Unlocking Secure APIs: Mastering FastAPI with OAuth2, Password Hashing, and JWT Bearer Tokens - Your Ultimate User Guide
Welcome to the ultimate guide on securing your APIs using FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints. In today's digital age, securing your application's data is paramount, and leveraging FastAPI with OAuth2, password hashing, and JWT (JSON Web Tokens) is a robust strategy to achieve this. This comprehensive guide will walk you through the essentials of implementing these security measures, ensuring that your application is not only secure but also scalable and efficient. Let's dive into the world of secure APIs!
Understanding FastAPI
FastAPI is an innovative web framework for building APIs with Python that simplifies the process of creating robust and secure web applications. Its key features include automatic validation of requests, generation of OpenAPI documentation, and support for asynchronous request handling, making it an ideal choice for high-performance applications. Before we delve into the specifics of OAuth2 and JWT, it's crucial to understand the foundation FastAPI provides for building secure APIs.
OAuth2 and Authentication
OAuth2 is an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service. It works by delegating user authentication to the service that hosts the user account and authorizing third-party applications to access the user account. OAuth2 provides several "grant" types, but for most applications, the "Authorization Code" grant type is the most appropriate. Implementing OAuth2 in FastAPI is straightforward, thanks to its comprehensive documentation and community-contributed security utilities.
Practical Tip: Implementing OAuth2 in FastAPI
When implementing OAuth2 in FastAPI, use the fastapi.security.OAuth2PasswordBearer
class, which is designed to work with the "password" flow. This flow is particularly well-suited for building APIs that are consumed by trusted first-party clients, such as your own mobile or web app.
Password Hashing
Password hashing is a critical security measure that protects users' passwords by converting them into a hash, a fixed-size string of characters that is virtually impossible to reverse-engineer. FastAPI does not include a built-in mechanism for password hashing; however, integrating a library such as Passlib is straightforward and significantly enhances the security of your application.
Example: Using Passlib with FastAPI
Passlib is a password hashing library for Python that supports multiple hashing algorithms. A common and highly recommended algorithm is Bcrypt. Here's a quick example of how to use Passlib with FastAPI:
from passlib.context import CryptContext
pwd_context = CryptContext(schemes=["bcrypt"], deprecated="auto")
def hash_password(password: str):
return pwd_context.hash(password)
def verify_password(plain_password, hashed_password):
return pwd_context.verify(plain_password, hashed_password)
JWT Bearer Tokens
JSON Web Tokens (JWT) provide a compact and self-contained way to securely transmit information between parties as a JSON object. JWTs can be signed using a secret (with the HMAC algorithm) or a public/private key pair using RSA or ECDSA. In the context of FastAPI, JWTs are commonly used for authentication and authorization, allowing servers to verify the token's integrity and the user's identity without querying the database on each request.
Insight: Generating and Validating JWTs in FastAPI
FastAPI makes it easy to work with JWTs through the PyJWT library. Generating a token involves specifying a secret key and an expiration time. Validating a token requires extracting the user information and ensuring the token is valid and not expired. This process effectively reduces the load on your database and speeds up request processing.
Conclusion
In this guide, we've explored how to secure your APIs using FastAPI with OAuth2, password hashing, and JWT bearer tokens. By understanding the foundational principles of FastAPI and integrating these security measures, you can build robust, efficient, and secure web applications. Remember, security is not a one-time setup but an ongoing process. Continuously update and audit your security practices to protect against new vulnerabilities and threats.
As a final thought, consider contributing to the FastAPI community or sharing your insights and experiences with these security practices. Together, we can make the digital world a safer place. Happy coding!
Recent Posts
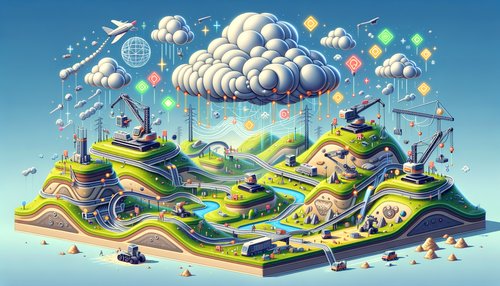
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
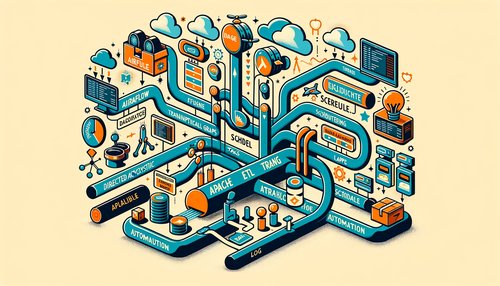
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow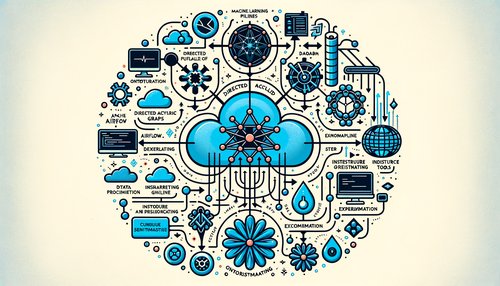
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow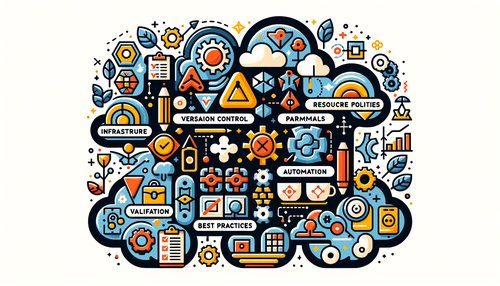
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
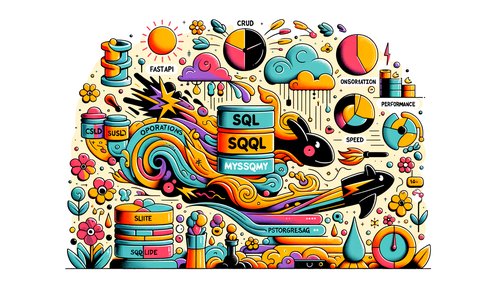