Unlocking Security in FastAPI: A Simple Guide to Implementing OAuth2 with Password and Bearer Authentication
Welcome to the world of FastAPI, a modern, fast (high-performance) web framework for building APIs with Python 3.6+ that's based on standard Python type hints. In this comprehensive guide, we will explore how to bolster the security of your FastAPI applications by implementing OAuth2 with Password and Bearer authentication schemes. Whether you're a seasoned developer or new to API security, this post will provide valuable insights and practical tips to help you navigate the complexities of OAuth2 and ensure your applications are secure and robust.
Understanding OAuth2 and Its Importance
Before diving into the implementation, let's first understand what OAuth2 is and why it's crucial for your FastAPI applications. OAuth2 is an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service. It works by delegating user authentication to the service that hosts the user account and authorizing third-party applications to access the user account. OAuth2 is widely used because it's secure, versatile, and supports several types of applications, including web and mobile apps.
Setting Up FastAPI with OAuth2
Implementing OAuth2 in FastAPI requires setting up a few key components:
- OAuth2PasswordBearer: A class that FastAPI provides to work with OAuth2 password flow. It's a token-based authentication scheme where the token is a bearer token.
- Security Schemes: Define how your application's API security is structured. FastAPI utilizes Pydantic models to declare request bodies, query parameters, and other elements, including security schemes.
Here's a simple example to set up OAuth2PasswordBearer in FastAPI:
from fastapi import FastAPI, Depends, HTTPException, status from fastapi.security import OAuth2PasswordBearer app = FastAPI() oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token") @app.post("/token") async def token(): return {"token": "your_secure_token"}
This snippet creates an OAuth2PasswordBearer instance that expects the client to send a token as a bearer token.
Creating a Password Flow with OAuth2
To implement a password flow with OAuth2 in FastAPI, follow these steps:
- Create user authentication logic that verifies username and password against your database or any other storage system.
- Generate a token if the authentication is successful. You can use libraries like
jwt
orpython-jose
to generate JWT tokens. - Return the token to the user.
Here's an example of generating a token:
from jose import JWTError, jwt from datetime import datetime, timedelta SECRET_KEY = "your_secret_key" ALGORITHM = "HS256" def create_access_token(data: dict): to_encode = data.copy() expire = datetime.utcnow() + timedelta(minutes=15) to_encode.update({"exp": expire}) encoded_jwt = jwt.encode(to_encode, SECRET_KEY, algorithm=ALGORITHM) return encoded_jwt
This function creates a JWT token that expires in 15 minutes.
Securing API Endpoints
With the OAuth2 setup complete and the ability to generate tokens, you can now secure your API endpoints. Use the Depends
dependency in your route functions to ensure that only authenticated users can access them.
@app.get("/users/me") async def read_users_me(token: str = Depends(oauth2_scheme)): user = get_current_user(token) return user
This endpoint requires a valid token to access the user's information.
Conclusion
Implementing OAuth2 with Password and Bearer authentication in FastAPI is a robust way to secure your applications. By following the steps outlined in this guide, you can ensure that your API endpoints are protected and only accessible to authenticated users. Remember, security is an ongoing process, and it's important to stay informed about best practices and updates in the FastAPI framework and OAuth2 specification.
Now that you have the knowledge to implement OAuth2 in your FastAPI applications, it's time to put it into practice. Secure your applications, protect your users, and build with confidence.
Recent Posts
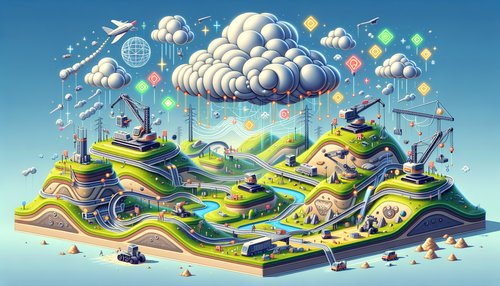
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
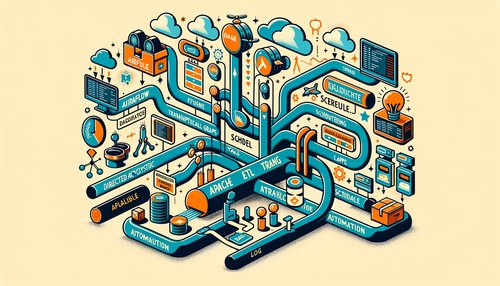
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow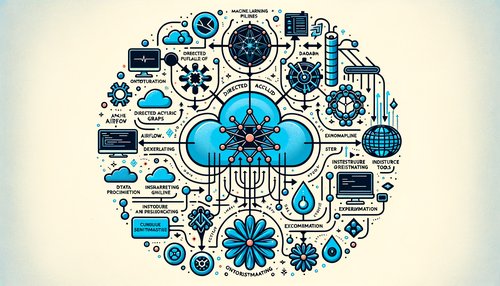
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow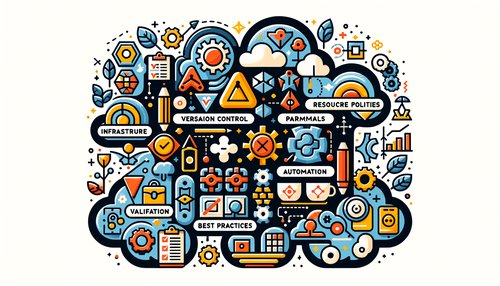
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
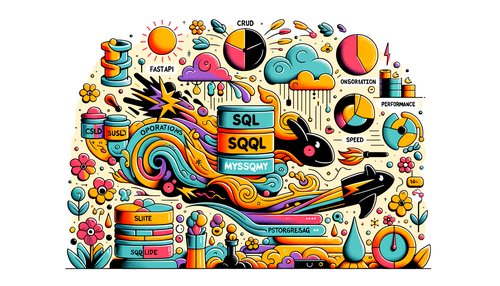