Unlocking Efficiency: A Deep Dive into Sparse Data Structures with the Pandas User Guide
In the ever-evolving world of data analysis, efficiency is key. Whether you're a data scientist, analyst, or enthusiast, you're likely familiar with the challenges of handling large datasets, especially when they are filled with missing or insignificant values. This is where sparse data structures come into play, offering a lifeline for optimizing memory and improving computational efficiency. In this blog post, we'll embark on a deep dive into sparse data structures, guided by insights from the Pandas User Guide. From understanding the basics to implementing advanced techniques, get ready to unlock the full potential of your data with pandas.
Understanding Sparse Data Structures
Sparse data structures are designed to efficiently store and manipulate data that contains a significant number of default or missing values. Unlike their dense counterparts, which allocate memory for every element, sparse structures only store non-default values, drastically reducing memory usage. This concept is particularly useful in pandas, a powerful Python library for data manipulation and analysis, which supports sparse data structures for Series and DataFrames.
Why Use Sparse Structures?
- Memory Efficiency: By only storing non-default values, sparse structures can lead to substantial memory savings, especially in datasets where the majority of values are default.
- Computational Speed: Operations on sparse structures can be faster, as they skip over the default values. This can speed up calculations and analyses on large datasets.
- Storage: Sparse structures can also reduce storage requirements, making it easier to handle and share large datasets.
Implementing Sparse Data Structures in Pandas
Pandas offers comprehensive support for sparse data through its SparseArray and SparseDataFrame objects. Let's explore how to implement and utilize these structures effectively.
Creating Sparse Arrays
To create a SparseArray in pandas, you can use the SparseArray
constructor. This is particularly useful when you have a sequence of data and you know that a significant portion of it consists of a fill value (e.g., 0, NaN).
import pandas as pd
import numpy as np
# Creating a SparseArray
data = np.random.choice([0, 1, 2], size=1000, p=[0.8, 0.1, 0.1])
sparse_array = pd.arrays.SparseArray(data)
print(sparse_array)
Working with Sparse DataFrames
While SparseArray is great for single dimensions, SparseDataFrame extends this efficiency to two-dimensional data. Starting from pandas 1.0.0, the recommended approach is to use a standard DataFrame with sparse values.
# Creating a DataFrame with sparse data
df = pd.DataFrame(np.random.choice([0, 1], size=(100, 4), p=[0.95, 0.05]))
sparse_df = df.astype(pd.SparseDtype(int, fill_value=0))
print(sparse_df.dtypes)
Optimizing Performance
While sparse data structures offer significant benefits, there are best practices to follow for optimizing performance:
- Choose the Right Fill Value: The efficiency of sparse structures depends on the choice of fill value. Ensure that the fill value is the most common value in your dataset.
- Consider the Density: If your data is not very sparse (i.e., the proportion of non-default values is high), sparse structures may not offer benefits and could even lead to overhead. Assess the sparsity of your data before converting.
- Use Sparse Data Where Appropriate: Sparse structures are not a one-size-fits-all solution. Use them judiciously, focusing on datasets and columns where they provide clear advantages.
Conclusion
Sparse data structures represent a powerful tool in the data analyst's toolkit, offering significant memory and computational efficiency gains for the right datasets. By understanding and implementing these structures within pandas, you can handle large, sparse datasets more effectively, freeing up resources for deeper analysis and insights. As we've explored, the key to unlocking these benefits lies in knowing when and how to use sparse structures. With the guidance provided in this post, you're well-equipped to start optimizing your data analysis projects. So why wait? Dive into your data with pandas and start reaping the benefits of sparse data structures today!
Whether you're a seasoned data professional or just starting out, embracing sparse data structures can be a game-changer for your projects. We encourage you to experiment with the techniques outlined in this post and discover the efficiency gains for yourself. Happy analyzing!
Recent Posts
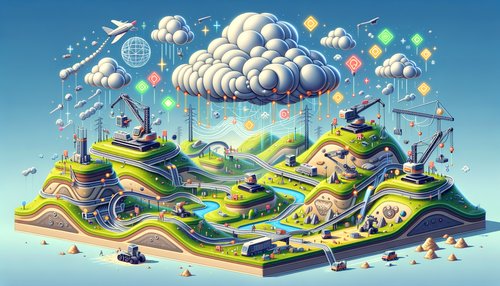
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
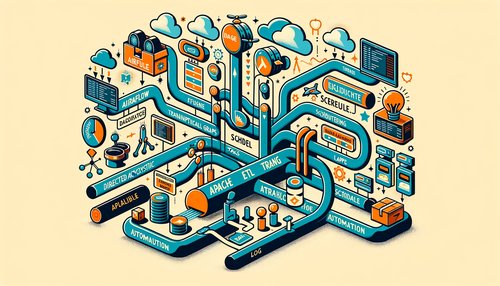
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow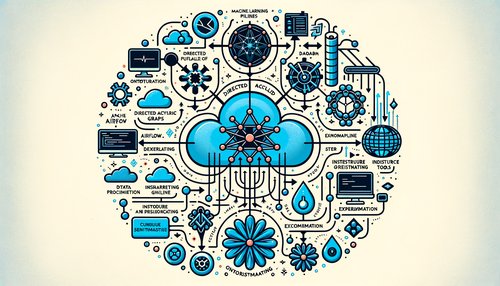
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow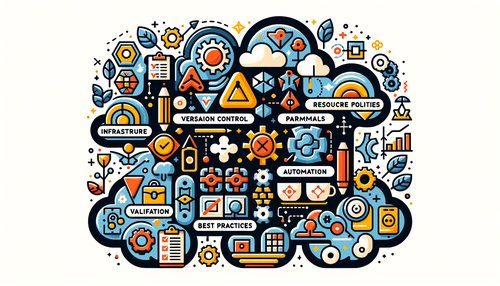
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
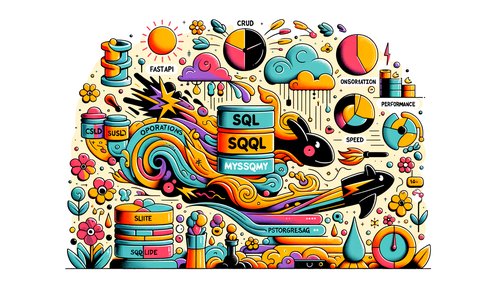