Here are some best practices for using Hooks:
-
Only call Hooks from React functions: Hooks should only be called from React functions, not regular JavaScript functions.
-
Only call Hooks at the top level: Hooks should only be called at the top level of the component, not inside loops, nested functions, or conditionals.
-
Use the useEffect Hook: The useEffect Hook is a great way to handle side effects, such as data fetching or setting up subscriptions.
-
Write custom Hooks: Writing your own custom Hooks can help you create reusable logic and reduce code duplication.
By following these best practices, you'll be able to unlock the power of React Hooks and create better apps with less code.
Conclusion
React Hooks are a powerful tool for creating powerful and efficient React applications. With the help of this guide, you'll be able to unlock the power of React Hooks and create better apps with less code. By following the best practices outlined above, you'll be able to take full advantage of React Hooks and create powerful and efficient apps.
Recent Posts
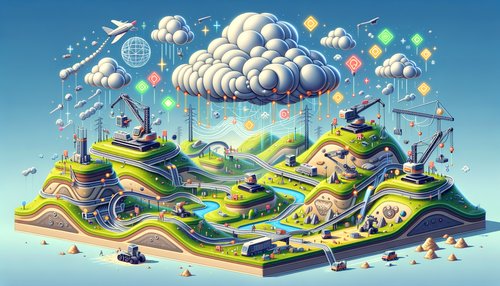
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
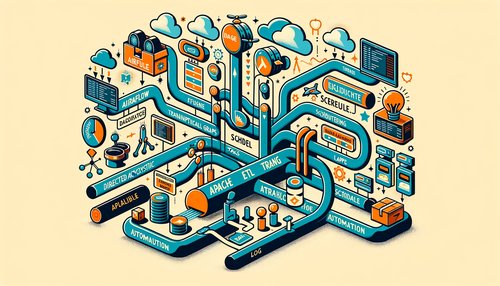
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow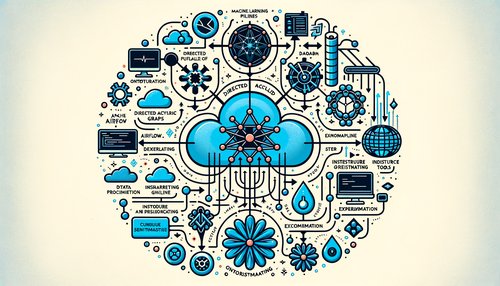
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow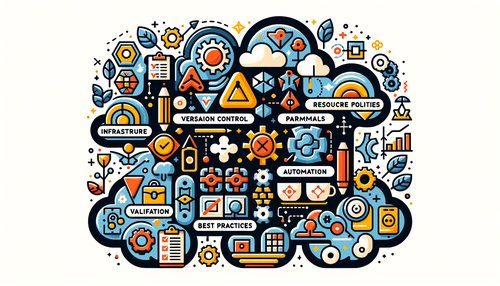
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
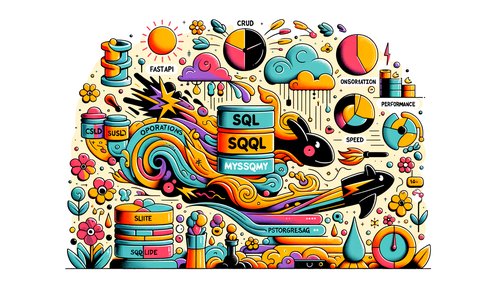