Unlocking Secure User Management in FastAPI: Your Ultimate Guide to Implementing GetCurrent User
Welcome to the definitive guide on mastering secure user management in FastAPI, with a special focus on implementing the GetCurrent user functionality. In this comprehensive blog post, we will navigate through the intricacies of setting up a robust authentication system that not only secures your application but also enhances user experience by efficiently managing user sessions. Whether you're building a new project from scratch or looking to improve an existing one, this guide is tailored to provide practical insights and actionable tips to elevate your FastAPI application.
Understanding User Authentication in FastAPI
Before diving into the GetCurrent user implementation, it's crucial to grasp the basics of user authentication in FastAPI. Authentication is the process of verifying the identity of a user or process. In web applications, this typically involves signing in with a username and password. FastAPI, being a modern and fast (high-performance) web framework for building APIs with Python 3.7+ based on standard Python type hints, provides several tools and mechanisms to implement secure authentication.
Why Focus on Secure User Management?
Secure user management is the cornerstone of any reliable application. It protects sensitive user information and ensures that unauthorized individuals cannot access critical functionalities. Moreover, it plays a significant role in compliance with data protection regulations and standards. By implementing GetCurrent user functionality, developers can streamline user experiences and provide personalized content, making applications more interactive and user-friendly.
Step-by-Step Guide to Implementing GetCurrent User in FastAPI
Implementing GetCurrent user functionality in FastAPI involves several key steps. This section will guide you through each phase, from setting up authentication dependencies to writing the actual code.
Setting Up Authentication Dependencies
The first step is to set up the necessary dependencies for authentication. FastAPI utilizes Pydantic and security utilities from Starlette (a lightweight ASGI framework/toolkit), which are essential for handling security schemes such as OAuth2:
pip install fastapi[all]
Creating User Models
Next, define your user models using Pydantic. These models will represent users within your application and will be crucial for handling authentication and authorization:
from pydantic import BaseModel
class User(BaseModel):
username: str
email: str
full_name: str = None
Implementing OAuth2 with Password (and Bearer)
FastAPI provides support for OAuth2 with Password (and Bearer), which is a simple way to implement access token control. This involves creating a security scheme and utilizing it in your endpoint functions:
from fastapi import Depends, FastAPI, HTTPException, status
from fastapi.security import OAuth2PasswordBearer
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
app = FastAPI()
async def get_current_user(token: str = Depends(oauth2_scheme)):
user = fake_decode_token(token)
if not user:
raise HTTPException(
status_code=status.HTTP_401_UNAUTHORIZED,
detail="Invalid authentication credentials",
headers={"WWW-Authenticate": "Bearer"},
)
return user
Validating Tokens
The get_current_user
function is where the magic happens. It's responsible for validating the access token and returning the current user. This function can be added as a dependency in any endpoint to ensure that the user is authenticated:
@app.get("/users/me")
async def read_users_me(current_user: User = Depends(get_current_user)):
return current_user
Best Practices for Secure User Management
While implementing GetCurrent user functionality is a significant step towards secure user management, there are several best practices you should follow to ensure maximum security:
- Use HTTPS: Always use HTTPS to encrypt data in transit between the client and server.
- Store Passwords Securely: Use hashing algorithms like Bcrypt to store passwords securely.
- Implement Rate Limiting: Protect your application from brute force attacks by limiting the number of login attempts.
- Regularly Update Dependencies: Keep all dependencies up to date to protect against known vulnerabilities.
Conclusion
In this blog post, we've explored the critical aspects of implementing secure user management in FastAPI, with a focus on the GetCurrent user functionality. By understanding the basics of user authentication, setting up the necessary dependencies, and following best practices, you can create a secure and user-friendly application. Remember, secure user management is not just about protecting data; it's about building trust with your users. So, take this guide as a starting point and continue to explore and implement advanced security features in your FastAPI applications.
As developers, our journey towards creating secure and efficient applications is ongoing. Let this guide be a stepping stone in your journey of mastering FastAPI and secure user management. Happy coding!
Recent Posts
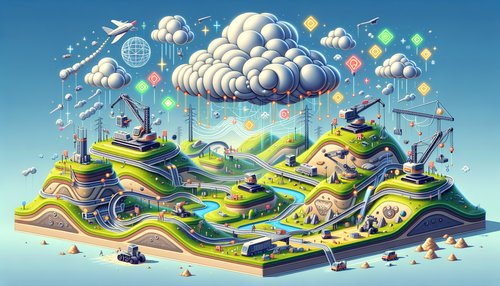
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
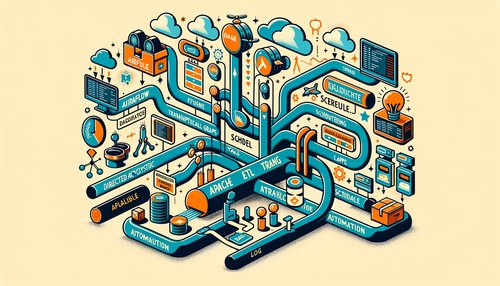
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow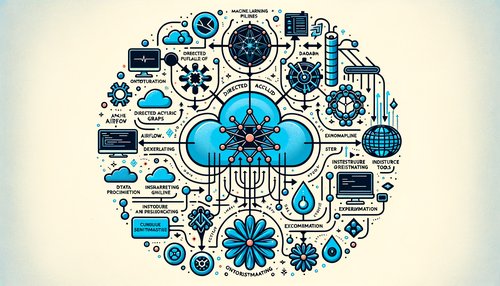
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow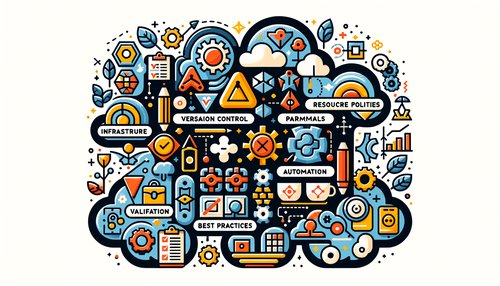
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
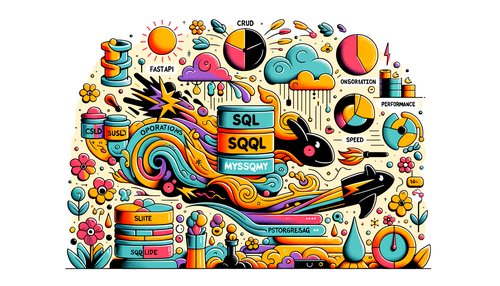