Unlocking the Full Potential of FastAPI: A Comprehensive User Guide to Metadata and Documentation URLs
FastAPI has quickly become one of the most appreciated web frameworks for building APIs with Python due to its stunning performance, ease of use, and developer-friendly features. In this guide, we will explore how you can leverage metadata and customize documentation URLs to make your API not just functional, but a pleasure to work with.
Introduction to FastAPI Metadata
FastAPI allows you to add metadata to your application, which can be extremely useful for generating automatic documentation, providing versioning, and including descriptive information about your API. This metadata can be added directly in your code, making it accessible within the development environment.
Adding Metadata
To include metadata in your FastAPI application, you can use the title
, description
, and version
parameters when creating an instance of the FastAPI class. Here is an example:
from fastapi import FastAPI
app = FastAPI(
title="My Awesome API",
description="This is an API that does awesome things.",
version="1.0.0"
)
This simple snippet adds a title, description, and version to your API, which will be reflected in the generated documentation.
Using Tags for Better Organization
Tags can be utilized to categorize your API routes, making them easier to navigate in the documentation. You can add tags by including them in the route decorators:
@app.get("/items/", tags=["items"])
def read_items():
return ["item1", "item2"]
Tags are displayed prominently in the auto-generated documentation, allowing developers to quickly find relevant endpoints.
Combining Tags with Metadata
By combining tags with metadata, you can create a highly structured and descriptive API documentation. Here's how:
app = FastAPI(
title="My Awesome API",
description="This API allows users to manage items.",
version="1.0.0",
openapi_tags=[
{
"name": "items",
"description": "Operations with items.",
},
]
)
Customizing Documentation URLs
FastAPI automatically generates interactive API documentation, but you can also customize the URLs where these documents are served. By default, the documentation is available at /docs
and /redoc
, but these can be changed to better suit your needs.
Changing the Documentation URLs
To change the documentation URLs, FastAPI provides the docs_url
and redoc_url
parameters:
app = FastAPI(
docs_url="/custom-docs",
redoc_url="/custom-redoc",
)
With this setup, you can access the interactive Swagger UI at /custom-docs
and the ReDoc documentation at /custom-redoc
.
Disabling Documentation
If for some reason you need to disable the automatic documentation generation, you can set the URLs to None
:
app = FastAPI(
docs_url=None,
redoc_url=None,
)
This will prevent the documentation routes from being created, which might be useful in production environments where you want to minimize exposed information.
Practical Tips for Using Metadata and Documentation URLs
- Keep your metadata up-to-date as your API evolves to ensure that developers always have accurate information.
- Use meaningful tags to organize your endpoints logically.
- Consider custom documentation URLs that align with your organizational standards.
- Regularly review your auto-generated documentation to catch any inconsistencies or errors.
Conclusion
By effectively utilizing metadata and customizing documentation URLs, you can significantly enhance the usability and maintainability of your FastAPI application. These features not only provide clear and comprehensive documentation for developers but also pave the way for a more structured and professional API. Start implementing these tips today and unlock the full potential of FastAPI!
Recent Posts
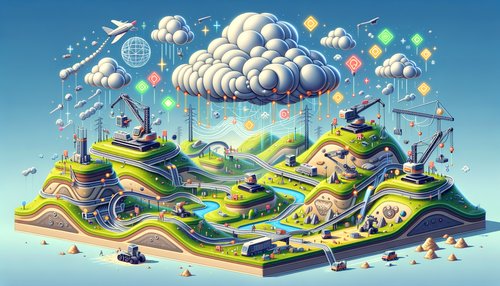
Unlocking the Power of Terraform: Mastering Conditional Expressions for Smarter Infrastructure Automation
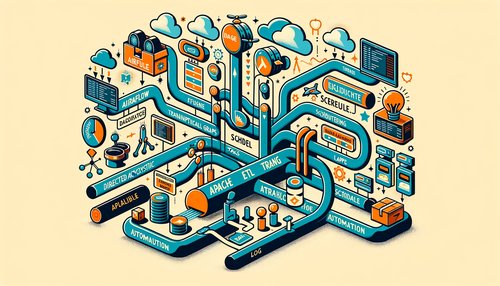
Unveiling the Future: Navigating the Public Interface of Apache Airflow for Streamlined Workflow Management
Apache Airflow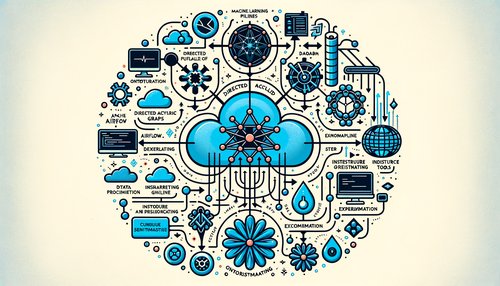
Mastering Workflow Automation: Unconventional Apache Airflow How-To Guides for the Modern Data Enthusiast
Apache Airflow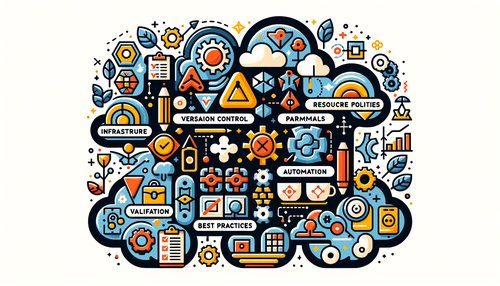
Mastering the Cloud: Unveiling AWS CloudFormation Best Practices for Seamless Infrastructure Management
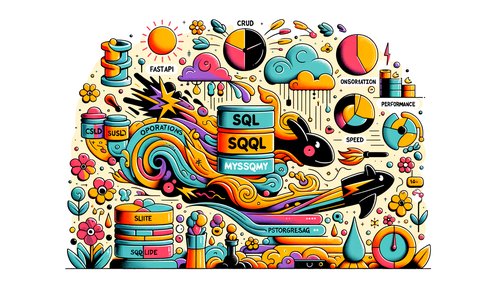