Unlocking Advanced Features: A Deep Dive into FastAPI with Extra Models User Guide
Welcome to an exciting exploration of FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. FastAPI is renowned for its speed, ease of use, and robustness. But beyond its well-known features, lies a treasure trove of advanced functionalities waiting to be unlocked. This guide aims to delve deep into these less explored territories, focusing particularly on the utilization of extra models. Whether you're looking to enhance your existing FastAPI projects or planning to start a new one, this guide promises to equip you with the knowledge to leverage FastAPI's full potential.
Understanding Extra Models in FastAPI
Before diving into the intricacies, it's crucial to understand what we mean by "extra models" in the context of FastAPI. Extra models typically refer to the use of Pydantic models that aren't directly linked to request or response models but are essential for complex data processing, validation, and more. These models can significantly streamline your code, making it more readable, maintainable, and scalable.
Why Use Extra Models?
Extra models come into play for several reasons:
- Validation Layer: They can serve as an additional validation layer for your data, ensuring that only valid data is processed by your application.
- Data Transformation: They are instrumental in data transformation, making it easy to convert user input into the desired format before processing.
- Code Reusability: By defining complex data structures as models, you can easily reuse them across your application, reducing redundancy.
Implementing Extra Models in Your FastAPI Project
Implementing extra models in FastAPI is straightforward, thanks to Pydantic. Here's a step-by-step guide to get you started:
Defining Your Extra Models
First, define your extra models by extending the BaseModel
class from Pydantic.
from pydantic import BaseModel
class ComplexDataType(BaseModel):
attribute1: str
attribute2: int
# Add more attributes as needed
Utilizing Extra Models in Endpoints
Once your extra models are defined, you can use them in your FastAPI endpoints. This can be done by including them as function parameters or within request/response models.
from fastapi import FastAPI
from models import ComplexDataType # Assuming your extra models are in a module named models
app = FastAPI()
@app.post("/process-data/")
async def process_data(complex_data: ComplexDataType):
# Your logic here
return {"message": "Data processed successfully"}
Best Practices for Working with Extra Models
While extra models can significantly enhance your application, following best practices is crucial for maximizing their benefits:
- Keep Models Lean: Avoid overloading your models with unnecessary attributes. Stick to what's essential for the task at hand.
- Use Sub-models: For complex data structures, break down your models into smaller sub-models. This approach enhances readability and maintainability.
- Validation Logic: Leverage Pydantic's validation features to enforce data integrity and constraints within your models.
Conclusion
FastAPI's support for extra models opens up a world of possibilities for developers, enabling more robust data validation, transformation, and code reusability. By understanding and implementing extra models in your FastAPI projects, you can unlock advanced features that lead to cleaner, more efficient, and scalable applications. Remember, the key to effectively using extra models lies in defining clear, concise models and adhering to best practices. So, take this knowledge, experiment with extra models in your next FastAPI project, and watch your applications transform!
Embrace the power of extra models and let them guide you towards building more sophisticated, high-quality APIs with FastAPI.
Recent Posts
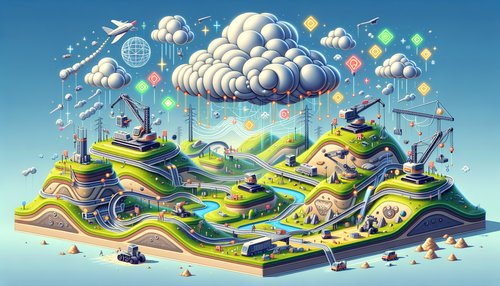
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
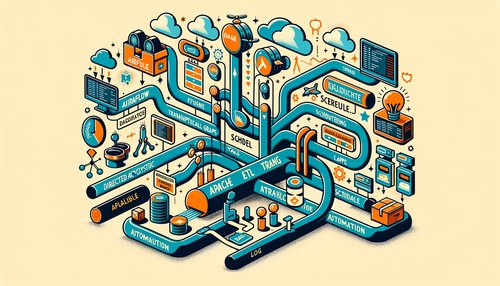
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow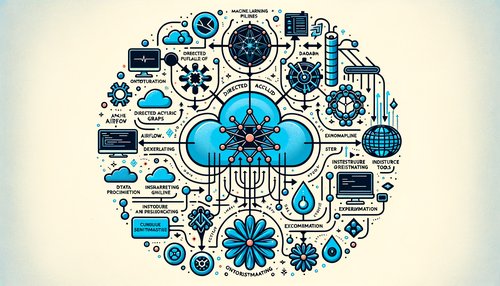
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow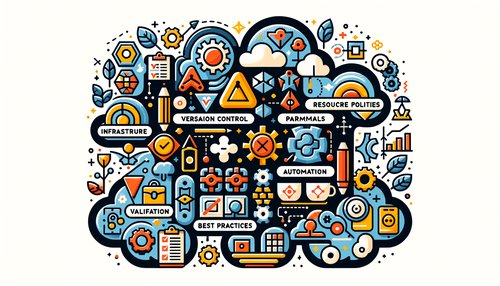
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
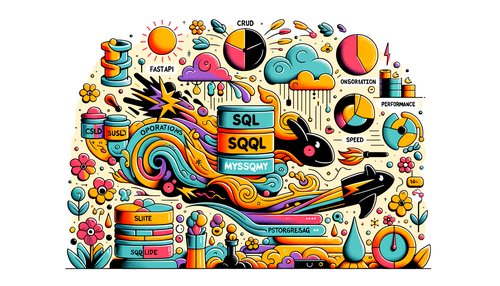