Unlocking Seamless Web Interactions: A Comprehensive Guide to Implementing CORS with FastAPI for Enhanced User Experience
In the rapidly evolving landscape of web development, creating seamless, interactive user experiences is paramount. One critical aspect that often becomes a stumbling block is dealing with Cross-Origin Resource Sharing (CORS) issues. This guide aims to demystify CORS and showcase how to effectively implement it within FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. By the end of this post, you'll have a solid understanding of CORS and how to leverage FastAPI features to resolve common issues, thereby enhancing your web applications' user experience.
Understanding CORS
Before diving into the implementation, let's unpack what CORS is and why it's crucial for web development. CORS is a security feature implemented by web browsers to prevent malicious websites from accessing resources and data from another domain without permission. While it's a critical security measure, it can often become an obstacle during development, especially when your frontend and backend are hosted on different domains.
Why FastAPI?
FastAPI is gaining traction for its speed, ease of use, and robustness in building APIs. It's designed to be easy to use, leveraging the latest Python features to provide a performance that rivals NodeJS and Go. Moreover, FastAPI simplifies dealing with CORS, making it an excellent choice for developers looking to streamline their development process and focus on creating fantastic user experiences.
Setting Up CORS in FastAPI
Implementing CORS in FastAPI is straightforward, thanks to its built-in middleware. Here's a step-by-step guide to setting it up:
- Install FastAPI and Uvicorn: If you haven't already, start by installing FastAPI and Uvicorn, an ASGI server, by running
pip install fastapi[all] uvicorn
. - Add CORS Middleware: Import
CORSMiddleware
fromfastapi.middleware.cors
and add it to your application. This involves creating a list of allowed origins (the domains you want to allow to access your API) and adding the middleware to your FastAPI app.
from fastapi import FastAPI
from fastapi.middleware.cors import CORSMiddleware
app = FastAPI()
origins = [
"http://www.example.com",
"https://www.example.com",
]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)
This snippet configures your FastAPI app to accept requests from http://www.example.com
and https://www.example.com
, allowing all methods and headers.
Advanced CORS Configurations
While the basic setup covers many use cases, sometimes you need more granular control over your CORS policy. FastAPI's CORSMiddleware
allows for advanced configurations, such as:
- Allowing Specific Methods and Headers: You can specify which HTTP methods and headers are allowed by replacing the
allow_methods=["*"]
andallow_headers=["*"]
with lists of permitted methods and headers. - Exposing Headers: Use the
expose_headers
argument to list which headers can be exposed to the browser. - Max Age: The
max_age
argument controls how long the results of a preflight request can be cached. This can reduce the number of preflight requests made by the browser, improving performance for repeated requests.
Testing and Debugging CORS
Once you've set up CORS in your FastAPI app, it's crucial to test and ensure everything works as expected. Tools like Postman or your browser's developer console can help you simulate requests from different origins and inspect the responses. Pay close attention to the response headers to ensure they include the correct CORS headers.
Conclusion
Implementing CORS with FastAPI can significantly enhance the user experience by ensuring seamless interactions between your frontend and backend, regardless of where they're hosted. By following this guide, you've learned the basics of CORS, how to set it up in FastAPI, and how to handle more advanced configurations. Remember, while CORS can seem daunting at first, FastAPI's straightforward approach simplifies the process, allowing you to focus on what truly matters: building incredible web applications.
With this knowledge in hand, you're well-equipped to tackle CORS-related challenges in your projects, ensuring your web applications are secure, reliable, and user-friendly. Happy coding!
Recent Posts
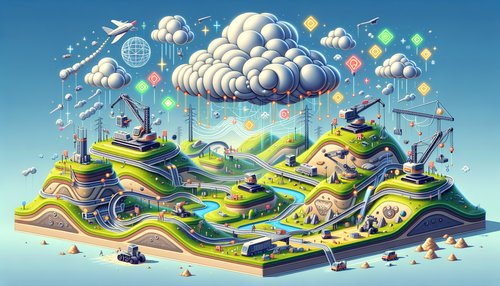
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
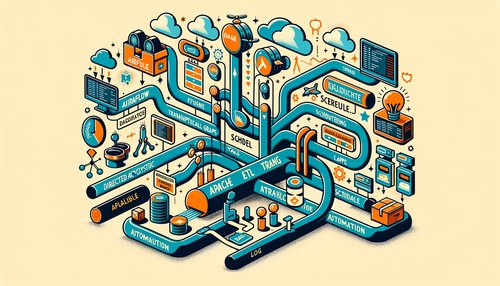
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow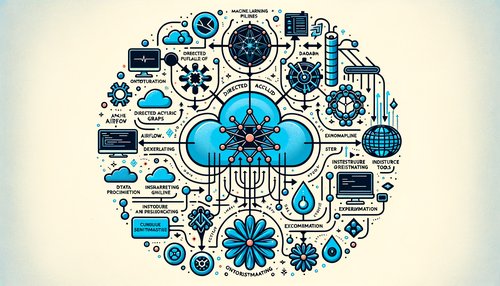
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow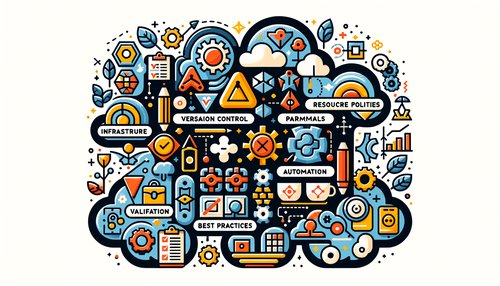
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
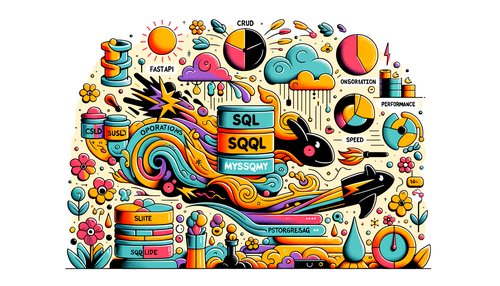