Unlocking Seamless Development with FastAPI: Mastering Dependencies in Path Operation Decorators - Your Ultimate Guide!
Welcome to the definitive guide on leveraging FastAPI to its full potential, focusing on one of its most powerful features: dependencies in path operation decorators. FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+, has taken the development world by storm, thanks to its simplicity, speed, and robustness. Among its arsenal of features, dependency injection stands out, allowing developers to create reusable components, share database sessions, and enforce security and authentication in a clean, efficient manner. This guide aims to demystify the process, providing you with the knowledge to enhance your development workflow significantly.
Understanding Dependency Injection in FastAPI
Before diving into the specifics, it's crucial to grasp what dependency injection is and how FastAPI implements this concept. At its core, dependency injection in FastAPI allows you to define how application components (such as database connections, user authentication systems, and external service clients) should be provided to your path operation functions. This is achieved through the use of "dependencies" - reusable pieces of logic that the FastAPI router can inject into your path operations, reducing code duplication and promoting cleaner, more maintainable code.
Creating Your First Dependency
Starting with dependencies is straightforward. Here's a simple example:
from fastapi import Depends, FastAPI
def common_parameters(q: str = None, skip: int = 0, limit: int = 100):
return {"q": q, "skip": skip, "limit": limit}
app = FastAPI()
@app.get("/items/")
async def read_items(commons: dict = Depends(common_parameters)):
return {"message": "Hello World", **commons}
This example illustrates a basic dependency (`common_parameters`) that extracts common query parameters. It's then injected into a path operation function (`read_items`) using the `Depends` class.
Advanced Dependency Techniques
FastAPI's dependency system doesn't stop at simple use cases. It supports more complex scenarios, such as sub-dependencies, dependencies with yield, and using classes as dependencies. These advanced techniques open up possibilities for even more powerful and flexible API designs.
Sub-dependencies
Dependencies can have their own dependencies, allowing for sophisticated composition and reuse. Here's how sub-dependencies work:
def sub_dependency():
return {"timestamp": datetime.utcnow()}
def main_dependency(sub_dep=Depends(sub_dependency)):
return {"sub": sub_dep}
@app.get("/sub/")
async def get_sub_dep(main_dep=Depends(main_dependency)):
return main_dep
This example demonstrates how a main dependency (`main_dependency`) can rely on a sub-dependency (`sub_dependency`), showcasing the power of dependency chaining.
Dependencies with Yield
FastAPI supports dependencies that perform some action before and after the request, using Python's `yield` syntax. This is particularly useful for resources that need to be opened and closed around a request, like database sessions:
from fastapi import Depends, FastAPI
from sqlalchemy.orm import Session
def get_db():
db = DBSession()
try:
yield db
finally:
db.close()
@app.get("/users/")
async def read_users(db: Session = Depends(get_db)):
users = db.query(User).all()
return users
This pattern ensures that the database session is properly closed after the request, regardless of whether an exception occurred.
Security and Authentication
FastAPI's dependency system is also your ally in implementing security and authentication mechanisms. By defining security dependencies, you can protect your API routes with various authentication schemes, including OAuth2, API keys, and more, all while keeping your code clean and DRY (Don't Repeat Yourself).
Implementing OAuth2 with Password (and Bearer)
FastAPI makes it easy to set up OAuth2 with password (and Bearer) authentication:
from fastapi.security import OAuth2PasswordBearer
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
@app.post("/token")
async def token():
return {"access_token": "your_access_token", "token_type": "bearer"}
@app.get("/users/me")
async def read_users_me(token: str = Depends(oauth2_scheme)):
return {"token": token}
In this example, `OAuth2PasswordBearer` is a dependency that ensures that the route receives a valid OAuth2 token.
Conclusion
FastAPI's dependency injection system is a game-changer, offering unparalleled flexibility and efficiency in developing APIs. By mastering dependencies in path operation decorators, you unlock the ability to write cleaner, more maintainable, and secure code. Whether you're managing database connections, implementing complex business logic, or securing your API, dependencies provide a robust solution. This guide has equipped you with the knowledge to start leveraging these powerful features in your FastAPI projects. The path to seamless development is now open; your next step is to explore and experiment with FastAPI's dependencies to create APIs that stand out in the modern web landscape.
Embrace the power of FastAPI and transform your development workflow today!
Recent Posts
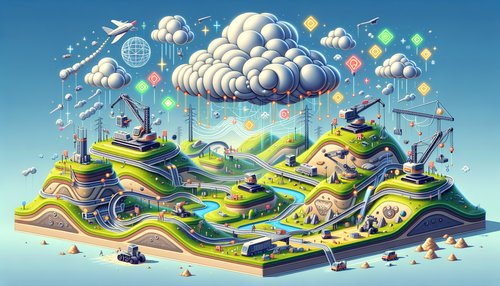
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
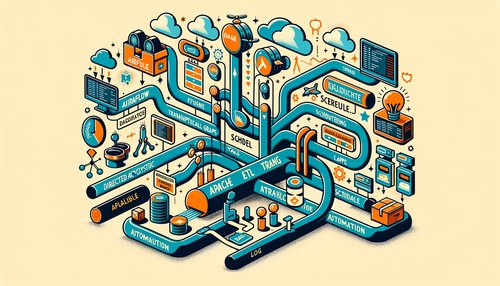
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow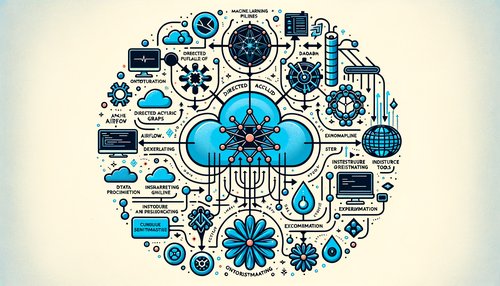
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow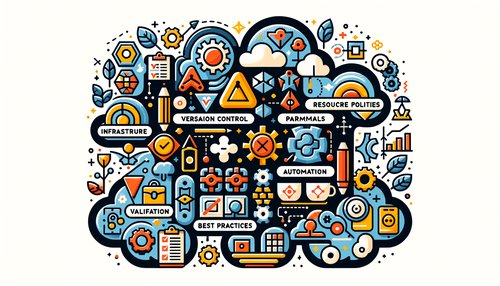
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
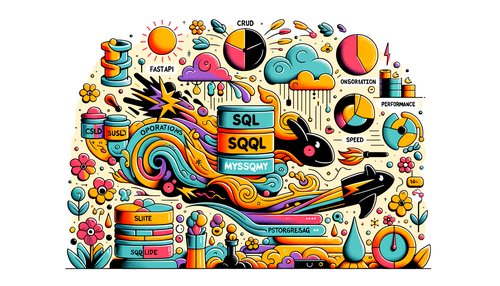