Unlock the Power of React Hooks to Supercharge Your App Development!
React Hooks are a powerful tool for React developers, allowing them to write cleaner, more reusable code that can be easily shared and maintained. React Hooks provide developers with the ability to use React components without the need to use classes or complex patterns. With React Hooks, developers can create powerful, dynamic applications without sacrificing performance or scalability.
React Hooks allow developers to write custom logic and create components that can be reused across multiple applications. This allows developers to create components that are both modular and extensible. Additionally, React Hooks allow developers to easily share code between projects, making it easier to maintain and develop applications.
React Hooks also allow developers to write code that is more concise and readable. The use of React Hooks makes it easy to create components that can be quickly understood and maintained. Additionally, React Hooks provide developers with the ability to write code that is more maintainable and testable.
Benefits of React Hooks
-
Reusable Components: React Hooks allow developers to create components that can be reused across multiple applications. This allows developers to create components that are both modular and extensible.
-
Concise Code: React Hooks make it easy to write code that is concise and readable. The use of React Hooks makes it easy to create components that can be quickly understood and maintained.
-
Testable Code: React Hooks also allow developers to write code that is more maintainable and testable. This makes it easier to quickly identify and fix bugs.
-
Performance: With React Hooks, developers can create powerful, dynamic applications without sacrificing performance or scalability.
Examples of React Hooks
One of the most popular React Hooks is the useState
hook. The useState
hook allows developers to create components that manage their own state. The following code snippet demonstrates how to use the useState
hook to create a simple counter component:
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
const decrement = () => {
setCount(count - 1);
};
return (
<div>
<p>{count}</p>
<button onClick={increment}>+</button>
<button onClick={decrement}>-</button>
</div>
);
The useEffect
hook is another popular React Hook. This hook allows developers to create components that can respond to changes in data or state. The following code snippet demonstrates how to use the useEffect
hook to create an effect that will be triggered when the count
state changes:
useEffect(() => {
console.log('count changed', count);
}, [count]);
Conclusion
React Hooks are a powerful tool for React developers, allowing them to write cleaner, more reusable code that can be easily shared and maintained. React Hooks provide developers with the ability to use React components without the need to use classes or complex patterns. With React Hooks, developers can create powerful, dynamic applications without sacrificing performance or scalability.
Recent Posts
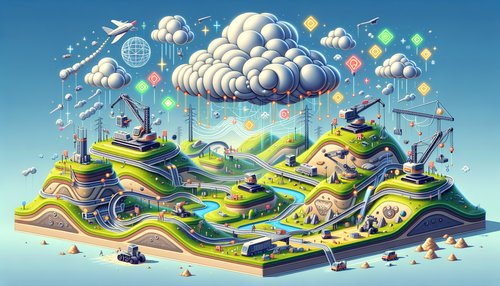
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
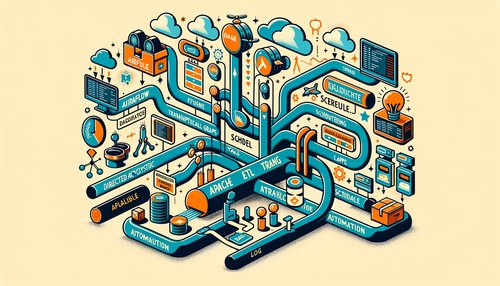
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow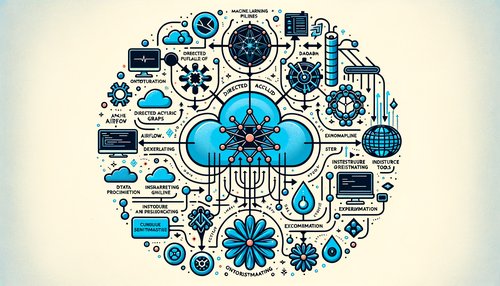
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow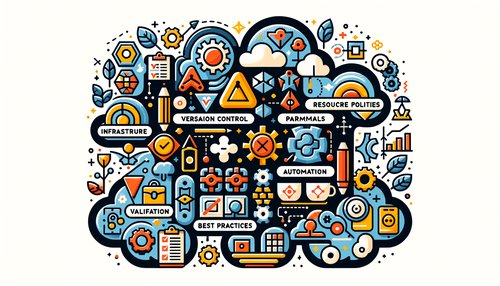
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
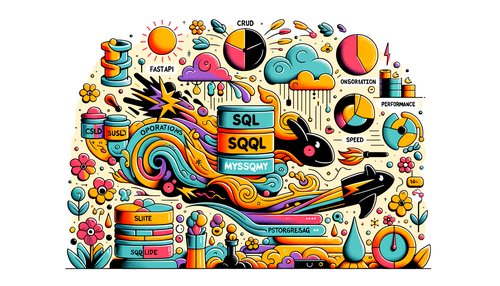