Unlocking Asynchronous Awesomeness: A Deep Dive into FastAPI's Background Tasks for User Guide Wizards
Welcome to a journey into the heart of asynchronous programming with FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. Today, we're diving deep into an exceptionally powerful feature of FastAPI: Background Tasks. This guide is crafted for those who are ready to enhance their web applications with non-blocking operations, ensuring a seamless user experience while performing heavy-lift operations in the background.
What are Background Tasks?
Before we embark on this adventure, let's clarify what background tasks are. In the context of web development, background tasks are operations that run asynchronously, separate from the main execution thread of your application. This means your application can continue to receive and respond to user requests while performing tasks like sending emails, processing files, or calling external APIs in the background. FastAPI makes handling these tasks straightforward and efficient, leveraging the power of Starlette for the web parts and Pydantic for the data parts.
Setting the Stage: FastAPI Configuration
First things first, setting up a FastAPI project to utilize background tasks is a breeze. Ensure you have FastAPI and Uvicorn (an ASGI server) installed. Your initial setup should look something like this:
from fastapi import FastAPI, BackgroundTasks
app = FastAPI()
@app.post("/send-notification/")
async def send_notification(email: str, background_tasks: BackgroundTasks):
background_tasks.add_task(send_email, email)
return {"message": "Notification is being sent in the background"}
# Define the asynchronous send_email function here
This snippet illustrates how to define an endpoint that utilizes a background task for sending an email. Notice how the send_notification
function accepts a BackgroundTasks
object as a parameter. This object is key to adding tasks that should run in the background.
Deep Dive: Working with Background Tasks
Adding a task to run in the background is as simple as calling the add_task
method on the BackgroundTasks
object, passing in the function you wish to run and any arguments it needs. But there's more to it. Let's explore some best practices and tips for maximizing the potential of background tasks in FastAPI.
Tip #1: Keep It Non-Blocking
When designing functions to run as background tasks, ensure they are non-blocking. Utilize asynchronous database calls, file operations, and network requests wherever possible. This approach maximizes the efficiency of your application, keeping it responsive and fast.
Tip #2: Error Handling
Robust error handling in background tasks is crucial. Since these tasks run independently of the main application flow, unhandled errors can crash your background operations without affecting the main application. Implement try-except blocks in your background functions to catch and log exceptions appropriately.
Tip #3: Task Monitoring and Management
While FastAPI's background tasks are designed to be fire-and-forget, certain applications might require monitoring or managing these tasks post-launch. For more complex needs, consider integrating a task queue like Celery with FastAPI. This setup offers greater control, including task retries, failures handling, and result querying.
Practical Example: File Processing
Imagine an application that allows users to upload files for processing. Such operations can be time-consuming, and running them synchronously could lead to a poor user experience. Here's how you might handle this with a background task:
from fastapi import FastAPI, BackgroundTasks, File, UploadFile
from some_file_processing_module import process_file
app = FastAPI()
@app.post("/uploadfile/")
async def upload_file(background_tasks: BackgroundTasks, file: UploadFile = File(...)):
background_tasks.add_task(process_file, file.file)
return {"filename": file.filename, "message": "File is being processed in the background"}
This code snippet demonstrates receiving a file from a user and adding a file processing operation as a background task, ensuring the user receives immediate feedback while the heavy lifting happens behind the scenes.
Conclusion: Embracing Asynchronous Operations
Integrating background tasks into your FastAPI applications unlocks a new level of performance and user experience. By offloading time-consuming operations to the background, your applications remain snappy and responsive, regardless of the workload. Remember to design your background functions to be non-blocking, handle errors gracefully, and consider more sophisticated task management solutions for complex scenarios.
As you venture into the realm of asynchronous programming with FastAPI, let the principles and examples outlined in this guide illuminate your path. Embrace the asynchronous awesomeness, and may your applications thrive in the fast lane of efficiency and scalability.
Happy coding!
Recent Posts
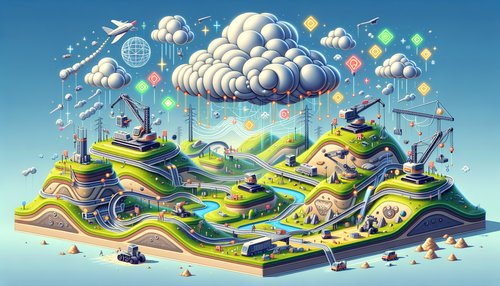
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
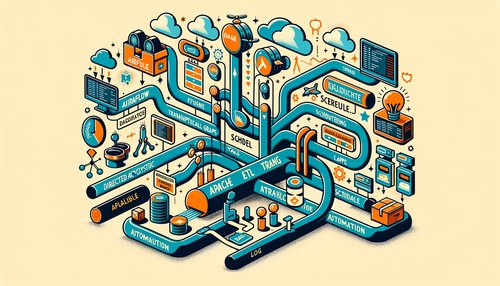
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow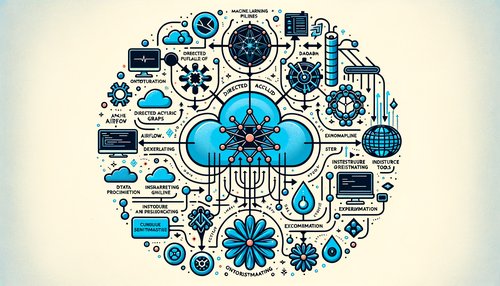
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow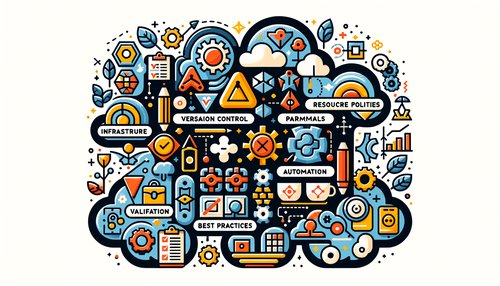
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
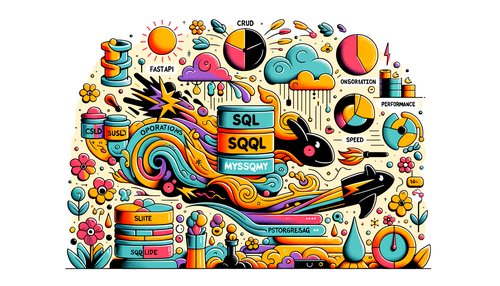