Unlocking Secure APIs: A Simplified Guide to Implementing OAuth2 with Password and Bearer in FastAPI
In today's digital age, securing APIs has become paramount for any application handling sensitive data. OAuth2 stands out as a robust protocol designed to offer secure and limited access to your resources without exposing user credentials. FastAPI, a modern, fast web framework for building APIs with Python, provides built-in support for OAuth2. This guide will walk you through the steps to implement OAuth2 authentication with Password and Bearer tokens in FastAPI, ensuring your API remains secure yet accessible.
Understanding OAuth2 and Its Importance
Before diving into the implementation, it's crucial to grasp what OAuth2 is and why it's essential. OAuth2 is an authorization framework that allows third-party services to exchange web resources on behalf of a user. It's the industry-standard protocol for authorization and is used worldwide to protect API endpoints. By implementing OAuth2, you ensure that your API's sensitive information remains secure from unauthorized access, providing peace of mind to both you and your users.
Setting Up Your FastAPI Project
First things first, you need to set up a FastAPI project. If you haven't done so already, you can start by installing FastAPI and Uvicorn, an ASGI server, to run your application. Use the following command to install both:
pip install fastapi uvicorn
Once installed, create a new Python file for your application, for example, main.py
, and import FastAPI:
from fastapi import FastAPI
app = FastAPI()
This code snippet initializes your FastAPI application, making it ready for further configurations and route definitions.
Implementing OAuth2 with Password Flow
OAuth2 supports various flows for different use cases. The Password flow, suitable for trusted applications, is one where users provide their credentials directly to the application. In FastAPI, you can implement this by utilizing the OAuth2PasswordBearer
and OAuth2PasswordRequestForm
classes.
First, define the token URL and the OAuth2PasswordBearer
instance:
from fastapi.security import OAuth2PasswordBearer
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
This code snippet specifies the URL where your application will receive the token requests.
Next, create an endpoint to generate tokens. This endpoint will receive the username and password, verify them, and return a JWT token if the authentication is successful:
from fastapi import Depends, FastAPI
from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm
from jose import JWTError, jwt
from datetime import datetime, timedelta
# Your secret key
SECRET_KEY = "YOUR_SECRET_KEY"
# Algorithm used for JWT encoding and decoding
ALGORITHM = "HS256"
# Expiration time of the token
ACCESS_TOKEN_EXPIRE_MINUTES = 30
app = FastAPI()
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
@app.post("/token")
async def generate_token(form_data: OAuth2PasswordRequestForm = Depends()):
# Here you'd verify username and password from form_data.username and form_data.password
# For this example, let's assume any username and password is valid
user_username = form_data.username
# If successful, create a JWT token
access_token_expires = timedelta(minutes=ACCESS_TOKEN_EXPIRE_MINUTES)
access_token = create_access_token(data={"sub": user_username}, expires_delta=access_token_expires)
return {"access_token": access_token, "token_type": "bearer"}
This endpoint uses the OAuth2PasswordRequestForm
to obtain user credentials and, if valid, returns a JWT token. The function create_access_token
is a utility function you would need to implement to generate JWT tokens.
Securing API Endpoints
With the authentication flow in place, you can now secure your API endpoints. To require a valid token for accessing an endpoint, you can depend on the oauth2_scheme
in your route functions:
from fastapi import Depends, HTTPException, status
@app.get("/users/me")
async def read_users_me(token: str = Depends(oauth2_scheme)):
# Your token verification logic here
return {"user": "example user"}
This endpoint extracts the token from the request and expects it to be validated within the function. If the token is valid, the endpoint will return the requested resource.
Conclusion
Implementing OAuth2 in FastAPI with Password and Bearer tokens is a straightforward process that significantly enhances the security of your APIs. By following the steps outlined in this guide, you can ensure that your API endpoints are protected against unauthorized access, providing a safe and reliable service to your users. Remember, security is an ongoing process, and it's crucial to stay updated on best practices and potential vulnerabilities within your application.
With this foundation, you're well on your way to developing secure applications with FastAPI. Happy coding!
Recent Posts
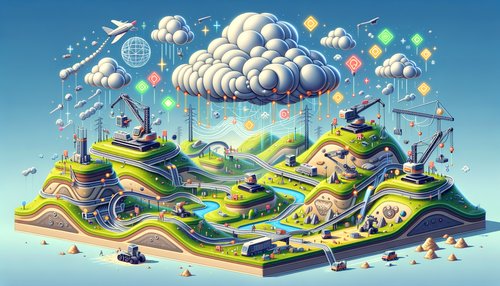
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
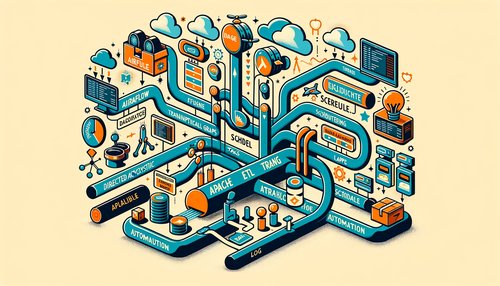
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow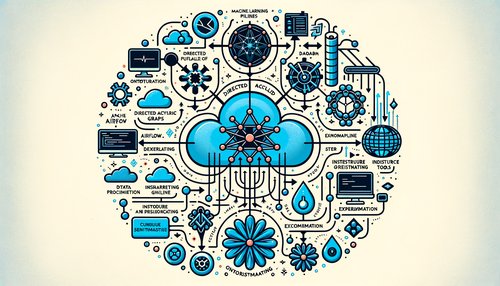
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow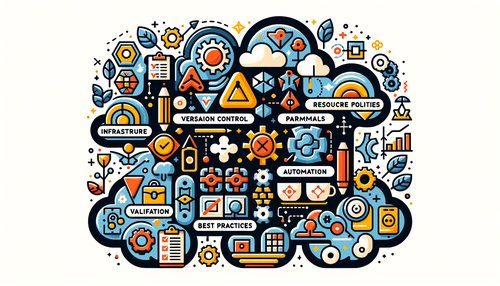
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
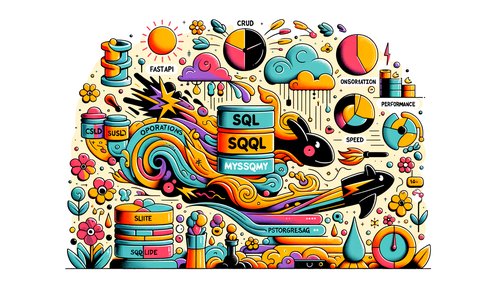