Unlocking Power: Mastering Terraform Expressions and Function Calls for Optimal Infrastructure Automation
As organizations migrate to the cloud and seek to automate their infrastructure, Terraform has emerged as a pivotal tool in infrastructure as code (IaC). Key to its versatility and power are its expressions and function calls. In this post, we’ll explore how you can unlock the power of Terraform expressions and function calls to optimize your infrastructure automation.
Introduction to Terraform Expressions
Terraform expressions are a fundamental part of writing configuration files. They allow you to calculate data, define conditions, and perform iterations. By mastering expressions, you can make your infrastructure configurations more dynamic and reusable. We'll start by looking at the basic syntax and then dive into more advanced usage.
Basic Syntax
In Terraform, expressions can be as simple as a static value or as complex as a combination of functions and variables. Here’s an example of a basic expression:
resource "aws_instance" "example" {
ami = "${var.ami_id}"
instance_type = "t2.micro"
}
In this example, the expression ${var.ami_id}
retrieves the value of the variable ami_id
. Understanding this basic syntax is essential as it forms the foundation for more complex expressions.
Advanced Expressions in Terraform
Terraform’s expressions become more powerful with their ability to incorporate conditionals, loops, and interpolations. These features reduce redundancy and enhance the flexibility of your configurations.
Conditionals
Conditionals in Terraform are similar to those in programming languages, enabling you to make decisions within your configuration. Here’s an example:
resource "aws_instance" "example" {
ami = var.environment == "production" ? var.prod_ami_id : var.dev_ami_id
}
In this instance, the AMI (Amazon Machine Image) ID is chosen based on the environment. If the environment is production, it uses var.prod_ami_id
; otherwise, it uses var.dev_ami_id
.
Loops
Loops are extremely useful when you need to create multiple resources of the same type with slight variations. Here’s an example using count
:
resource "aws_instance" "example" {
count = 3
ami = var.ami_id
instance_type = "t2.micro"
}
This loop will create three instances with the defined AMI and instance type.
Terraform Function Calls
Functions in Terraform provide powerful capabilities for data manipulation. They enable various operations such as string manipulations, numeric calculations, and type conversions. Understanding and utilizing these functions can greatly enhance your Terraform scripts.
Commonly Used Functions
Here are some commonly used Terraform functions:
- length(): Computes the length of a list or string.
- concat(): Concatenates two or more lists.
- format(): Formats a string with variables.
- lookup(): Retrieves a value from a map.
Let's see some of these functions in action:
locals {
list_example = ["one", "two", "three"]
map_example = {
key1 = "value1"
key2 = "value2"
}
}
output "list_length" {
value = length(local.list_example)
}
output "combined_list" {
value = concat(local.list_example, ["four"])
}
output "formatted_string" {
value = format("The length of the list is %d", length(local.list_example))
}
output "map_value" {
value = lookup(local.map_example, "key1", "default_value")
}
Practical Tips for Using Terraform Expressions and Functions
To make the most out of Terraform expressions and functions, consider the following practical tips:
- Always validate your expressions and functions using
terraform validate
to catch syntax errors early. - Use local values to simplify your configurations and improve readability.
- Leverage conditionals and loops to minimize redundancy in your infrastructure code.
- Regularly consult the Terraform documentation and learn about new functions and updates.
Conclusion
Mastering Terraform expressions and function calls is crucial for optimizing infrastructure automation. By understanding the syntax, utilizing advanced features like conditionals and loops, and applying appropriate functions, you can create dynamic, efficient, and maintainable infrastructure as code.
If you haven’t started exploring Terraform expressions and functions already, now is the perfect time to dive in. Unlock the full potential of Terraform and take your infrastructure automation to the next level.
Happy coding!
Recent Posts
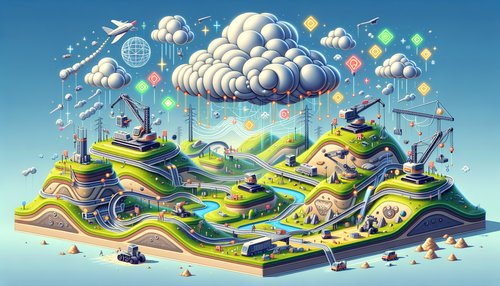
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
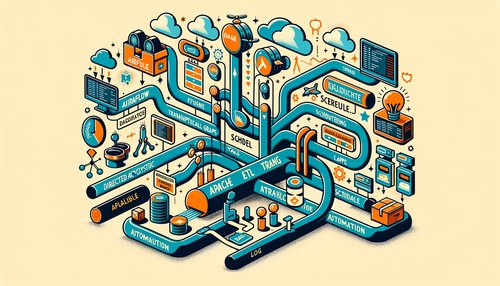
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow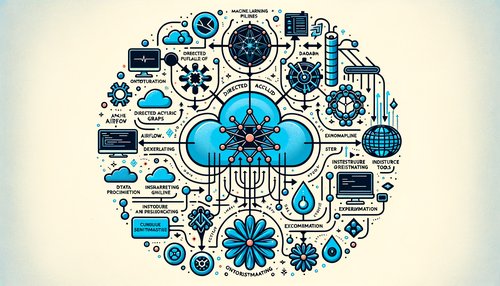
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow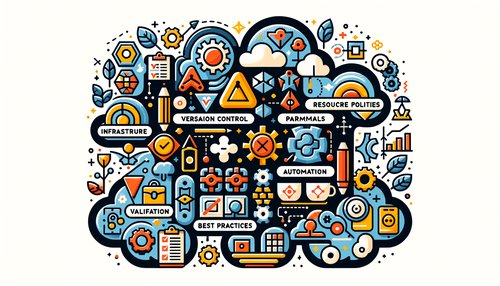
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
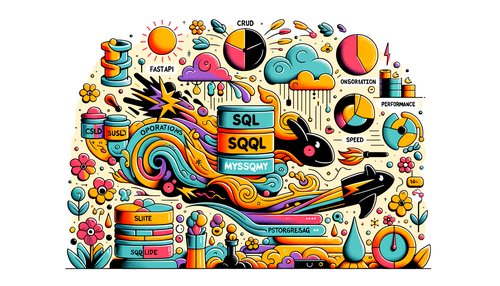